Merge branch 'feature/Chatbot_megabox' into 'master'
Feature/chatbot megabox See merge request !35
Showing
8 changed files
with
371 additions
and
21 deletions
Chatbot/Megabox.js
0 → 100644
1 | +const chatbot = require("./app.js"); | ||
2 | +const request = require('request'); | ||
3 | +const cheerio = require('cheerio'); | ||
4 | +const puppeteer = require('puppeteer'); | ||
5 | +require('chromedriver'); | ||
6 | +const {Builder,until} = require('selenium-webdriver'); //모듈 불러오기 | ||
7 | +var webdriver = require('selenium-webdriver'); | ||
8 | +var By = webdriver.By; | ||
9 | +const chrome = require('selenium-webdriver/chrome');//크롬 사용시 | ||
10 | +const async = require('async') | ||
11 | +let express = require('express'); | ||
12 | +let app = express(); | ||
13 | +let bodyParser = require('body-parser'); | ||
14 | +const { timeout } = require('async'); | ||
15 | +app.use(bodyParser.urlencoded({ extended: false })); | ||
16 | +app.use(bodyParser.json()); | ||
17 | +const booking_url = "https://megabox.co.kr/booking?"; | ||
18 | +exports.booking_url = booking_url; | ||
19 | +const rate_url = "https://www.megabox.co.kr/movie"; | ||
20 | +let r =0; | ||
21 | +let movie_data = []; | ||
22 | +exports.movie_data = movie_data; | ||
23 | +let location_data = []; | ||
24 | +exports.location_data = location_data; | ||
25 | +let index = 0; | ||
26 | +exports.init = ()=>{async.waterfall([//for 동기적 처리 | ||
27 | + async () => { | ||
28 | + const driver = new webdriver.Builder().forBrowser('chrome').setChromeOptions(new chrome.Options().headless()).build();// | ||
29 | + driver.get(booking_url); | ||
30 | + driver.switchTo().frame(0)//frameBokdMBooking 프레임 가져옴 | ||
31 | + let seoul = await driver.wait(until.elementsLocated(By.css('#mCSB_4_container>ul>li>#btn'))); | ||
32 | + let Gyeonggi = await driver.wait(until.elementsLocated(By.css('#mCSB_5_container>ul>li>#btn'))); | ||
33 | + const Incheon = await driver.wait(until.elementsLocated(By.css('#mCSB_6_container>ul>li>#btn'))); | ||
34 | + const DCS = await driver.wait(until.elementsLocated(By.css('#mCSB_7_container>ul>li>#btn')));//Daejeon Chungcheong Sejong | ||
35 | + const BDG = await driver.wait(until.elementsLocated(By.css('#mCSB_8_container>ul>li>#btn')));//Busan Daegu Gyeongsang | ||
36 | + const GJ= await driver.wait(until.elementsLocated(By.css('#mCSB_9_container>ul>li>#btn')));//gwangju_jeonla | ||
37 | + const Gangwon = await driver.wait(until.elementsLocated(By.css('#mCSB_10_container>ul>li>#btn'))); | ||
38 | + const location_list = [seoul, Gyeonggi, Incheon, DCS, BDG, GJ, Gangwon]// | ||
39 | + for(let i = 0; i < location_list.length; i++){ | ||
40 | + for (item of location_list[i]) { | ||
41 | + location_data[index++] = { | ||
42 | + 'LocationName':await item.getAttribute("brch-nm"), | ||
43 | + 'LocationNum' : await item.getAttribute("brch-no") | ||
44 | + } | ||
45 | + // let location_name = await item.getAttribute("brch-nm"); | ||
46 | + // let location_num = await item.getAttribute("brch-no"); | ||
47 | + // let obj = {}; | ||
48 | + // obj[location_name]= location_num | ||
49 | + // location_data[index++] = obj; | ||
50 | + } | ||
51 | + } | ||
52 | + let movie_list = await driver.wait(until.elementsLocated(By.css('#mCSB_1_container>ul>li>.btn'))); | ||
53 | + r = 0; | ||
54 | + for (item of movie_list) { | ||
55 | + //Using getAttribute to get the data | ||
56 | + movie_data[r++] = { | ||
57 | + 'rank' : r, | ||
58 | + 'title' : await item.getAttribute("movie-nm"), | ||
59 | + 'movie_num':await item.getAttribute("movie-no"), | ||
60 | + } | ||
61 | + } | ||
62 | + | ||
63 | + driver.close(); | ||
64 | + | ||
65 | + }, | ||
66 | + async () => { | ||
67 | + r = 0; | ||
68 | + const browser = await puppeteer.launch({ | ||
69 | + headless: true | ||
70 | + }); | ||
71 | + const page = await browser.newPage(); | ||
72 | + await page.goto(rate_url); | ||
73 | + const content = await page.content(); | ||
74 | + | ||
75 | + const $ = cheerio.load(content); | ||
76 | + const $rate_lists = $("ol.list>li"); | ||
77 | + $rate_lists.each((index, list) => { | ||
78 | + const name = $(list).find('div.tit-area > p.tit').attr('title'); | ||
79 | + const rate = $(list).find('div.rate-date > span.rate').text(); | ||
80 | + | ||
81 | + if(movie_data[r].title === name){ | ||
82 | + movie_data[r++]['rate'] = rate; | ||
83 | + } | ||
84 | + }); | ||
85 | + for(i of movie_data){ | ||
86 | + if(Object.keys(i).length==3){ | ||
87 | + movie_data[r++]['rate'] = '예매율 0%'; | ||
88 | + } | ||
89 | + } | ||
90 | + | ||
91 | + browser.close(); | ||
92 | + console.log("Comepleted!"); | ||
93 | + }, | ||
94 | + | ||
95 | +])} | ||
96 | + | ||
97 | +let appdriver; | ||
98 | +exports.using_PlayingMovieURL = async(PlayingMovieURL) => { | ||
99 | + appdriver = new webdriver.Builder().forBrowser('chrome').setChromeOptions(new chrome.Options().headless()).build(); | ||
100 | + appdriver.get(PlayingMovieURL); | ||
101 | + //appdriver.switchTo().frame(0) | ||
102 | + //frameBokdMBooking 프레임 가져옴 | ||
103 | +} | ||
104 | +exports.geting_PlayingMovie= async() => { | ||
105 | + let movie_list = await appdriver.wait(until.elementsLocated(By.css('#mCSB_1_container>ul>li>.btn'))); | ||
106 | + let n = 0; | ||
107 | + console.log(movie_list); | ||
108 | + for (item of movie_list) { | ||
109 | + movie_data[n++]['running'] = await item.getAttribute('form-at'); | ||
110 | + } | ||
111 | + console.log("Completed get Running"); | ||
112 | +} | ||
113 | + | ||
114 | +app.listen(5000); | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
Chatbot/app.js
0 → 100644
This diff is collapsed. Click to expand it.
Chatbot/package-lock.json
0 → 100644
This diff could not be displayed because it is too large.
Chatbot/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "megabox", | ||
3 | + "version": "1.0.0", | ||
4 | + "description": "", | ||
5 | + "main": "app.js", | ||
6 | + "scripts": { | ||
7 | + "test": "echo \"Error: no test specified\" && exit 1" | ||
8 | + }, | ||
9 | + "keywords": [], | ||
10 | + "author": "", | ||
11 | + "license": "ISC", | ||
12 | + "dependencies": { | ||
13 | + "async": "^3.2.3", | ||
14 | + "body-parser": "^1.20.0", | ||
15 | + "cheerio": "^1.0.0-rc.11", | ||
16 | + "chromedriver": "^101.0.0", | ||
17 | + "express": "^4.18.1", | ||
18 | + "express-async-handler": "^1.2.0", | ||
19 | + "moment": "^2.29.3", | ||
20 | + "puppeteer": "^14.1.1", | ||
21 | + "request": "^2.88.2", | ||
22 | + "selenium-webdriver": "^4.1.2" | ||
23 | + } | ||
24 | +} |
ChatbotTest_Megabox/Megabox.js
0 → 100644
1 | +const chatbot = require("./app.js"); | ||
2 | +const request = require('request'); | ||
3 | +const cheerio = require('cheerio'); | ||
4 | +const puppeteer = require('puppeteer'); | ||
5 | +require('chromedriver'); | ||
6 | +const {Builder,until} = require('selenium-webdriver'); //모듈 불러오기 | ||
7 | +var webdriver = require('selenium-webdriver'); | ||
8 | +var By = webdriver.By; | ||
9 | +const chrome = require('selenium-webdriver/chrome');//크롬 사용시 | ||
10 | +const async = require('async') | ||
11 | +let express = require('express'); | ||
12 | +let app = express(); | ||
13 | +let bodyParser = require('body-parser'); | ||
14 | +const { timeout } = require('async'); | ||
15 | +app.use(bodyParser.urlencoded({ extended: false })); | ||
16 | +app.use(bodyParser.json()); | ||
17 | +const booking_url = "https://megabox.co.kr/booking?"; | ||
18 | +exports.booking_url = booking_url; | ||
19 | +const rate_url = "https://www.megabox.co.kr/movie"; | ||
20 | +let r =0; | ||
21 | +let movie_data = []; | ||
22 | +exports.movie_data = movie_data; | ||
23 | +let location_data = []; | ||
24 | +exports.location_data = location_data; | ||
25 | +let index = 0; | ||
26 | +exports.init = ()=>{async.waterfall([//for 동기적 처리 | ||
27 | + async () => { | ||
28 | + const driver = new webdriver.Builder().forBrowser('chrome').setChromeOptions(new chrome.Options().headless()).build();// | ||
29 | + driver.get(booking_url); | ||
30 | + driver.switchTo().frame(0)//frameBokdMBooking 프레임 가져옴 | ||
31 | + let seoul = await driver.wait(until.elementsLocated(By.css('#mCSB_4_container>ul>li>#btn'))); | ||
32 | + let Gyeonggi = await driver.wait(until.elementsLocated(By.css('#mCSB_5_container>ul>li>#btn'))); | ||
33 | + const Incheon = await driver.wait(until.elementsLocated(By.css('#mCSB_6_container>ul>li>#btn'))); | ||
34 | + const DCS = await driver.wait(until.elementsLocated(By.css('#mCSB_7_container>ul>li>#btn')));//Daejeon Chungcheong Sejong | ||
35 | + const BDG = await driver.wait(until.elementsLocated(By.css('#mCSB_8_container>ul>li>#btn')));//Busan Daegu Gyeongsang | ||
36 | + const GJ= await driver.wait(until.elementsLocated(By.css('#mCSB_9_container>ul>li>#btn')));//gwangju_jeonla | ||
37 | + const Gangwon = await driver.wait(until.elementsLocated(By.css('#mCSB_10_container>ul>li>#btn'))); | ||
38 | + const location_list = [seoul, Gyeonggi, Incheon, DCS, BDG, GJ, Gangwon]// | ||
39 | + for(let i = 0; i < location_list.length; i++){ | ||
40 | + for (item of location_list[i]) { | ||
41 | + location_data[index++] = { | ||
42 | + 'LocationName':await item.getAttribute("brch-nm"), | ||
43 | + 'LocationNum' : await item.getAttribute("brch-no") | ||
44 | + } | ||
45 | + // let location_name = await item.getAttribute("brch-nm"); | ||
46 | + // let location_num = await item.getAttribute("brch-no"); | ||
47 | + // let obj = {}; | ||
48 | + // obj[location_name]= location_num | ||
49 | + // location_data[index++] = obj; | ||
50 | + } | ||
51 | + } | ||
52 | + let movie_list = await driver.wait(until.elementsLocated(By.css('#mCSB_1_container>ul>li>.btn'))); | ||
53 | + r = 0; | ||
54 | + for (item of movie_list) { | ||
55 | + //Using getAttribute to get the data | ||
56 | + movie_data[r++] = { | ||
57 | + 'rank' : r, | ||
58 | + 'title' : await item.getAttribute("movie-nm"), | ||
59 | + 'movie_num':await item.getAttribute("movie-no"), | ||
60 | + } | ||
61 | + } | ||
62 | + | ||
63 | + driver.close(); | ||
64 | + | ||
65 | + }, | ||
66 | + async () => { | ||
67 | + r = 0; | ||
68 | + const browser = await puppeteer.launch({ | ||
69 | + headless: true | ||
70 | + }); | ||
71 | + const page = await browser.newPage(); | ||
72 | + await page.goto(rate_url); | ||
73 | + const content = await page.content(); | ||
74 | + | ||
75 | + const $ = cheerio.load(content); | ||
76 | + const $rate_lists = $("ol.list>li"); | ||
77 | + $rate_lists.each((index, list) => { | ||
78 | + const name = $(list).find('div.tit-area > p.tit').attr('title'); | ||
79 | + const rate = $(list).find('div.rate-date > span.rate').text(); | ||
80 | + | ||
81 | + if(movie_data[r].title === name){ | ||
82 | + movie_data[r++]['rate'] = rate; | ||
83 | + } | ||
84 | + }); | ||
85 | + for(i of movie_data){ | ||
86 | + if(Object.keys(i).length==3){ | ||
87 | + movie_data[r++]['rate'] = '예매율 0%'; | ||
88 | + } | ||
89 | + } | ||
90 | + | ||
91 | + browser.close(); | ||
92 | + console.log("Comepleted!"); | ||
93 | + }, | ||
94 | + | ||
95 | +])} | ||
96 | +const appdriver = new webdriver.Builder().forBrowser('chrome').setChromeOptions(new chrome.Options().headless()).build(); | ||
97 | +exports.using_PlayingMovieURL = async(PlayingMovieURL) => { | ||
98 | + appdriver.get(PlayingMovieURL); | ||
99 | + //appdriver.switchTo().frame(0) | ||
100 | + //frameBokdMBooking 프레임 가져옴 | ||
101 | +} | ||
102 | +exports.geting_PlayingMovie= async() => { | ||
103 | + let movie_list = await appdriver.wait(until.elementsLocated(By.css('#mCSB_1_container>ul>li>.btn'))); | ||
104 | + let n = 0; | ||
105 | + for (item of movie_list) { | ||
106 | + movie_data[n++]['running'] = await item.getAttribute('form-at'); | ||
107 | + } | ||
108 | + console.log("Completed get Running"); | ||
109 | +} | ||
110 | +// let userData = { | ||
111 | +// 'Date': '', | ||
112 | +// 'location':'' | ||
113 | +// }; | ||
114 | +// // const _sleep = (delay) => new Promise((resolve) => setTimeout(resolve, delay)); | ||
115 | +// app.get('/Megabox', (req, res) => { | ||
116 | +// res.send(movie_data); | ||
117 | +// }) | ||
118 | +// app.post('/Megabox', (req, res) => {//사용자에게 Date와 location(영화관 장소) 받아옴 | ||
119 | +// let PlayingMovieURL; | ||
120 | +// userData['Date'] = req.body.Date; | ||
121 | +// for(i of location_data){ | ||
122 | +// if(i['LocationName'] == req.body.location){ | ||
123 | +// userData['location']=i['LocationNum']; | ||
124 | +// break; | ||
125 | +// } | ||
126 | +// } | ||
127 | +// PlayingMovieURL = booking_url + '?brchNo1='+userData['location']+'&playDe='+userData['Date'];//사용자 정보 바탕으로 해당 일자 영화관 영화 상영 여부 확인 | ||
128 | + | ||
129 | +// appdriver.get(PlayingMovieURL); | ||
130 | +// appdriver.switchTo().frame(0)//frameBokdMBooking 프레임 가져옴 | ||
131 | +// res.send(movie_data); | ||
132 | +// }) | ||
133 | +// app.post('/Megabox', (req, res) => {//사용자에게 Date와 location(영화관 장소) 받아옴 | ||
134 | +// userData['Date'] = req.body.Date; | ||
135 | +// for(i of location_data){ | ||
136 | +// if(i['LocationName'] == req.body.location){ | ||
137 | +// userData['location']=i['LocationNum']; | ||
138 | +// break; | ||
139 | +// } | ||
140 | +// } | ||
141 | +// let PlayingMovieURL = booking_url + '?brchNo1='+userData['location']+'&playDe='+userData['Date'];//사용자 정보 바탕으로 해당 일자 영화관 영화 상영 여부 확인 | ||
142 | + | ||
143 | +// appdriver.get(PlayingMovieURL); | ||
144 | +// appdriver.switchTo().frame(0)//frameBokdMBooking 프레임 가져옴 | ||
145 | +// res.send(movie_data); | ||
146 | +// }) | ||
147 | +app.get('/Megabox/GetPlayingMovie', async(req, res, next) => {//영화 상영 여부 객체에 넣음 | ||
148 | + | ||
149 | + // let movie_list = await appdriver.wait(until.elementsLocated(By.css('#mCSB_1_container>ul>li>.btn'))); | ||
150 | + // let n = 0; | ||
151 | + // for (item of movie_list) { | ||
152 | + // movie_data[n++]['running'] = await item.getAttribute('form-at') | ||
153 | + // } | ||
154 | + using_PlayingMovieURL('https://megabox.co.kr/booking?brchNo1=4451&playDe=20220606'); | ||
155 | + geting_PlayingMovie(); | ||
156 | + res.send(PlayingMovieList); | ||
157 | +}) | ||
158 | +app.listen(5000); | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
ChatbotTest_Megabox/app.js
0 → 100644
This diff is collapsed. Click to expand it.
ChatbotTest_Megabox/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "megabox", | ||
3 | + "version": "1.0.0", | ||
4 | + "description": "", | ||
5 | + "main": "app.js", | ||
6 | + "scripts": { | ||
7 | + "test": "echo \"Error: no test specified\" && exit 1" | ||
8 | + }, | ||
9 | + "keywords": [], | ||
10 | + "author": "", | ||
11 | + "license": "ISC", | ||
12 | + "dependencies": { | ||
13 | + "async": "^3.2.3", | ||
14 | + "body-parser": "^1.20.0", | ||
15 | + "cheerio": "^1.0.0-rc.11", | ||
16 | + "chromedriver": "^101.0.0", | ||
17 | + "express": "^4.18.1", | ||
18 | + "puppeteer": "^14.1.1", | ||
19 | + "express-async-handler": "^1.2.0", | ||
20 | + "selenium-webdriver": "^4.1.2" | ||
21 | + } | ||
22 | +} | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
1 | +[](https://nodejs.org/ko/) [](https://expressjs.com/ko/) | ||
2 | +[](https://github.com/puppeteer/puppeteer) [](https://www.selenium.dev/documentation/webdriver/) [](https://www.npmjs.com/package/express-async-handler) [](https://cheerio.js.org/) | ||
1 | # 3대 멀티플렉스 통합 예매 챗봇 | 3 | # 3대 멀티플렉스 통합 예매 챗봇 |
2 | 4 | ||
3 | 5 | ||
... | @@ -20,40 +22,70 @@ CGV, 롯데시네마, MEGABOX 영화관의 정보를 통합 제공 및 예매를 | ... | @@ -20,40 +22,70 @@ CGV, 롯데시네마, MEGABOX 영화관의 정보를 통합 제공 및 예매를 |
20 | + Line Messaging API | 22 | + Line Messaging API |
21 | + Kakao Search-by-Keyword API | 23 | + Kakao Search-by-Keyword API |
22 | 24 | ||
23 | -## Getting Started | ||
24 | 25 | ||
25 | -### Prerequisites | 26 | +## Getting Started |
26 | 27 | ||
27 | ### Installation | 28 | ### Installation |
28 | 29 | ||
30 | +1. Kakao REST API관련 KEY를 발급받습니다. | ||
31 | + [Kakao Search API](https://developers.kakao.com/) | ||
32 | +2. 해당 Repository를 Clone합니다. | ||
33 | + `git clone http://khuhub.khu.ac.kr/{YourID}/Multiplex_Ticketing_Platform.git` | ||
34 | +3. 코드를 실행하는데 필요한 npm 요소들을 Install합니다. | ||
35 | + `npm install` | ||
36 | +4. Line Messaging API - Webhook 설정에서 본인의 domain을 입력합니다. | ||
37 | +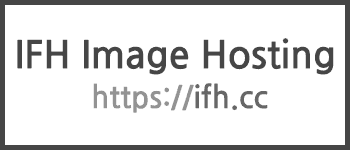 | ||
38 | +5. 코드에 본인이 발급받은 API KEY, Domain을 입력합니다. | ||
39 | + `const USER_ID = '{YOUR OWN LINE MESSAGING API USER_ID}';` | ||
40 | + `const TOKEN = '{YOUR OWN LINE MESSAGING API TOKEN}';` | ||
41 | + `const domain = '{YOUR OWN DOMAIN}';` | ||
42 | + `const KAKAO_KEY = '{YOUR OWN KAKAO REST API KEY}';` | ||
43 | +6. QR 코드를 휴대폰의 카메라로 스캔하거나 <__@583zdtpz__>을 친구 찾기에 입력하여 "영화관통합예매챗봇"을 추가합니다. | ||
44 | +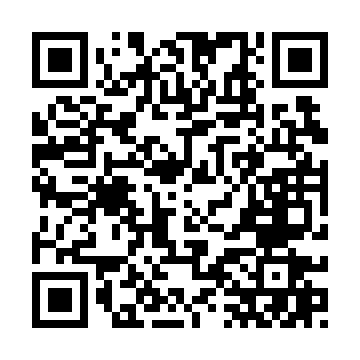 | ||
29 | 45 | ||
30 | -# Contributing | ||
31 | 46 | ||
32 | -1. 해당 Repository를 Fork합니다. | 47 | +## Usage |
33 | -`git fork http://khuhub.khu.ac.kr/2021105632/Multiplex_Ticketing_Platform.git` | ||
34 | -2. Fork한 Repository를 Clone합니다. | ||
35 | -`git clone http://khuhub.khu.ac.kr/{YourID}/Multiplex_Ticketing_Platform.git` | ||
36 | -3. 당신이 개발하고자 하는 기능의 Branch를 추가합니다. | ||
37 | -`git checkout -b feature/{YourBranchName}` | ||
38 | -4. 기능을 구현합니다. | ||
39 | -5. 당신이 추가한 기능 또는 수정 사항을 Commit합니다. | ||
40 | -`git commit -m 'Add feature {FeatureName}'` | ||
41 | -6. 당신의 Branch를 Push합니다. | ||
42 | -`git push origin feature/{YourBranchName}` | ||
43 | -7. Pull Request를 엽니다. | ||
44 | 48 | ||
49 | +챗봇을 추가하게 되면 자동으로 다음과 같은 메세지가 전송됩니다. | ||
45 | 50 | ||
46 | -## License | 51 | +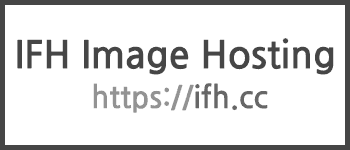 |
47 | 52 | ||
48 | -Apache License를 사용합니다. LICENSE.txt를 통해 자세한 정보를 확인해주세요. | 53 | +영화 예매 링크를 받는데 까지는 총 4가지의 단계를 거치게 됩니다! |
49 | 54 | ||
55 | +1. 브랜드 선택 | ||
56 | +2. 영화관 선택 | ||
57 | + 2-1.영화관 세부 선택 | ||
58 | +3. 날짜 선택 | ||
59 | +4. 상영 중인 영화 목록에서 원하는 영화 선택 | ||
50 | 60 | ||
51 | -## Contact | 61 | +위와 같은 단계로 입력이 모두 완료되면 선택하신 영화 예매 링크 및 영화관 위치 링크를 챗봇을 통해 바로 전달받으실 수 있습니다!! |
62 | + | ||
63 | + | ||
64 | +## Contributing | ||
52 | 65 | ||
53 | -> 임승현 - kevinlsh17@khu.ac.kr | 66 | +1. 해당 Repository를 Fork합니다. |
67 | +`git fork http://khuhub.khu.ac.kr/2021105632/Multiplex_Ticketing_Platform.git` | ||
68 | +2. Fork한 Repository를 Clone합니다. | ||
69 | +`git clone http://khuhub.khu.ac.kr/{YourID}/Multiplex_Ticketing_Platform.git` | ||
70 | +3. 당신이 개발하고자 하는 기능의 Branch를 추가합니다. | ||
71 | +`git checkout -b feature/{YourBranchName}` | ||
72 | +4. 기능을 구현합니다. | ||
73 | +5. 당신이 추가한 기능 또는 수정 사항을 Commit합니다. | ||
74 | +`git commit -m 'Add feature {FeatureName}'` | ||
75 | +6. 당신의 Branch를 Push합니다. | ||
76 | +`git push origin feature/{YourBranchName}` | ||
77 | +7. Pull Request를 엽니다. | ||
54 | 78 | ||
55 | -> 이혜인 - hil0409@khu.ac.kr | ||
56 | 79 | ||
57 | -> 신승민 - s091506@khu.ac.kr | 80 | +## License |
81 | + | ||
82 | +Apache License를 사용합니다. LICENSE.txt를 통해 자세한 정보를 확인해주세요. | ||
83 | + | ||
84 | + | ||
85 | +## Contact | ||
58 | 86 | ||
87 | +> 임승현 - kevinlsh17@khu.ac.kr | ||
88 | +> 이혜인 - hil0409@khu.ac.kr | ||
89 | +> 신승민 - s091506@khu.ac.kr | ||
90 | +> | ||
59 | > Project Link: [http://khuhub.khu.ac.kr/2021105632/Multiplex_Ticketing_Platform.git](http://khuhub.khu.ac.kr/2021105632/Multiplex_Ticketing_Platform.git) | 91 | > Project Link: [http://khuhub.khu.ac.kr/2021105632/Multiplex_Ticketing_Platform.git](http://khuhub.khu.ac.kr/2021105632/Multiplex_Ticketing_Platform.git) |
... | \ No newline at end of file | ... | \ No newline at end of file | ... | ... |
-
Please register or login to post a comment