Showing
334 changed files
with
4832 additions
and
0 deletions
File moved
node_modules/.bin/jpeg-autorotate
0 → 120000
1 | +../jpeg-autorotate/src/cli.js | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/.bin/opencollective-postinstall
0 → 120000
1 | +../opencollective-postinstall/index.js | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
This diff is collapsed. Click to expand it.
node_modules/balanced-match/LICENSE.md
0 → 100644
1 | +(MIT) | ||
2 | + | ||
3 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
6 | +this software and associated documentation files (the "Software"), to deal in | ||
7 | +the Software without restriction, including without limitation the rights to | ||
8 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
9 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
10 | +so, subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in all | ||
13 | +copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
17 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
18 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
19 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
20 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
21 | +SOFTWARE. |
node_modules/balanced-match/README.md
0 → 100644
1 | +# balanced-match | ||
2 | + | ||
3 | +Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`. Supports regular expressions as well! | ||
4 | + | ||
5 | +[](http://travis-ci.org/juliangruber/balanced-match) | ||
6 | +[](https://www.npmjs.org/package/balanced-match) | ||
7 | + | ||
8 | +[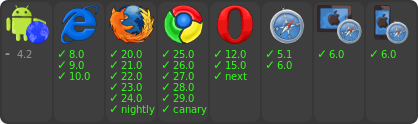](https://ci.testling.com/juliangruber/balanced-match) | ||
9 | + | ||
10 | +## Example | ||
11 | + | ||
12 | +Get the first matching pair of braces: | ||
13 | + | ||
14 | +```js | ||
15 | +var balanced = require('balanced-match'); | ||
16 | + | ||
17 | +console.log(balanced('{', '}', 'pre{in{nested}}post')); | ||
18 | +console.log(balanced('{', '}', 'pre{first}between{second}post')); | ||
19 | +console.log(balanced(/\s+\{\s+/, /\s+\}\s+/, 'pre { in{nest} } post')); | ||
20 | +``` | ||
21 | + | ||
22 | +The matches are: | ||
23 | + | ||
24 | +```bash | ||
25 | +$ node example.js | ||
26 | +{ start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' } | ||
27 | +{ start: 3, | ||
28 | + end: 9, | ||
29 | + pre: 'pre', | ||
30 | + body: 'first', | ||
31 | + post: 'between{second}post' } | ||
32 | +{ start: 3, end: 17, pre: 'pre', body: 'in{nest}', post: 'post' } | ||
33 | +``` | ||
34 | + | ||
35 | +## API | ||
36 | + | ||
37 | +### var m = balanced(a, b, str) | ||
38 | + | ||
39 | +For the first non-nested matching pair of `a` and `b` in `str`, return an | ||
40 | +object with those keys: | ||
41 | + | ||
42 | +* **start** the index of the first match of `a` | ||
43 | +* **end** the index of the matching `b` | ||
44 | +* **pre** the preamble, `a` and `b` not included | ||
45 | +* **body** the match, `a` and `b` not included | ||
46 | +* **post** the postscript, `a` and `b` not included | ||
47 | + | ||
48 | +If there's no match, `undefined` will be returned. | ||
49 | + | ||
50 | +If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']` and `{a}}` will match `['', 'a', '}']`. | ||
51 | + | ||
52 | +### var r = balanced.range(a, b, str) | ||
53 | + | ||
54 | +For the first non-nested matching pair of `a` and `b` in `str`, return an | ||
55 | +array with indexes: `[ <a index>, <b index> ]`. | ||
56 | + | ||
57 | +If there's no match, `undefined` will be returned. | ||
58 | + | ||
59 | +If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `[ 1, 3 ]` and `{a}}` will match `[0, 2]`. | ||
60 | + | ||
61 | +## Installation | ||
62 | + | ||
63 | +With [npm](https://npmjs.org) do: | ||
64 | + | ||
65 | +```bash | ||
66 | +npm install balanced-match | ||
67 | +``` | ||
68 | + | ||
69 | +## Security contact information | ||
70 | + | ||
71 | +To report a security vulnerability, please use the | ||
72 | +[Tidelift security contact](https://tidelift.com/security). | ||
73 | +Tidelift will coordinate the fix and disclosure. | ||
74 | + | ||
75 | +## License | ||
76 | + | ||
77 | +(MIT) | ||
78 | + | ||
79 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
80 | + | ||
81 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
82 | +this software and associated documentation files (the "Software"), to deal in | ||
83 | +the Software without restriction, including without limitation the rights to | ||
84 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
85 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
86 | +so, subject to the following conditions: | ||
87 | + | ||
88 | +The above copyright notice and this permission notice shall be included in all | ||
89 | +copies or substantial portions of the Software. | ||
90 | + | ||
91 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
92 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
93 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
94 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
95 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
96 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
97 | +SOFTWARE. |
node_modules/balanced-match/index.js
0 → 100644
1 | +'use strict'; | ||
2 | +module.exports = balanced; | ||
3 | +function balanced(a, b, str) { | ||
4 | + if (a instanceof RegExp) a = maybeMatch(a, str); | ||
5 | + if (b instanceof RegExp) b = maybeMatch(b, str); | ||
6 | + | ||
7 | + var r = range(a, b, str); | ||
8 | + | ||
9 | + return r && { | ||
10 | + start: r[0], | ||
11 | + end: r[1], | ||
12 | + pre: str.slice(0, r[0]), | ||
13 | + body: str.slice(r[0] + a.length, r[1]), | ||
14 | + post: str.slice(r[1] + b.length) | ||
15 | + }; | ||
16 | +} | ||
17 | + | ||
18 | +function maybeMatch(reg, str) { | ||
19 | + var m = str.match(reg); | ||
20 | + return m ? m[0] : null; | ||
21 | +} | ||
22 | + | ||
23 | +balanced.range = range; | ||
24 | +function range(a, b, str) { | ||
25 | + var begs, beg, left, right, result; | ||
26 | + var ai = str.indexOf(a); | ||
27 | + var bi = str.indexOf(b, ai + 1); | ||
28 | + var i = ai; | ||
29 | + | ||
30 | + if (ai >= 0 && bi > 0) { | ||
31 | + if(a===b) { | ||
32 | + return [ai, bi]; | ||
33 | + } | ||
34 | + begs = []; | ||
35 | + left = str.length; | ||
36 | + | ||
37 | + while (i >= 0 && !result) { | ||
38 | + if (i == ai) { | ||
39 | + begs.push(i); | ||
40 | + ai = str.indexOf(a, i + 1); | ||
41 | + } else if (begs.length == 1) { | ||
42 | + result = [ begs.pop(), bi ]; | ||
43 | + } else { | ||
44 | + beg = begs.pop(); | ||
45 | + if (beg < left) { | ||
46 | + left = beg; | ||
47 | + right = bi; | ||
48 | + } | ||
49 | + | ||
50 | + bi = str.indexOf(b, i + 1); | ||
51 | + } | ||
52 | + | ||
53 | + i = ai < bi && ai >= 0 ? ai : bi; | ||
54 | + } | ||
55 | + | ||
56 | + if (begs.length) { | ||
57 | + result = [ left, right ]; | ||
58 | + } | ||
59 | + } | ||
60 | + | ||
61 | + return result; | ||
62 | +} |
node_modules/balanced-match/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "balanced-match", | ||
3 | + "description": "Match balanced character pairs, like \"{\" and \"}\"", | ||
4 | + "version": "1.0.2", | ||
5 | + "repository": { | ||
6 | + "type": "git", | ||
7 | + "url": "git://github.com/juliangruber/balanced-match.git" | ||
8 | + }, | ||
9 | + "homepage": "https://github.com/juliangruber/balanced-match", | ||
10 | + "main": "index.js", | ||
11 | + "scripts": { | ||
12 | + "test": "tape test/test.js", | ||
13 | + "bench": "matcha test/bench.js" | ||
14 | + }, | ||
15 | + "devDependencies": { | ||
16 | + "matcha": "^0.7.0", | ||
17 | + "tape": "^4.6.0" | ||
18 | + }, | ||
19 | + "keywords": [ | ||
20 | + "match", | ||
21 | + "regexp", | ||
22 | + "test", | ||
23 | + "balanced", | ||
24 | + "parse" | ||
25 | + ], | ||
26 | + "author": { | ||
27 | + "name": "Julian Gruber", | ||
28 | + "email": "mail@juliangruber.com", | ||
29 | + "url": "http://juliangruber.com" | ||
30 | + }, | ||
31 | + "license": "MIT", | ||
32 | + "testling": { | ||
33 | + "files": "test/*.js", | ||
34 | + "browsers": [ | ||
35 | + "ie/8..latest", | ||
36 | + "firefox/20..latest", | ||
37 | + "firefox/nightly", | ||
38 | + "chrome/25..latest", | ||
39 | + "chrome/canary", | ||
40 | + "opera/12..latest", | ||
41 | + "opera/next", | ||
42 | + "safari/5.1..latest", | ||
43 | + "ipad/6.0..latest", | ||
44 | + "iphone/6.0..latest", | ||
45 | + "android-browser/4.2..latest" | ||
46 | + ] | ||
47 | + } | ||
48 | +} |
node_modules/blueimp-load-image/LICENSE.txt
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Copyright © 2011 Sebastian Tschan, https://blueimp.net | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
6 | +this software and associated documentation files (the "Software"), to deal in | ||
7 | +the Software without restriction, including without limitation the rights to | ||
8 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of | ||
9 | +the Software, and to permit persons to whom the Software is furnished to do so, | ||
10 | +subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in all | ||
13 | +copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS | ||
17 | +FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR | ||
18 | +COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER | ||
19 | +IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN | ||
20 | +CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
node_modules/blueimp-load-image/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/blueimp-load-image/js/index.js
0 → 100644
1 | +/* global module, require */ | ||
2 | + | ||
3 | +module.exports = require('./load-image') | ||
4 | + | ||
5 | +require('./load-image-scale') | ||
6 | +require('./load-image-meta') | ||
7 | +require('./load-image-fetch') | ||
8 | +require('./load-image-exif') | ||
9 | +require('./load-image-exif-map') | ||
10 | +require('./load-image-iptc') | ||
11 | +require('./load-image-iptc-map') | ||
12 | +require('./load-image-orientation') |
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
1 | +/* | ||
2 | + * JavaScript Load Image Fetch | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2017, Sebastian Tschan | ||
6 | + * https://blueimp.net | ||
7 | + * | ||
8 | + * Licensed under the MIT license: | ||
9 | + * https://opensource.org/licenses/MIT | ||
10 | + */ | ||
11 | + | ||
12 | +/* global define, module, require */ | ||
13 | + | ||
14 | +;(function (factory) { | ||
15 | + 'use strict' | ||
16 | + if (typeof define === 'function' && define.amd) { | ||
17 | + // Register as an anonymous AMD module: | ||
18 | + define(['./load-image'], factory) | ||
19 | + } else if (typeof module === 'object' && module.exports) { | ||
20 | + factory(require('./load-image')) | ||
21 | + } else { | ||
22 | + // Browser globals: | ||
23 | + factory(window.loadImage) | ||
24 | + } | ||
25 | +})(function (loadImage) { | ||
26 | + 'use strict' | ||
27 | + | ||
28 | + if (typeof fetch !== 'undefined' && typeof Request !== 'undefined') { | ||
29 | + loadImage.fetchBlob = function (url, callback, options) { | ||
30 | + fetch(new Request(url, options)) | ||
31 | + .then(function (response) { | ||
32 | + return response.blob() | ||
33 | + }) | ||
34 | + .then(callback) | ||
35 | + .catch(function (err) { | ||
36 | + callback(null, err) | ||
37 | + }) | ||
38 | + } | ||
39 | + } else if ( | ||
40 | + // Check for XHR2 support: | ||
41 | + typeof XMLHttpRequest !== 'undefined' && | ||
42 | + typeof ProgressEvent !== 'undefined' | ||
43 | + ) { | ||
44 | + loadImage.fetchBlob = function (url, callback, options) { | ||
45 | + // eslint-disable-next-line no-param-reassign | ||
46 | + options = options || {} | ||
47 | + var req = new XMLHttpRequest() | ||
48 | + req.open(options.method || 'GET', url) | ||
49 | + if (options.headers) { | ||
50 | + Object.keys(options.headers).forEach(function (key) { | ||
51 | + req.setRequestHeader(key, options.headers[key]) | ||
52 | + }) | ||
53 | + } | ||
54 | + req.withCredentials = options.credentials === 'include' | ||
55 | + req.responseType = 'blob' | ||
56 | + req.onload = function () { | ||
57 | + callback(req.response) | ||
58 | + } | ||
59 | + req.onerror = req.onabort = req.ontimeout = function (err) { | ||
60 | + callback(null, err) | ||
61 | + } | ||
62 | + req.send(options.body) | ||
63 | + } | ||
64 | + } | ||
65 | +}) |
1 | +/* | ||
2 | + * JavaScript Load Image IPTC Map | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2013, Sebastian Tschan | ||
6 | + * Copyright 2018, Dave Bevan | ||
7 | + * | ||
8 | + * IPTC tags mapping based on | ||
9 | + * https://iptc.org/standards/photo-metadata | ||
10 | + * https://exiftool.org/TagNames/IPTC.html | ||
11 | + * | ||
12 | + * Licensed under the MIT license: | ||
13 | + * https://opensource.org/licenses/MIT | ||
14 | + */ | ||
15 | + | ||
16 | +/* global define, module, require */ | ||
17 | + | ||
18 | +;(function (factory) { | ||
19 | + 'use strict' | ||
20 | + if (typeof define === 'function' && define.amd) { | ||
21 | + // Register as an anonymous AMD module: | ||
22 | + define(['./load-image', './load-image-iptc'], factory) | ||
23 | + } else if (typeof module === 'object' && module.exports) { | ||
24 | + factory(require('./load-image'), require('./load-image-iptc')) | ||
25 | + } else { | ||
26 | + // Browser globals: | ||
27 | + factory(window.loadImage) | ||
28 | + } | ||
29 | +})(function (loadImage) { | ||
30 | + 'use strict' | ||
31 | + | ||
32 | + var IptcMapProto = loadImage.IptcMap.prototype | ||
33 | + | ||
34 | + IptcMapProto.tags = { | ||
35 | + 0: 'ApplicationRecordVersion', | ||
36 | + 3: 'ObjectTypeReference', | ||
37 | + 4: 'ObjectAttributeReference', | ||
38 | + 5: 'ObjectName', | ||
39 | + 7: 'EditStatus', | ||
40 | + 8: 'EditorialUpdate', | ||
41 | + 10: 'Urgency', | ||
42 | + 12: 'SubjectReference', | ||
43 | + 15: 'Category', | ||
44 | + 20: 'SupplementalCategories', | ||
45 | + 22: 'FixtureIdentifier', | ||
46 | + 25: 'Keywords', | ||
47 | + 26: 'ContentLocationCode', | ||
48 | + 27: 'ContentLocationName', | ||
49 | + 30: 'ReleaseDate', | ||
50 | + 35: 'ReleaseTime', | ||
51 | + 37: 'ExpirationDate', | ||
52 | + 38: 'ExpirationTime', | ||
53 | + 40: 'SpecialInstructions', | ||
54 | + 42: 'ActionAdvised', | ||
55 | + 45: 'ReferenceService', | ||
56 | + 47: 'ReferenceDate', | ||
57 | + 50: 'ReferenceNumber', | ||
58 | + 55: 'DateCreated', | ||
59 | + 60: 'TimeCreated', | ||
60 | + 62: 'DigitalCreationDate', | ||
61 | + 63: 'DigitalCreationTime', | ||
62 | + 65: 'OriginatingProgram', | ||
63 | + 70: 'ProgramVersion', | ||
64 | + 75: 'ObjectCycle', | ||
65 | + 80: 'Byline', | ||
66 | + 85: 'BylineTitle', | ||
67 | + 90: 'City', | ||
68 | + 92: 'Sublocation', | ||
69 | + 95: 'State', | ||
70 | + 100: 'CountryCode', | ||
71 | + 101: 'Country', | ||
72 | + 103: 'OriginalTransmissionReference', | ||
73 | + 105: 'Headline', | ||
74 | + 110: 'Credit', | ||
75 | + 115: 'Source', | ||
76 | + 116: 'CopyrightNotice', | ||
77 | + 118: 'Contact', | ||
78 | + 120: 'Caption', | ||
79 | + 121: 'LocalCaption', | ||
80 | + 122: 'Writer', | ||
81 | + 125: 'RasterizedCaption', | ||
82 | + 130: 'ImageType', | ||
83 | + 131: 'ImageOrientation', | ||
84 | + 135: 'LanguageIdentifier', | ||
85 | + 150: 'AudioType', | ||
86 | + 151: 'AudioSamplingRate', | ||
87 | + 152: 'AudioSamplingResolution', | ||
88 | + 153: 'AudioDuration', | ||
89 | + 154: 'AudioOutcue', | ||
90 | + 184: 'JobID', | ||
91 | + 185: 'MasterDocumentID', | ||
92 | + 186: 'ShortDocumentID', | ||
93 | + 187: 'UniqueDocumentID', | ||
94 | + 188: 'OwnerID', | ||
95 | + 200: 'ObjectPreviewFileFormat', | ||
96 | + 201: 'ObjectPreviewFileVersion', | ||
97 | + 202: 'ObjectPreviewData', | ||
98 | + 221: 'Prefs', | ||
99 | + 225: 'ClassifyState', | ||
100 | + 228: 'SimilarityIndex', | ||
101 | + 230: 'DocumentNotes', | ||
102 | + 231: 'DocumentHistory', | ||
103 | + 232: 'ExifCameraInfo', | ||
104 | + 255: 'CatalogSets' | ||
105 | + } | ||
106 | + | ||
107 | + IptcMapProto.stringValues = { | ||
108 | + 10: { | ||
109 | + 0: '0 (reserved)', | ||
110 | + 1: '1 (most urgent)', | ||
111 | + 2: '2', | ||
112 | + 3: '3', | ||
113 | + 4: '4', | ||
114 | + 5: '5 (normal urgency)', | ||
115 | + 6: '6', | ||
116 | + 7: '7', | ||
117 | + 8: '8 (least urgent)', | ||
118 | + 9: '9 (user-defined priority)' | ||
119 | + }, | ||
120 | + 75: { | ||
121 | + a: 'Morning', | ||
122 | + b: 'Both Morning and Evening', | ||
123 | + p: 'Evening' | ||
124 | + }, | ||
125 | + 131: { | ||
126 | + L: 'Landscape', | ||
127 | + P: 'Portrait', | ||
128 | + S: 'Square' | ||
129 | + } | ||
130 | + } | ||
131 | + | ||
132 | + IptcMapProto.getText = function (id) { | ||
133 | + var value = this.get(id) | ||
134 | + var tagCode = this.map[id] | ||
135 | + var stringValue = this.stringValues[tagCode] | ||
136 | + if (stringValue) return stringValue[value] | ||
137 | + return String(value) | ||
138 | + } | ||
139 | + | ||
140 | + IptcMapProto.getAll = function () { | ||
141 | + var map = {} | ||
142 | + var prop | ||
143 | + var name | ||
144 | + for (prop in this) { | ||
145 | + if (Object.prototype.hasOwnProperty.call(this, prop)) { | ||
146 | + name = this.tags[prop] | ||
147 | + if (name) map[name] = this.getText(name) | ||
148 | + } | ||
149 | + } | ||
150 | + return map | ||
151 | + } | ||
152 | + | ||
153 | + IptcMapProto.getName = function (tagCode) { | ||
154 | + return this.tags[tagCode] | ||
155 | + } | ||
156 | + | ||
157 | + // Extend the map of tag names to tag codes: | ||
158 | + ;(function () { | ||
159 | + var tags = IptcMapProto.tags | ||
160 | + var map = IptcMapProto.map || {} | ||
161 | + var prop | ||
162 | + // Map the tag names to tags: | ||
163 | + for (prop in tags) { | ||
164 | + if (Object.prototype.hasOwnProperty.call(tags, prop)) { | ||
165 | + map[tags[prop]] = Number(prop) | ||
166 | + } | ||
167 | + } | ||
168 | + })() | ||
169 | +}) |
1 | +/* | ||
2 | + * JavaScript Load Image IPTC Parser | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2013, Sebastian Tschan | ||
6 | + * Copyright 2018, Dave Bevan | ||
7 | + * https://blueimp.net | ||
8 | + * | ||
9 | + * Licensed under the MIT license: | ||
10 | + * https://opensource.org/licenses/MIT | ||
11 | + */ | ||
12 | + | ||
13 | +/* global define, module, require, DataView */ | ||
14 | + | ||
15 | +;(function (factory) { | ||
16 | + 'use strict' | ||
17 | + if (typeof define === 'function' && define.amd) { | ||
18 | + // Register as an anonymous AMD module: | ||
19 | + define(['./load-image', './load-image-meta'], factory) | ||
20 | + } else if (typeof module === 'object' && module.exports) { | ||
21 | + factory(require('./load-image'), require('./load-image-meta')) | ||
22 | + } else { | ||
23 | + // Browser globals: | ||
24 | + factory(window.loadImage) | ||
25 | + } | ||
26 | +})(function (loadImage) { | ||
27 | + 'use strict' | ||
28 | + | ||
29 | + /** | ||
30 | + * IPTC tag map | ||
31 | + * | ||
32 | + * @name IptcMap | ||
33 | + * @class | ||
34 | + */ | ||
35 | + function IptcMap() {} | ||
36 | + | ||
37 | + IptcMap.prototype.map = { | ||
38 | + ObjectName: 5 | ||
39 | + } | ||
40 | + | ||
41 | + IptcMap.prototype.types = { | ||
42 | + 0: 'Uint16', // ApplicationRecordVersion | ||
43 | + 200: 'Uint16', // ObjectPreviewFileFormat | ||
44 | + 201: 'Uint16', // ObjectPreviewFileVersion | ||
45 | + 202: 'binary' // ObjectPreviewData | ||
46 | + } | ||
47 | + | ||
48 | + /** | ||
49 | + * Retrieves IPTC tag value | ||
50 | + * | ||
51 | + * @param {number|string} id IPTC tag code or name | ||
52 | + * @returns {object} IPTC tag value | ||
53 | + */ | ||
54 | + IptcMap.prototype.get = function (id) { | ||
55 | + return this[id] || this[this.map[id]] | ||
56 | + } | ||
57 | + | ||
58 | + /** | ||
59 | + * Retrieves string for the given DataView and range | ||
60 | + * | ||
61 | + * @param {DataView} dataView Data view interface | ||
62 | + * @param {number} offset Offset start | ||
63 | + * @param {number} length Offset length | ||
64 | + * @returns {string} String value | ||
65 | + */ | ||
66 | + function getStringValue(dataView, offset, length) { | ||
67 | + var outstr = '' | ||
68 | + var end = offset + length | ||
69 | + for (var n = offset; n < end; n += 1) { | ||
70 | + outstr += String.fromCharCode(dataView.getUint8(n)) | ||
71 | + } | ||
72 | + return outstr | ||
73 | + } | ||
74 | + | ||
75 | + /** | ||
76 | + * Retrieves tag value for the given DataView and range | ||
77 | + * | ||
78 | + * @param {number} tagCode Private IFD tag code | ||
79 | + * @param {IptcMap} map IPTC tag map | ||
80 | + * @param {DataView} dataView Data view interface | ||
81 | + * @param {number} offset Range start | ||
82 | + * @param {number} length Range length | ||
83 | + * @returns {object} Tag value | ||
84 | + */ | ||
85 | + function getTagValue(tagCode, map, dataView, offset, length) { | ||
86 | + if (map.types[tagCode] === 'binary') { | ||
87 | + return new Blob([dataView.buffer.slice(offset, offset + length)]) | ||
88 | + } | ||
89 | + if (map.types[tagCode] === 'Uint16') { | ||
90 | + return dataView.getUint16(offset) | ||
91 | + } | ||
92 | + return getStringValue(dataView, offset, length) | ||
93 | + } | ||
94 | + | ||
95 | + /** | ||
96 | + * Combines IPTC value with existing ones. | ||
97 | + * | ||
98 | + * @param {object} value Existing IPTC field value | ||
99 | + * @param {object} newValue New IPTC field value | ||
100 | + * @returns {object} Resulting IPTC field value | ||
101 | + */ | ||
102 | + function combineTagValues(value, newValue) { | ||
103 | + if (value === undefined) return newValue | ||
104 | + if (value instanceof Array) { | ||
105 | + value.push(newValue) | ||
106 | + return value | ||
107 | + } | ||
108 | + return [value, newValue] | ||
109 | + } | ||
110 | + | ||
111 | + /** | ||
112 | + * Parses IPTC tags. | ||
113 | + * | ||
114 | + * @param {DataView} dataView Data view interface | ||
115 | + * @param {number} segmentOffset Segment offset | ||
116 | + * @param {number} segmentLength Segment length | ||
117 | + * @param {object} data Data export object | ||
118 | + * @param {object} includeTags Map of tags to include | ||
119 | + * @param {object} excludeTags Map of tags to exclude | ||
120 | + */ | ||
121 | + function parseIptcTags( | ||
122 | + dataView, | ||
123 | + segmentOffset, | ||
124 | + segmentLength, | ||
125 | + data, | ||
126 | + includeTags, | ||
127 | + excludeTags | ||
128 | + ) { | ||
129 | + var value, tagSize, tagCode | ||
130 | + var segmentEnd = segmentOffset + segmentLength | ||
131 | + var offset = segmentOffset | ||
132 | + while (offset < segmentEnd) { | ||
133 | + if ( | ||
134 | + dataView.getUint8(offset) === 0x1c && // tag marker | ||
135 | + dataView.getUint8(offset + 1) === 0x02 // record number, only handles v2 | ||
136 | + ) { | ||
137 | + tagCode = dataView.getUint8(offset + 2) | ||
138 | + if ( | ||
139 | + (!includeTags || includeTags[tagCode]) && | ||
140 | + (!excludeTags || !excludeTags[tagCode]) | ||
141 | + ) { | ||
142 | + tagSize = dataView.getInt16(offset + 3) | ||
143 | + value = getTagValue(tagCode, data.iptc, dataView, offset + 5, tagSize) | ||
144 | + data.iptc[tagCode] = combineTagValues(data.iptc[tagCode], value) | ||
145 | + if (data.iptcOffsets) { | ||
146 | + data.iptcOffsets[tagCode] = offset | ||
147 | + } | ||
148 | + } | ||
149 | + } | ||
150 | + offset += 1 | ||
151 | + } | ||
152 | + } | ||
153 | + | ||
154 | + /** | ||
155 | + * Tests if field segment starts at offset. | ||
156 | + * | ||
157 | + * @param {DataView} dataView Data view interface | ||
158 | + * @param {number} offset Segment offset | ||
159 | + * @returns {boolean} True if '8BIM<EOT><EOT>' exists at offset | ||
160 | + */ | ||
161 | + function isSegmentStart(dataView, offset) { | ||
162 | + return ( | ||
163 | + dataView.getUint32(offset) === 0x3842494d && // Photoshop segment start | ||
164 | + dataView.getUint16(offset + 4) === 0x0404 // IPTC segment start | ||
165 | + ) | ||
166 | + } | ||
167 | + | ||
168 | + /** | ||
169 | + * Returns header length. | ||
170 | + * | ||
171 | + * @param {DataView} dataView Data view interface | ||
172 | + * @param {number} offset Segment offset | ||
173 | + * @returns {number} Header length | ||
174 | + */ | ||
175 | + function getHeaderLength(dataView, offset) { | ||
176 | + var length = dataView.getUint8(offset + 7) | ||
177 | + if (length % 2 !== 0) length += 1 | ||
178 | + // Check for pre photoshop 6 format | ||
179 | + if (length === 0) { | ||
180 | + // Always 4 | ||
181 | + length = 4 | ||
182 | + } | ||
183 | + return length | ||
184 | + } | ||
185 | + | ||
186 | + loadImage.parseIptcData = function (dataView, offset, length, data, options) { | ||
187 | + if (options.disableIptc) { | ||
188 | + return | ||
189 | + } | ||
190 | + var markerLength = offset + length | ||
191 | + while (offset + 8 < markerLength) { | ||
192 | + if (isSegmentStart(dataView, offset)) { | ||
193 | + var headerLength = getHeaderLength(dataView, offset) | ||
194 | + var segmentOffset = offset + 8 + headerLength | ||
195 | + if (segmentOffset > markerLength) { | ||
196 | + // eslint-disable-next-line no-console | ||
197 | + console.log('Invalid IPTC data: Invalid segment offset.') | ||
198 | + break | ||
199 | + } | ||
200 | + var segmentLength = dataView.getUint16(offset + 6 + headerLength) | ||
201 | + if (offset + segmentLength > markerLength) { | ||
202 | + // eslint-disable-next-line no-console | ||
203 | + console.log('Invalid IPTC data: Invalid segment size.') | ||
204 | + break | ||
205 | + } | ||
206 | + // Create the iptc object to store the tags: | ||
207 | + data.iptc = new IptcMap() | ||
208 | + if (!options.disableIptcOffsets) { | ||
209 | + data.iptcOffsets = new IptcMap() | ||
210 | + } | ||
211 | + parseIptcTags( | ||
212 | + dataView, | ||
213 | + segmentOffset, | ||
214 | + segmentLength, | ||
215 | + data, | ||
216 | + options.includeIptcTags, | ||
217 | + options.excludeIptcTags || { 202: true } // ObjectPreviewData | ||
218 | + ) | ||
219 | + return | ||
220 | + } | ||
221 | + // eslint-disable-next-line no-param-reassign | ||
222 | + offset += 1 | ||
223 | + } | ||
224 | + } | ||
225 | + | ||
226 | + // Registers this IPTC parser for the APP13 JPEG meta data segment: | ||
227 | + loadImage.metaDataParsers.jpeg[0xffed].push(loadImage.parseIptcData) | ||
228 | + | ||
229 | + loadImage.IptcMap = IptcMap | ||
230 | + | ||
231 | + // Adds the following properties to the parseMetaData callback data: | ||
232 | + // - iptc: The iptc tags, parsed by the parseIptcData method | ||
233 | + | ||
234 | + // Adds the following options to the parseMetaData method: | ||
235 | + // - disableIptc: Disables IPTC parsing when true. | ||
236 | + // - disableIptcOffsets: Disables storing IPTC tag offsets when true. | ||
237 | + // - includeIptcTags: A map of IPTC tags to include for parsing. | ||
238 | + // - excludeIptcTags: A map of IPTC tags to exclude from parsing. | ||
239 | +}) |
1 | +/* | ||
2 | + * JavaScript Load Image Meta | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2013, Sebastian Tschan | ||
6 | + * https://blueimp.net | ||
7 | + * | ||
8 | + * Image meta data handling implementation | ||
9 | + * based on the help and contribution of | ||
10 | + * Achim Stöhr. | ||
11 | + * | ||
12 | + * Licensed under the MIT license: | ||
13 | + * https://opensource.org/licenses/MIT | ||
14 | + */ | ||
15 | + | ||
16 | +/* global define, module, require, DataView, Uint8Array */ | ||
17 | + | ||
18 | +;(function (factory) { | ||
19 | + 'use strict' | ||
20 | + if (typeof define === 'function' && define.amd) { | ||
21 | + // Register as an anonymous AMD module: | ||
22 | + define(['./load-image'], factory) | ||
23 | + } else if (typeof module === 'object' && module.exports) { | ||
24 | + factory(require('./load-image')) | ||
25 | + } else { | ||
26 | + // Browser globals: | ||
27 | + factory(window.loadImage) | ||
28 | + } | ||
29 | +})(function (loadImage) { | ||
30 | + 'use strict' | ||
31 | + | ||
32 | + var hasblobSlice = | ||
33 | + typeof Blob !== 'undefined' && | ||
34 | + (Blob.prototype.slice || | ||
35 | + Blob.prototype.webkitSlice || | ||
36 | + Blob.prototype.mozSlice) | ||
37 | + | ||
38 | + loadImage.blobSlice = | ||
39 | + hasblobSlice && | ||
40 | + function () { | ||
41 | + var slice = this.slice || this.webkitSlice || this.mozSlice | ||
42 | + return slice.apply(this, arguments) | ||
43 | + } | ||
44 | + | ||
45 | + loadImage.metaDataParsers = { | ||
46 | + jpeg: { | ||
47 | + 0xffe1: [], // APP1 marker | ||
48 | + 0xffed: [] // APP13 marker | ||
49 | + } | ||
50 | + } | ||
51 | + | ||
52 | + // Parses image meta data and calls the callback with an object argument | ||
53 | + // with the following properties: | ||
54 | + // * imageHead: The complete image head as ArrayBuffer (Uint8Array for IE10) | ||
55 | + // The options argument accepts an object and supports the following | ||
56 | + // properties: | ||
57 | + // * maxMetaDataSize: Defines the maximum number of bytes to parse. | ||
58 | + // * disableImageHead: Disables creating the imageHead property. | ||
59 | + loadImage.parseMetaData = function (file, callback, options, data) { | ||
60 | + // eslint-disable-next-line no-param-reassign | ||
61 | + options = options || {} | ||
62 | + // eslint-disable-next-line no-param-reassign | ||
63 | + data = data || {} | ||
64 | + var that = this | ||
65 | + // 256 KiB should contain all EXIF/ICC/IPTC segments: | ||
66 | + var maxMetaDataSize = options.maxMetaDataSize || 262144 | ||
67 | + var noMetaData = !( | ||
68 | + typeof DataView !== 'undefined' && | ||
69 | + file && | ||
70 | + file.size >= 12 && | ||
71 | + file.type === 'image/jpeg' && | ||
72 | + loadImage.blobSlice | ||
73 | + ) | ||
74 | + if ( | ||
75 | + noMetaData || | ||
76 | + !loadImage.readFile( | ||
77 | + loadImage.blobSlice.call(file, 0, maxMetaDataSize), | ||
78 | + function (e) { | ||
79 | + if (e.target.error) { | ||
80 | + // FileReader error | ||
81 | + // eslint-disable-next-line no-console | ||
82 | + console.log(e.target.error) | ||
83 | + callback(data) | ||
84 | + return | ||
85 | + } | ||
86 | + // Note on endianness: | ||
87 | + // Since the marker and length bytes in JPEG files are always | ||
88 | + // stored in big endian order, we can leave the endian parameter | ||
89 | + // of the DataView methods undefined, defaulting to big endian. | ||
90 | + var buffer = e.target.result | ||
91 | + var dataView = new DataView(buffer) | ||
92 | + var offset = 2 | ||
93 | + var maxOffset = dataView.byteLength - 4 | ||
94 | + var headLength = offset | ||
95 | + var markerBytes | ||
96 | + var markerLength | ||
97 | + var parsers | ||
98 | + var i | ||
99 | + // Check for the JPEG marker (0xffd8): | ||
100 | + if (dataView.getUint16(0) === 0xffd8) { | ||
101 | + while (offset < maxOffset) { | ||
102 | + markerBytes = dataView.getUint16(offset) | ||
103 | + // Search for APPn (0xffeN) and COM (0xfffe) markers, | ||
104 | + // which contain application-specific meta-data like | ||
105 | + // Exif, ICC and IPTC data and text comments: | ||
106 | + if ( | ||
107 | + (markerBytes >= 0xffe0 && markerBytes <= 0xffef) || | ||
108 | + markerBytes === 0xfffe | ||
109 | + ) { | ||
110 | + // The marker bytes (2) are always followed by | ||
111 | + // the length bytes (2), indicating the length of the | ||
112 | + // marker segment, which includes the length bytes, | ||
113 | + // but not the marker bytes, so we add 2: | ||
114 | + markerLength = dataView.getUint16(offset + 2) + 2 | ||
115 | + if (offset + markerLength > dataView.byteLength) { | ||
116 | + // eslint-disable-next-line no-console | ||
117 | + console.log('Invalid meta data: Invalid segment size.') | ||
118 | + break | ||
119 | + } | ||
120 | + parsers = loadImage.metaDataParsers.jpeg[markerBytes] | ||
121 | + if (parsers && !options.disableMetaDataParsers) { | ||
122 | + for (i = 0; i < parsers.length; i += 1) { | ||
123 | + parsers[i].call( | ||
124 | + that, | ||
125 | + dataView, | ||
126 | + offset, | ||
127 | + markerLength, | ||
128 | + data, | ||
129 | + options | ||
130 | + ) | ||
131 | + } | ||
132 | + } | ||
133 | + offset += markerLength | ||
134 | + headLength = offset | ||
135 | + } else { | ||
136 | + // Not an APPn or COM marker, probably safe to | ||
137 | + // assume that this is the end of the meta data | ||
138 | + break | ||
139 | + } | ||
140 | + } | ||
141 | + // Meta length must be longer than JPEG marker (2) | ||
142 | + // plus APPn marker (2), followed by length bytes (2): | ||
143 | + if (!options.disableImageHead && headLength > 6) { | ||
144 | + if (buffer.slice) { | ||
145 | + data.imageHead = buffer.slice(0, headLength) | ||
146 | + } else { | ||
147 | + // Workaround for IE10, which does not yet | ||
148 | + // support ArrayBuffer.slice: | ||
149 | + data.imageHead = new Uint8Array(buffer).subarray(0, headLength) | ||
150 | + } | ||
151 | + } | ||
152 | + } else { | ||
153 | + // eslint-disable-next-line no-console | ||
154 | + console.log('Invalid JPEG file: Missing JPEG marker.') | ||
155 | + } | ||
156 | + callback(data) | ||
157 | + }, | ||
158 | + 'readAsArrayBuffer' | ||
159 | + ) | ||
160 | + ) { | ||
161 | + callback(data) | ||
162 | + } | ||
163 | + } | ||
164 | + | ||
165 | + // Replaces the image head of a JPEG blob with the given one. | ||
166 | + // Calls the callback with the new Blob: | ||
167 | + loadImage.replaceHead = function (blob, head, callback) { | ||
168 | + loadImage.parseMetaData( | ||
169 | + blob, | ||
170 | + function (data) { | ||
171 | + callback( | ||
172 | + new Blob( | ||
173 | + [head, loadImage.blobSlice.call(blob, data.imageHead.byteLength)], | ||
174 | + { type: 'image/jpeg' } | ||
175 | + ) | ||
176 | + ) | ||
177 | + }, | ||
178 | + { maxMetaDataSize: 256, disableMetaDataParsers: true } | ||
179 | + ) | ||
180 | + } | ||
181 | + | ||
182 | + var originalTransform = loadImage.transform | ||
183 | + loadImage.transform = function (img, options, callback, file, data) { | ||
184 | + if (loadImage.hasMetaOption(options)) { | ||
185 | + loadImage.parseMetaData( | ||
186 | + file, | ||
187 | + function (data) { | ||
188 | + originalTransform.call(loadImage, img, options, callback, file, data) | ||
189 | + }, | ||
190 | + options, | ||
191 | + data | ||
192 | + ) | ||
193 | + } else { | ||
194 | + originalTransform.apply(loadImage, arguments) | ||
195 | + } | ||
196 | + } | ||
197 | +}) |
1 | +/* | ||
2 | + * JavaScript Load Image Orientation | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2013, Sebastian Tschan | ||
6 | + * https://blueimp.net | ||
7 | + * | ||
8 | + * Licensed under the MIT license: | ||
9 | + * https://opensource.org/licenses/MIT | ||
10 | + */ | ||
11 | + | ||
12 | +/* global define, module, require */ | ||
13 | + | ||
14 | +;(function (factory) { | ||
15 | + 'use strict' | ||
16 | + if (typeof define === 'function' && define.amd) { | ||
17 | + // Register as an anonymous AMD module: | ||
18 | + define(['./load-image', './load-image-scale', './load-image-meta'], factory) | ||
19 | + } else if (typeof module === 'object' && module.exports) { | ||
20 | + factory( | ||
21 | + require('./load-image'), | ||
22 | + require('./load-image-scale'), | ||
23 | + require('./load-image-meta') | ||
24 | + ) | ||
25 | + } else { | ||
26 | + // Browser globals: | ||
27 | + factory(window.loadImage) | ||
28 | + } | ||
29 | +})(function (loadImage) { | ||
30 | + 'use strict' | ||
31 | + | ||
32 | + var originalHasCanvasOption = loadImage.hasCanvasOption | ||
33 | + var originalHasMetaOption = loadImage.hasMetaOption | ||
34 | + var originalTransformCoordinates = loadImage.transformCoordinates | ||
35 | + var originalGetTransformedOptions = loadImage.getTransformedOptions | ||
36 | + | ||
37 | + ;(function () { | ||
38 | + // black 2x1 JPEG, with the following meta information set: | ||
39 | + // - EXIF Orientation: 6 (Rotated 90° CCW) | ||
40 | + var testImageURL = | ||
41 | + 'data:image/jpeg;base64,/9j/4QAiRXhpZgAATU0AKgAAAAgAAQESAAMAAAABAAYAAAA' + | ||
42 | + 'AAAD/2wCEAAEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBA' + | ||
43 | + 'QEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQE' + | ||
44 | + 'BAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAf/AABEIAAEAAgMBEQACEQEDEQH/x' + | ||
45 | + 'ABKAAEAAAAAAAAAAAAAAAAAAAALEAEAAAAAAAAAAAAAAAAAAAAAAQEAAAAAAAAAAAAAAAA' + | ||
46 | + 'AAAAAEQEAAAAAAAAAAAAAAAAAAAAA/9oADAMBAAIRAxEAPwA/8H//2Q==' | ||
47 | + var img = document.createElement('img') | ||
48 | + img.onload = function () { | ||
49 | + // Check if browser supports automatic image orientation: | ||
50 | + loadImage.orientation = img.width === 1 && img.height === 2 | ||
51 | + } | ||
52 | + img.src = testImageURL | ||
53 | + })() | ||
54 | + | ||
55 | + // Determines if the target image should be a canvas element: | ||
56 | + loadImage.hasCanvasOption = function (options) { | ||
57 | + return ( | ||
58 | + (!!options.orientation === true && !loadImage.orientation) || | ||
59 | + (options.orientation > 1 && options.orientation < 9) || | ||
60 | + originalHasCanvasOption.call(loadImage, options) | ||
61 | + ) | ||
62 | + } | ||
63 | + | ||
64 | + // Determines if meta data should be loaded automatically: | ||
65 | + loadImage.hasMetaOption = function (options) { | ||
66 | + return ( | ||
67 | + (options && options.orientation === true && !loadImage.orientation) || | ||
68 | + originalHasMetaOption.call(loadImage, options) | ||
69 | + ) | ||
70 | + } | ||
71 | + | ||
72 | + // Transform image orientation based on | ||
73 | + // the given EXIF orientation option: | ||
74 | + loadImage.transformCoordinates = function (canvas, options) { | ||
75 | + originalTransformCoordinates.call(loadImage, canvas, options) | ||
76 | + var ctx = canvas.getContext('2d') | ||
77 | + var width = canvas.width | ||
78 | + var height = canvas.height | ||
79 | + var styleWidth = canvas.style.width | ||
80 | + var styleHeight = canvas.style.height | ||
81 | + var orientation = options.orientation | ||
82 | + if (!(orientation > 1 && orientation < 9)) { | ||
83 | + return | ||
84 | + } | ||
85 | + if (orientation > 4) { | ||
86 | + canvas.width = height | ||
87 | + canvas.height = width | ||
88 | + canvas.style.width = styleHeight | ||
89 | + canvas.style.height = styleWidth | ||
90 | + } | ||
91 | + switch (orientation) { | ||
92 | + case 2: | ||
93 | + // horizontal flip | ||
94 | + ctx.translate(width, 0) | ||
95 | + ctx.scale(-1, 1) | ||
96 | + break | ||
97 | + case 3: | ||
98 | + // 180° rotate left | ||
99 | + ctx.translate(width, height) | ||
100 | + ctx.rotate(Math.PI) | ||
101 | + break | ||
102 | + case 4: | ||
103 | + // vertical flip | ||
104 | + ctx.translate(0, height) | ||
105 | + ctx.scale(1, -1) | ||
106 | + break | ||
107 | + case 5: | ||
108 | + // vertical flip + 90 rotate right | ||
109 | + ctx.rotate(0.5 * Math.PI) | ||
110 | + ctx.scale(1, -1) | ||
111 | + break | ||
112 | + case 6: | ||
113 | + // 90° rotate right | ||
114 | + ctx.rotate(0.5 * Math.PI) | ||
115 | + ctx.translate(0, -height) | ||
116 | + break | ||
117 | + case 7: | ||
118 | + // horizontal flip + 90 rotate right | ||
119 | + ctx.rotate(0.5 * Math.PI) | ||
120 | + ctx.translate(width, -height) | ||
121 | + ctx.scale(-1, 1) | ||
122 | + break | ||
123 | + case 8: | ||
124 | + // 90° rotate left | ||
125 | + ctx.rotate(-0.5 * Math.PI) | ||
126 | + ctx.translate(-width, 0) | ||
127 | + break | ||
128 | + } | ||
129 | + } | ||
130 | + | ||
131 | + // Transforms coordinate and dimension options | ||
132 | + // based on the given orientation option: | ||
133 | + loadImage.getTransformedOptions = function (img, opts, data) { | ||
134 | + var options = originalGetTransformedOptions.call(loadImage, img, opts) | ||
135 | + var orientation = options.orientation | ||
136 | + var newOptions | ||
137 | + var i | ||
138 | + if (orientation === true) { | ||
139 | + if (loadImage.orientation) { | ||
140 | + // Browser supports automatic image orientation | ||
141 | + return options | ||
142 | + } | ||
143 | + orientation = data && data.exif && data.exif.get('Orientation') | ||
144 | + } | ||
145 | + if (!(orientation > 1 && orientation < 9)) { | ||
146 | + return options | ||
147 | + } | ||
148 | + newOptions = {} | ||
149 | + for (i in options) { | ||
150 | + if (Object.prototype.hasOwnProperty.call(options, i)) { | ||
151 | + newOptions[i] = options[i] | ||
152 | + } | ||
153 | + } | ||
154 | + newOptions.orientation = orientation | ||
155 | + switch (orientation) { | ||
156 | + case 2: | ||
157 | + // horizontal flip | ||
158 | + newOptions.left = options.right | ||
159 | + newOptions.right = options.left | ||
160 | + break | ||
161 | + case 3: | ||
162 | + // 180° rotate left | ||
163 | + newOptions.left = options.right | ||
164 | + newOptions.top = options.bottom | ||
165 | + newOptions.right = options.left | ||
166 | + newOptions.bottom = options.top | ||
167 | + break | ||
168 | + case 4: | ||
169 | + // vertical flip | ||
170 | + newOptions.top = options.bottom | ||
171 | + newOptions.bottom = options.top | ||
172 | + break | ||
173 | + case 5: | ||
174 | + // vertical flip + 90 rotate right | ||
175 | + newOptions.left = options.top | ||
176 | + newOptions.top = options.left | ||
177 | + newOptions.right = options.bottom | ||
178 | + newOptions.bottom = options.right | ||
179 | + break | ||
180 | + case 6: | ||
181 | + // 90° rotate right | ||
182 | + newOptions.left = options.top | ||
183 | + newOptions.top = options.right | ||
184 | + newOptions.right = options.bottom | ||
185 | + newOptions.bottom = options.left | ||
186 | + break | ||
187 | + case 7: | ||
188 | + // horizontal flip + 90 rotate right | ||
189 | + newOptions.left = options.bottom | ||
190 | + newOptions.top = options.right | ||
191 | + newOptions.right = options.top | ||
192 | + newOptions.bottom = options.left | ||
193 | + break | ||
194 | + case 8: | ||
195 | + // 90° rotate left | ||
196 | + newOptions.left = options.bottom | ||
197 | + newOptions.top = options.left | ||
198 | + newOptions.right = options.top | ||
199 | + newOptions.bottom = options.right | ||
200 | + break | ||
201 | + } | ||
202 | + if (newOptions.orientation > 4) { | ||
203 | + newOptions.maxWidth = options.maxHeight | ||
204 | + newOptions.maxHeight = options.maxWidth | ||
205 | + newOptions.minWidth = options.minHeight | ||
206 | + newOptions.minHeight = options.minWidth | ||
207 | + newOptions.sourceWidth = options.sourceHeight | ||
208 | + newOptions.sourceHeight = options.sourceWidth | ||
209 | + } | ||
210 | + return newOptions | ||
211 | + } | ||
212 | +}) |
1 | +/* | ||
2 | + * JavaScript Load Image Scaling | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2011, Sebastian Tschan | ||
6 | + * https://blueimp.net | ||
7 | + * | ||
8 | + * Licensed under the MIT license: | ||
9 | + * https://opensource.org/licenses/MIT | ||
10 | + */ | ||
11 | + | ||
12 | +/* global define, module, require */ | ||
13 | + | ||
14 | +;(function (factory) { | ||
15 | + 'use strict' | ||
16 | + if (typeof define === 'function' && define.amd) { | ||
17 | + // Register as an anonymous AMD module: | ||
18 | + define(['./load-image'], factory) | ||
19 | + } else if (typeof module === 'object' && module.exports) { | ||
20 | + factory(require('./load-image')) | ||
21 | + } else { | ||
22 | + // Browser globals: | ||
23 | + factory(window.loadImage) | ||
24 | + } | ||
25 | +})(function (loadImage) { | ||
26 | + 'use strict' | ||
27 | + | ||
28 | + var originalTransform = loadImage.transform | ||
29 | + | ||
30 | + loadImage.transform = function (img, options, callback, file, data) { | ||
31 | + originalTransform.call( | ||
32 | + loadImage, | ||
33 | + loadImage.scale(img, options, data), | ||
34 | + options, | ||
35 | + callback, | ||
36 | + file, | ||
37 | + data | ||
38 | + ) | ||
39 | + } | ||
40 | + | ||
41 | + // Transform image coordinates, allows to override e.g. | ||
42 | + // the canvas orientation based on the orientation option, | ||
43 | + // gets canvas, options passed as arguments: | ||
44 | + loadImage.transformCoordinates = function () {} | ||
45 | + | ||
46 | + // Returns transformed options, allows to override e.g. | ||
47 | + // maxWidth, maxHeight and crop options based on the aspectRatio. | ||
48 | + // gets img, options passed as arguments: | ||
49 | + loadImage.getTransformedOptions = function (img, options) { | ||
50 | + var aspectRatio = options.aspectRatio | ||
51 | + var newOptions | ||
52 | + var i | ||
53 | + var width | ||
54 | + var height | ||
55 | + if (!aspectRatio) { | ||
56 | + return options | ||
57 | + } | ||
58 | + newOptions = {} | ||
59 | + for (i in options) { | ||
60 | + if (Object.prototype.hasOwnProperty.call(options, i)) { | ||
61 | + newOptions[i] = options[i] | ||
62 | + } | ||
63 | + } | ||
64 | + newOptions.crop = true | ||
65 | + width = img.naturalWidth || img.width | ||
66 | + height = img.naturalHeight || img.height | ||
67 | + if (width / height > aspectRatio) { | ||
68 | + newOptions.maxWidth = height * aspectRatio | ||
69 | + newOptions.maxHeight = height | ||
70 | + } else { | ||
71 | + newOptions.maxWidth = width | ||
72 | + newOptions.maxHeight = width / aspectRatio | ||
73 | + } | ||
74 | + return newOptions | ||
75 | + } | ||
76 | + | ||
77 | + // Canvas render method, allows to implement a different rendering algorithm: | ||
78 | + loadImage.renderImageToCanvas = function ( | ||
79 | + canvas, | ||
80 | + img, | ||
81 | + sourceX, | ||
82 | + sourceY, | ||
83 | + sourceWidth, | ||
84 | + sourceHeight, | ||
85 | + destX, | ||
86 | + destY, | ||
87 | + destWidth, | ||
88 | + destHeight, | ||
89 | + options | ||
90 | + ) { | ||
91 | + var ctx = canvas.getContext('2d') | ||
92 | + if (options.imageSmoothingEnabled === false) { | ||
93 | + ctx.imageSmoothingEnabled = false | ||
94 | + } else if (options.imageSmoothingQuality) { | ||
95 | + ctx.imageSmoothingQuality = options.imageSmoothingQuality | ||
96 | + } | ||
97 | + ctx.drawImage( | ||
98 | + img, | ||
99 | + sourceX, | ||
100 | + sourceY, | ||
101 | + sourceWidth, | ||
102 | + sourceHeight, | ||
103 | + destX, | ||
104 | + destY, | ||
105 | + destWidth, | ||
106 | + destHeight | ||
107 | + ) | ||
108 | + return canvas | ||
109 | + } | ||
110 | + | ||
111 | + // Determines if the target image should be a canvas element: | ||
112 | + loadImage.hasCanvasOption = function (options) { | ||
113 | + return options.canvas || options.crop || !!options.aspectRatio | ||
114 | + } | ||
115 | + | ||
116 | + // Scales and/or crops the given image (img or canvas HTML element) | ||
117 | + // using the given options. | ||
118 | + // Returns a canvas object if the browser supports canvas | ||
119 | + // and the hasCanvasOption method returns true or a canvas | ||
120 | + // object is passed as image, else the scaled image: | ||
121 | + loadImage.scale = function (img, options, data) { | ||
122 | + // eslint-disable-next-line no-param-reassign | ||
123 | + options = options || {} | ||
124 | + var canvas = document.createElement('canvas') | ||
125 | + var useCanvas = | ||
126 | + img.getContext || | ||
127 | + (loadImage.hasCanvasOption(options) && canvas.getContext) | ||
128 | + var width = img.naturalWidth || img.width | ||
129 | + var height = img.naturalHeight || img.height | ||
130 | + var destWidth = width | ||
131 | + var destHeight = height | ||
132 | + var maxWidth | ||
133 | + var maxHeight | ||
134 | + var minWidth | ||
135 | + var minHeight | ||
136 | + var sourceWidth | ||
137 | + var sourceHeight | ||
138 | + var sourceX | ||
139 | + var sourceY | ||
140 | + var pixelRatio | ||
141 | + var downsamplingRatio | ||
142 | + var tmp | ||
143 | + /** | ||
144 | + * Scales up image dimensions | ||
145 | + */ | ||
146 | + function scaleUp() { | ||
147 | + var scale = Math.max( | ||
148 | + (minWidth || destWidth) / destWidth, | ||
149 | + (minHeight || destHeight) / destHeight | ||
150 | + ) | ||
151 | + if (scale > 1) { | ||
152 | + destWidth *= scale | ||
153 | + destHeight *= scale | ||
154 | + } | ||
155 | + } | ||
156 | + /** | ||
157 | + * Scales down image dimensions | ||
158 | + */ | ||
159 | + function scaleDown() { | ||
160 | + var scale = Math.min( | ||
161 | + (maxWidth || destWidth) / destWidth, | ||
162 | + (maxHeight || destHeight) / destHeight | ||
163 | + ) | ||
164 | + if (scale < 1) { | ||
165 | + destWidth *= scale | ||
166 | + destHeight *= scale | ||
167 | + } | ||
168 | + } | ||
169 | + if (useCanvas) { | ||
170 | + // eslint-disable-next-line no-param-reassign | ||
171 | + options = loadImage.getTransformedOptions(img, options, data) | ||
172 | + sourceX = options.left || 0 | ||
173 | + sourceY = options.top || 0 | ||
174 | + if (options.sourceWidth) { | ||
175 | + sourceWidth = options.sourceWidth | ||
176 | + if (options.right !== undefined && options.left === undefined) { | ||
177 | + sourceX = width - sourceWidth - options.right | ||
178 | + } | ||
179 | + } else { | ||
180 | + sourceWidth = width - sourceX - (options.right || 0) | ||
181 | + } | ||
182 | + if (options.sourceHeight) { | ||
183 | + sourceHeight = options.sourceHeight | ||
184 | + if (options.bottom !== undefined && options.top === undefined) { | ||
185 | + sourceY = height - sourceHeight - options.bottom | ||
186 | + } | ||
187 | + } else { | ||
188 | + sourceHeight = height - sourceY - (options.bottom || 0) | ||
189 | + } | ||
190 | + destWidth = sourceWidth | ||
191 | + destHeight = sourceHeight | ||
192 | + } | ||
193 | + maxWidth = options.maxWidth | ||
194 | + maxHeight = options.maxHeight | ||
195 | + minWidth = options.minWidth | ||
196 | + minHeight = options.minHeight | ||
197 | + if (useCanvas && maxWidth && maxHeight && options.crop) { | ||
198 | + destWidth = maxWidth | ||
199 | + destHeight = maxHeight | ||
200 | + tmp = sourceWidth / sourceHeight - maxWidth / maxHeight | ||
201 | + if (tmp < 0) { | ||
202 | + sourceHeight = (maxHeight * sourceWidth) / maxWidth | ||
203 | + if (options.top === undefined && options.bottom === undefined) { | ||
204 | + sourceY = (height - sourceHeight) / 2 | ||
205 | + } | ||
206 | + } else if (tmp > 0) { | ||
207 | + sourceWidth = (maxWidth * sourceHeight) / maxHeight | ||
208 | + if (options.left === undefined && options.right === undefined) { | ||
209 | + sourceX = (width - sourceWidth) / 2 | ||
210 | + } | ||
211 | + } | ||
212 | + } else { | ||
213 | + if (options.contain || options.cover) { | ||
214 | + minWidth = maxWidth = maxWidth || minWidth | ||
215 | + minHeight = maxHeight = maxHeight || minHeight | ||
216 | + } | ||
217 | + if (options.cover) { | ||
218 | + scaleDown() | ||
219 | + scaleUp() | ||
220 | + } else { | ||
221 | + scaleUp() | ||
222 | + scaleDown() | ||
223 | + } | ||
224 | + } | ||
225 | + if (useCanvas) { | ||
226 | + pixelRatio = options.pixelRatio | ||
227 | + if (pixelRatio > 1) { | ||
228 | + canvas.style.width = destWidth + 'px' | ||
229 | + canvas.style.height = destHeight + 'px' | ||
230 | + destWidth *= pixelRatio | ||
231 | + destHeight *= pixelRatio | ||
232 | + canvas.getContext('2d').scale(pixelRatio, pixelRatio) | ||
233 | + } | ||
234 | + downsamplingRatio = options.downsamplingRatio | ||
235 | + if ( | ||
236 | + downsamplingRatio > 0 && | ||
237 | + downsamplingRatio < 1 && | ||
238 | + destWidth < sourceWidth && | ||
239 | + destHeight < sourceHeight | ||
240 | + ) { | ||
241 | + while (sourceWidth * downsamplingRatio > destWidth) { | ||
242 | + canvas.width = sourceWidth * downsamplingRatio | ||
243 | + canvas.height = sourceHeight * downsamplingRatio | ||
244 | + loadImage.renderImageToCanvas( | ||
245 | + canvas, | ||
246 | + img, | ||
247 | + sourceX, | ||
248 | + sourceY, | ||
249 | + sourceWidth, | ||
250 | + sourceHeight, | ||
251 | + 0, | ||
252 | + 0, | ||
253 | + canvas.width, | ||
254 | + canvas.height, | ||
255 | + options | ||
256 | + ) | ||
257 | + sourceX = 0 | ||
258 | + sourceY = 0 | ||
259 | + sourceWidth = canvas.width | ||
260 | + sourceHeight = canvas.height | ||
261 | + // eslint-disable-next-line no-param-reassign | ||
262 | + img = document.createElement('canvas') | ||
263 | + img.width = sourceWidth | ||
264 | + img.height = sourceHeight | ||
265 | + loadImage.renderImageToCanvas( | ||
266 | + img, | ||
267 | + canvas, | ||
268 | + 0, | ||
269 | + 0, | ||
270 | + sourceWidth, | ||
271 | + sourceHeight, | ||
272 | + 0, | ||
273 | + 0, | ||
274 | + sourceWidth, | ||
275 | + sourceHeight, | ||
276 | + options | ||
277 | + ) | ||
278 | + } | ||
279 | + } | ||
280 | + canvas.width = destWidth | ||
281 | + canvas.height = destHeight | ||
282 | + loadImage.transformCoordinates(canvas, options) | ||
283 | + return loadImage.renderImageToCanvas( | ||
284 | + canvas, | ||
285 | + img, | ||
286 | + sourceX, | ||
287 | + sourceY, | ||
288 | + sourceWidth, | ||
289 | + sourceHeight, | ||
290 | + 0, | ||
291 | + 0, | ||
292 | + destWidth, | ||
293 | + destHeight, | ||
294 | + options | ||
295 | + ) | ||
296 | + } | ||
297 | + img.width = destWidth | ||
298 | + img.height = destHeight | ||
299 | + return img | ||
300 | + } | ||
301 | +}) |
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
1 | +/* | ||
2 | + * JavaScript Load Image | ||
3 | + * https://github.com/blueimp/JavaScript-Load-Image | ||
4 | + * | ||
5 | + * Copyright 2011, Sebastian Tschan | ||
6 | + * https://blueimp.net | ||
7 | + * | ||
8 | + * Licensed under the MIT license: | ||
9 | + * https://opensource.org/licenses/MIT | ||
10 | + */ | ||
11 | + | ||
12 | +/* global define, webkitURL, module */ | ||
13 | + | ||
14 | +;(function ($) { | ||
15 | + 'use strict' | ||
16 | + | ||
17 | + /** | ||
18 | + * Loads an image for a given File object. | ||
19 | + * Invokes the callback with an img or optional canvas element | ||
20 | + * (if supported by the browser) as parameter:. | ||
21 | + * | ||
22 | + * @param {File|Blob|string} file File or Blob object or image URL | ||
23 | + * @param {Function} [callback] Image load event callback | ||
24 | + * @param {object} [options] Options object | ||
25 | + * @returns {HTMLImageElement|HTMLCanvasElement|FileReader} image object | ||
26 | + */ | ||
27 | + function loadImage(file, callback, options) { | ||
28 | + var img = document.createElement('img') | ||
29 | + var url | ||
30 | + /** | ||
31 | + * Callback for the fetchBlob call. | ||
32 | + * | ||
33 | + * @param {Blob} blob Blob object | ||
34 | + * @param {Error} err Error object | ||
35 | + */ | ||
36 | + function fetchBlobCallback(blob, err) { | ||
37 | + if (err) console.log(err) // eslint-disable-line no-console | ||
38 | + if (blob && loadImage.isInstanceOf('Blob', blob)) { | ||
39 | + // eslint-disable-next-line no-param-reassign | ||
40 | + file = blob | ||
41 | + url = loadImage.createObjectURL(file) | ||
42 | + } else { | ||
43 | + url = file | ||
44 | + if (options && options.crossOrigin) { | ||
45 | + img.crossOrigin = options.crossOrigin | ||
46 | + } | ||
47 | + } | ||
48 | + img.src = url | ||
49 | + } | ||
50 | + img.onerror = function (event) { | ||
51 | + return loadImage.onerror(img, event, file, url, callback, options) | ||
52 | + } | ||
53 | + img.onload = function (event) { | ||
54 | + return loadImage.onload(img, event, file, url, callback, options) | ||
55 | + } | ||
56 | + if (typeof file === 'string') { | ||
57 | + if (loadImage.hasMetaOption(options)) { | ||
58 | + loadImage.fetchBlob(file, fetchBlobCallback, options) | ||
59 | + } else { | ||
60 | + fetchBlobCallback() | ||
61 | + } | ||
62 | + return img | ||
63 | + } else if ( | ||
64 | + loadImage.isInstanceOf('Blob', file) || | ||
65 | + // Files are also Blob instances, but some browsers | ||
66 | + // (Firefox 3.6) support the File API but not Blobs: | ||
67 | + loadImage.isInstanceOf('File', file) | ||
68 | + ) { | ||
69 | + url = loadImage.createObjectURL(file) | ||
70 | + if (url) { | ||
71 | + img.src = url | ||
72 | + return img | ||
73 | + } | ||
74 | + return loadImage.readFile(file, function (e) { | ||
75 | + var target = e.target | ||
76 | + if (target && target.result) { | ||
77 | + img.src = target.result | ||
78 | + } else if (callback) { | ||
79 | + callback(e) | ||
80 | + } | ||
81 | + }) | ||
82 | + } | ||
83 | + } | ||
84 | + // The check for URL.revokeObjectURL fixes an issue with Opera 12, | ||
85 | + // which provides URL.createObjectURL but doesn't properly implement it: | ||
86 | + var urlAPI = | ||
87 | + ($.createObjectURL && $) || | ||
88 | + ($.URL && URL.revokeObjectURL && URL) || | ||
89 | + ($.webkitURL && webkitURL) | ||
90 | + | ||
91 | + /** | ||
92 | + * Helper function to revoke an object URL | ||
93 | + * | ||
94 | + * @param {string} url Blob Object URL | ||
95 | + * @param {object} [options] Options object | ||
96 | + */ | ||
97 | + function revokeHelper(url, options) { | ||
98 | + if (url && url.slice(0, 5) === 'blob:' && !(options && options.noRevoke)) { | ||
99 | + loadImage.revokeObjectURL(url) | ||
100 | + } | ||
101 | + } | ||
102 | + | ||
103 | + // Determines if meta data should be loaded automatically. | ||
104 | + // Requires the load image meta extension to load meta data. | ||
105 | + loadImage.hasMetaOption = function (options) { | ||
106 | + return options && options.meta | ||
107 | + } | ||
108 | + | ||
109 | + // If the callback given to this function returns a blob, it is used as image | ||
110 | + // source instead of the original url and overrides the file argument used in | ||
111 | + // the onload and onerror event callbacks: | ||
112 | + loadImage.fetchBlob = function (url, callback) { | ||
113 | + callback() | ||
114 | + } | ||
115 | + | ||
116 | + loadImage.isInstanceOf = function (type, obj) { | ||
117 | + // Cross-frame instanceof check | ||
118 | + return Object.prototype.toString.call(obj) === '[object ' + type + ']' | ||
119 | + } | ||
120 | + | ||
121 | + loadImage.transform = function (img, options, callback, file, data) { | ||
122 | + callback(img, data) | ||
123 | + } | ||
124 | + | ||
125 | + loadImage.onerror = function (img, event, file, url, callback, options) { | ||
126 | + revokeHelper(url, options) | ||
127 | + if (callback) { | ||
128 | + callback.call(img, event) | ||
129 | + } | ||
130 | + } | ||
131 | + | ||
132 | + loadImage.onload = function (img, event, file, url, callback, options) { | ||
133 | + revokeHelper(url, options) | ||
134 | + if (callback) { | ||
135 | + loadImage.transform(img, options, callback, file, { | ||
136 | + originalWidth: img.naturalWidth || img.width, | ||
137 | + originalHeight: img.naturalHeight || img.height | ||
138 | + }) | ||
139 | + } | ||
140 | + } | ||
141 | + | ||
142 | + loadImage.createObjectURL = function (file) { | ||
143 | + return urlAPI ? urlAPI.createObjectURL(file) : false | ||
144 | + } | ||
145 | + | ||
146 | + loadImage.revokeObjectURL = function (url) { | ||
147 | + return urlAPI ? urlAPI.revokeObjectURL(url) : false | ||
148 | + } | ||
149 | + | ||
150 | + // Loads a given File object via FileReader interface, | ||
151 | + // invokes the callback with the event object (load or error). | ||
152 | + // The result can be read via event.target.result: | ||
153 | + loadImage.readFile = function (file, callback, method) { | ||
154 | + if ($.FileReader) { | ||
155 | + var fileReader = new FileReader() | ||
156 | + fileReader.onload = fileReader.onerror = callback | ||
157 | + // eslint-disable-next-line no-param-reassign | ||
158 | + method = method || 'readAsDataURL' | ||
159 | + if (fileReader[method]) { | ||
160 | + fileReader[method](file) | ||
161 | + return fileReader | ||
162 | + } | ||
163 | + } | ||
164 | + return false | ||
165 | + } | ||
166 | + | ||
167 | + if (typeof define === 'function' && define.amd) { | ||
168 | + define(function () { | ||
169 | + return loadImage | ||
170 | + }) | ||
171 | + } else if (typeof module === 'object' && module.exports) { | ||
172 | + module.exports = loadImage | ||
173 | + } else { | ||
174 | + $.loadImage = loadImage | ||
175 | + } | ||
176 | +})((typeof window !== 'undefined' && window) || this) |
node_modules/blueimp-load-image/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "blueimp-load-image", | ||
3 | + "version": "3.0.0", | ||
4 | + "title": "JavaScript Load Image", | ||
5 | + "description": "JavaScript Load Image is a library to load images provided as File or Blob objects or via URL. It returns an optionally scaled and/or cropped HTML img or canvas element. It also provides methods to parse image meta data to extract IPTC and Exif tags as well as embedded thumbnail images and to restore the complete image header after resizing.", | ||
6 | + "keywords": [ | ||
7 | + "javascript", | ||
8 | + "load", | ||
9 | + "loading", | ||
10 | + "image", | ||
11 | + "file", | ||
12 | + "blob", | ||
13 | + "url", | ||
14 | + "scale", | ||
15 | + "crop", | ||
16 | + "img", | ||
17 | + "canvas", | ||
18 | + "meta", | ||
19 | + "exif", | ||
20 | + "iptc", | ||
21 | + "thumbnail", | ||
22 | + "resizing" | ||
23 | + ], | ||
24 | + "homepage": "https://github.com/blueimp/JavaScript-Load-Image", | ||
25 | + "author": { | ||
26 | + "name": "Sebastian Tschan", | ||
27 | + "url": "https://blueimp.net" | ||
28 | + }, | ||
29 | + "repository": { | ||
30 | + "type": "git", | ||
31 | + "url": "git://github.com/blueimp/JavaScript-Load-Image.git" | ||
32 | + }, | ||
33 | + "license": "MIT", | ||
34 | + "devDependencies": { | ||
35 | + "eslint": "6", | ||
36 | + "eslint-config-blueimp": "1", | ||
37 | + "eslint-config-prettier": "6", | ||
38 | + "eslint-plugin-jsdoc": "22", | ||
39 | + "eslint-plugin-prettier": "3", | ||
40 | + "prettier": "2", | ||
41 | + "uglify-js": "3" | ||
42 | + }, | ||
43 | + "eslintConfig": { | ||
44 | + "extends": [ | ||
45 | + "blueimp", | ||
46 | + "plugin:jsdoc/recommended", | ||
47 | + "plugin:prettier/recommended" | ||
48 | + ], | ||
49 | + "env": { | ||
50 | + "browser": true | ||
51 | + } | ||
52 | + }, | ||
53 | + "eslintIgnore": [ | ||
54 | + "js/*.min.js", | ||
55 | + "js/vendor", | ||
56 | + "test/vendor" | ||
57 | + ], | ||
58 | + "prettier": { | ||
59 | + "arrowParens": "avoid", | ||
60 | + "proseWrap": "always", | ||
61 | + "semi": false, | ||
62 | + "singleQuote": true, | ||
63 | + "trailingComma": "none" | ||
64 | + }, | ||
65 | + "scripts": { | ||
66 | + "lint": "eslint .", | ||
67 | + "unit": "docker-compose run --rm mocha", | ||
68 | + "test": "npm run lint && npm run unit", | ||
69 | + "posttest": "docker-compose down -v", | ||
70 | + "build": "cd js && uglifyjs load-image.js load-image-scale.js load-image-meta.js load-image-fetch.js load-image-orientation.js load-image-exif.js load-image-exif-map.js load-image-iptc.js load-image-iptc-map.js -c -m -o load-image.all.min.js --source-map url=load-image.all.min.js.map", | ||
71 | + "preversion": "npm test", | ||
72 | + "version": "npm run build && git add -A js", | ||
73 | + "postversion": "git push --tags origin master master:gh-pages && npm publish" | ||
74 | + }, | ||
75 | + "files": [ | ||
76 | + "js/*.js", | ||
77 | + "js/*.js.map" | ||
78 | + ], | ||
79 | + "main": "js/index.js" | ||
80 | +} |
node_modules/bmp-js/.npmignore
0 → 100644
node_modules/bmp-js/LICENSE
0 → 100644
1 | +The MIT License (MIT) | ||
2 | + | ||
3 | +Copyright (c) 2014 @丝刀口 | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
6 | +of this software and associated documentation files (the "Software"), to deal | ||
7 | +in the Software without restriction, including without limitation the rights | ||
8 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
9 | +copies of the Software, and to permit persons to whom the Software is | ||
10 | +furnished to do so, subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in all | ||
13 | +copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
17 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
18 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
19 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
20 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
21 | +SOFTWARE. |
node_modules/bmp-js/README.md
0 → 100644
1 | +bmp-js | ||
2 | +====== | ||
3 | + | ||
4 | +A pure javascript Bmp encoder and decoder for node.js | ||
5 | + | ||
6 | +Supports all bits decoding(1,4,8,16,24,32) and encoding with 24bit. | ||
7 | + | ||
8 | +##Install | ||
9 | + | ||
10 | + $ npm install bmp-js | ||
11 | + | ||
12 | + | ||
13 | +How to use? | ||
14 | +--- | ||
15 | +###Decode BMP | ||
16 | +```js | ||
17 | +var bmp = require("bmp-js"); | ||
18 | +var bmpBuffer = fs.readFileSync('bit24.bmp'); | ||
19 | +var bmpData = bmp.decode(bmpBuffer); | ||
20 | + | ||
21 | +``` | ||
22 | + | ||
23 | +`bmpData` has all properties includes: | ||
24 | + | ||
25 | +1. fileSize,reserved,offset | ||
26 | + | ||
27 | +2. headerSize,width,height,planes,bitPP,compress,rawSize,hr,vr,colors,importantColors | ||
28 | + | ||
29 | +3. palette | ||
30 | + | ||
31 | +4. data-------byte array order by ABGR ABGR ABGR,4 bytes per pixel | ||
32 | + | ||
33 | + | ||
34 | +###Encode RGB | ||
35 | +```js | ||
36 | +var bmp = require("bmp-js"); | ||
37 | +//bmpData={data:Buffer,width:Number,height:Height} | ||
38 | +var rawData = bmp.encode(bmpData);//default no compression,write rawData to .bmp file | ||
39 | + | ||
40 | +``` | ||
41 | + | ||
42 | +License | ||
43 | +--- | ||
44 | +U can use on free with [MIT License](https://github.com/shaozilee/bmp-js/blob/master/LICENSE) | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/bmp-js/bmp-js.iml
0 → 100644
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<module type="WEB_MODULE" version="4"> | ||
3 | + <component name="NewModuleRootManager" inherit-compiler-output="true"> | ||
4 | + <exclude-output /> | ||
5 | + <content url="file://$MODULE_DIR$" /> | ||
6 | + <orderEntry type="inheritedJdk" /> | ||
7 | + <orderEntry type="sourceFolder" forTests="false" /> | ||
8 | + </component> | ||
9 | +</module> | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/bmp-js/index.js
0 → 100644
node_modules/bmp-js/lib/decoder.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/bmp-js/lib/encoder.js
0 → 100644
1 | +/** | ||
2 | + * @author shaozilee | ||
3 | + * | ||
4 | + * BMP format encoder,encode 24bit BMP | ||
5 | + * Not support quality compression | ||
6 | + * | ||
7 | + */ | ||
8 | + | ||
9 | +function BmpEncoder(imgData){ | ||
10 | + this.buffer = imgData.data; | ||
11 | + this.width = imgData.width; | ||
12 | + this.height = imgData.height; | ||
13 | + this.extraBytes = this.width%4; | ||
14 | + this.rgbSize = this.height*(3*this.width+this.extraBytes); | ||
15 | + this.headerInfoSize = 40; | ||
16 | + | ||
17 | + this.data = []; | ||
18 | + /******************header***********************/ | ||
19 | + this.flag = "BM"; | ||
20 | + this.reserved = 0; | ||
21 | + this.offset = 54; | ||
22 | + this.fileSize = this.rgbSize+this.offset; | ||
23 | + this.planes = 1; | ||
24 | + this.bitPP = 24; | ||
25 | + this.compress = 0; | ||
26 | + this.hr = 0; | ||
27 | + this.vr = 0; | ||
28 | + this.colors = 0; | ||
29 | + this.importantColors = 0; | ||
30 | +} | ||
31 | + | ||
32 | +BmpEncoder.prototype.encode = function() { | ||
33 | + var tempBuffer = new Buffer(this.offset+this.rgbSize); | ||
34 | + this.pos = 0; | ||
35 | + tempBuffer.write(this.flag,this.pos,2);this.pos+=2; | ||
36 | + tempBuffer.writeUInt32LE(this.fileSize,this.pos);this.pos+=4; | ||
37 | + tempBuffer.writeUInt32LE(this.reserved,this.pos);this.pos+=4; | ||
38 | + tempBuffer.writeUInt32LE(this.offset,this.pos);this.pos+=4; | ||
39 | + | ||
40 | + tempBuffer.writeUInt32LE(this.headerInfoSize,this.pos);this.pos+=4; | ||
41 | + tempBuffer.writeUInt32LE(this.width,this.pos);this.pos+=4; | ||
42 | + tempBuffer.writeInt32LE(-this.height,this.pos);this.pos+=4; | ||
43 | + tempBuffer.writeUInt16LE(this.planes,this.pos);this.pos+=2; | ||
44 | + tempBuffer.writeUInt16LE(this.bitPP,this.pos);this.pos+=2; | ||
45 | + tempBuffer.writeUInt32LE(this.compress,this.pos);this.pos+=4; | ||
46 | + tempBuffer.writeUInt32LE(this.rgbSize,this.pos);this.pos+=4; | ||
47 | + tempBuffer.writeUInt32LE(this.hr,this.pos);this.pos+=4; | ||
48 | + tempBuffer.writeUInt32LE(this.vr,this.pos);this.pos+=4; | ||
49 | + tempBuffer.writeUInt32LE(this.colors,this.pos);this.pos+=4; | ||
50 | + tempBuffer.writeUInt32LE(this.importantColors,this.pos);this.pos+=4; | ||
51 | + | ||
52 | + var i=0; | ||
53 | + var rowBytes = 3*this.width+this.extraBytes; | ||
54 | + | ||
55 | + for (var y = 0; y <this.height; y++){ | ||
56 | + for (var x = 0; x < this.width; x++){ | ||
57 | + var p = this.pos+y*rowBytes+x*3; | ||
58 | + i++;//a | ||
59 | + tempBuffer[p]= this.buffer[i++];//b | ||
60 | + tempBuffer[p+1] = this.buffer[i++];//g | ||
61 | + tempBuffer[p+2] = this.buffer[i++];//r | ||
62 | + } | ||
63 | + if(this.extraBytes>0){ | ||
64 | + var fillOffset = this.pos+y*rowBytes+this.width*3; | ||
65 | + tempBuffer.fill(0,fillOffset,fillOffset+this.extraBytes); | ||
66 | + } | ||
67 | + } | ||
68 | + | ||
69 | + return tempBuffer; | ||
70 | +}; | ||
71 | + | ||
72 | +module.exports = function(imgData, quality) { | ||
73 | + if (typeof quality === 'undefined') quality = 100; | ||
74 | + var encoder = new BmpEncoder(imgData); | ||
75 | + var data = encoder.encode(); | ||
76 | + return { | ||
77 | + data: data, | ||
78 | + width: imgData.width, | ||
79 | + height: imgData.height | ||
80 | + }; | ||
81 | +}; |
node_modules/bmp-js/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "bmp-js", | ||
3 | + "version": "0.1.0", | ||
4 | + "description": "A pure javascript BMP encoder and decoder", | ||
5 | + "main": "index.js", | ||
6 | + "repository": { | ||
7 | + "type": "git", | ||
8 | + "url": "https://github.com/shaozilee/bmp-js" | ||
9 | + }, | ||
10 | + "keywords": [ | ||
11 | + "bmp", | ||
12 | + "1bit", | ||
13 | + "4bit", | ||
14 | + "8bit", | ||
15 | + "16bit", | ||
16 | + "24bit", | ||
17 | + "32bit", | ||
18 | + "encoder", | ||
19 | + "decoder", | ||
20 | + "image", | ||
21 | + "javascript", | ||
22 | + "js" | ||
23 | + ], | ||
24 | + "author": { | ||
25 | + "name": "shaozilee", | ||
26 | + "email": "shaozilee@gmail.com" | ||
27 | + }, | ||
28 | + "license": "MIT", | ||
29 | + "dependencies": {}, | ||
30 | + "devDependencies": { | ||
31 | + } | ||
32 | +} |
node_modules/bmp-js/test/bit1.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_565.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_565_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_a444.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_a444_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_a555.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_a555_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_x444.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_x444_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_x555.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit16_x555_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit1_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit24.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit24_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit32.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit32_alpha.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit32_alpha_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit32_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit4.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit4_RLE.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit4_RLE_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit4_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit8.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit8_RLE.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit8_RLE_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/bit8_out.bmp
0 → 100644
No preview for this file type
node_modules/bmp-js/test/test.js
0 → 100644
1 | +var fs = require("fs"); | ||
2 | + | ||
3 | +var coder = require("../index.js"); | ||
4 | +var bmps = ["./bit1", "./bit4", "./bit4_RLE", "./bit8", "./bit8_RLE", "./bit16_565", "./bit16_a444", "./bit16_a555", "./bit16_x444", "./bit16_x555", "./bit24", "./bit32", "./bit32_alpha"]; | ||
5 | + | ||
6 | +console.log("test bmp decoding and encoding..."); | ||
7 | + | ||
8 | +for(var b=0; b<bmps.length;b++){ | ||
9 | + var src =bmps[b]; | ||
10 | + console.log("----------------"+src+".bmp"); | ||
11 | + var bufferData = fs.readFileSync(src+".bmp"); | ||
12 | + var decoder = coder.decode(bufferData); | ||
13 | + console.log("width:",decoder.width); | ||
14 | + console.log("height",decoder.height); | ||
15 | + console.log("fileSize:",decoder.fileSize); | ||
16 | + | ||
17 | + | ||
18 | + //encode with 24bit | ||
19 | + var encodeData = coder.encode(decoder); | ||
20 | + fs.writeFileSync(src+"_out.bmp", encodeData.data); | ||
21 | +} | ||
22 | + | ||
23 | +console.log("test bmp success!"); | ||
24 | + | ||
25 | + | ||
26 | + | ||
27 | + | ||
28 | + | ||
29 | + | ||
30 | + | ||
31 | + | ||
32 | + | ||
33 | + |
node_modules/brace-expansion/LICENSE
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
6 | +of this software and associated documentation files (the "Software"), to deal | ||
7 | +in the Software without restriction, including without limitation the rights | ||
8 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
9 | +copies of the Software, and to permit persons to whom the Software is | ||
10 | +furnished to do so, subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in all | ||
13 | +copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
17 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
18 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
19 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
20 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
21 | +SOFTWARE. |
node_modules/brace-expansion/README.md
0 → 100644
1 | +# brace-expansion | ||
2 | + | ||
3 | +[Brace expansion](https://www.gnu.org/software/bash/manual/html_node/Brace-Expansion.html), | ||
4 | +as known from sh/bash, in JavaScript. | ||
5 | + | ||
6 | +[](http://travis-ci.org/juliangruber/brace-expansion) | ||
7 | +[](https://www.npmjs.org/package/brace-expansion) | ||
8 | +[](https://greenkeeper.io/) | ||
9 | + | ||
10 | +[](https://ci.testling.com/juliangruber/brace-expansion) | ||
11 | + | ||
12 | +## Example | ||
13 | + | ||
14 | +```js | ||
15 | +var expand = require('brace-expansion'); | ||
16 | + | ||
17 | +expand('file-{a,b,c}.jpg') | ||
18 | +// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg'] | ||
19 | + | ||
20 | +expand('-v{,,}') | ||
21 | +// => ['-v', '-v', '-v'] | ||
22 | + | ||
23 | +expand('file{0..2}.jpg') | ||
24 | +// => ['file0.jpg', 'file1.jpg', 'file2.jpg'] | ||
25 | + | ||
26 | +expand('file-{a..c}.jpg') | ||
27 | +// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg'] | ||
28 | + | ||
29 | +expand('file{2..0}.jpg') | ||
30 | +// => ['file2.jpg', 'file1.jpg', 'file0.jpg'] | ||
31 | + | ||
32 | +expand('file{0..4..2}.jpg') | ||
33 | +// => ['file0.jpg', 'file2.jpg', 'file4.jpg'] | ||
34 | + | ||
35 | +expand('file-{a..e..2}.jpg') | ||
36 | +// => ['file-a.jpg', 'file-c.jpg', 'file-e.jpg'] | ||
37 | + | ||
38 | +expand('file{00..10..5}.jpg') | ||
39 | +// => ['file00.jpg', 'file05.jpg', 'file10.jpg'] | ||
40 | + | ||
41 | +expand('{{A..C},{a..c}}') | ||
42 | +// => ['A', 'B', 'C', 'a', 'b', 'c'] | ||
43 | + | ||
44 | +expand('ppp{,config,oe{,conf}}') | ||
45 | +// => ['ppp', 'pppconfig', 'pppoe', 'pppoeconf'] | ||
46 | +``` | ||
47 | + | ||
48 | +## API | ||
49 | + | ||
50 | +```js | ||
51 | +var expand = require('brace-expansion'); | ||
52 | +``` | ||
53 | + | ||
54 | +### var expanded = expand(str) | ||
55 | + | ||
56 | +Return an array of all possible and valid expansions of `str`. If none are | ||
57 | +found, `[str]` is returned. | ||
58 | + | ||
59 | +Valid expansions are: | ||
60 | + | ||
61 | +```js | ||
62 | +/^(.*,)+(.+)?$/ | ||
63 | +// {a,b,...} | ||
64 | +``` | ||
65 | + | ||
66 | +A comma separated list of options, like `{a,b}` or `{a,{b,c}}` or `{,a,}`. | ||
67 | + | ||
68 | +```js | ||
69 | +/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/ | ||
70 | +// {x..y[..incr]} | ||
71 | +``` | ||
72 | + | ||
73 | +A numeric sequence from `x` to `y` inclusive, with optional increment. | ||
74 | +If `x` or `y` start with a leading `0`, all the numbers will be padded | ||
75 | +to have equal length. Negative numbers and backwards iteration work too. | ||
76 | + | ||
77 | +```js | ||
78 | +/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/ | ||
79 | +// {x..y[..incr]} | ||
80 | +``` | ||
81 | + | ||
82 | +An alphabetic sequence from `x` to `y` inclusive, with optional increment. | ||
83 | +`x` and `y` must be exactly one character, and if given, `incr` must be a | ||
84 | +number. | ||
85 | + | ||
86 | +For compatibility reasons, the string `${` is not eligible for brace expansion. | ||
87 | + | ||
88 | +## Installation | ||
89 | + | ||
90 | +With [npm](https://npmjs.org) do: | ||
91 | + | ||
92 | +```bash | ||
93 | +npm install brace-expansion | ||
94 | +``` | ||
95 | + | ||
96 | +## Contributors | ||
97 | + | ||
98 | +- [Julian Gruber](https://github.com/juliangruber) | ||
99 | +- [Isaac Z. Schlueter](https://github.com/isaacs) | ||
100 | + | ||
101 | +## Sponsors | ||
102 | + | ||
103 | +This module is proudly supported by my [Sponsors](https://github.com/juliangruber/sponsors)! | ||
104 | + | ||
105 | +Do you want to support modules like this to improve their quality, stability and weigh in on new features? Then please consider donating to my [Patreon](https://www.patreon.com/juliangruber). Not sure how much of my modules you're using? Try [feross/thanks](https://github.com/feross/thanks)! | ||
106 | + | ||
107 | +## License | ||
108 | + | ||
109 | +(MIT) | ||
110 | + | ||
111 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
112 | + | ||
113 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
114 | +this software and associated documentation files (the "Software"), to deal in | ||
115 | +the Software without restriction, including without limitation the rights to | ||
116 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
117 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
118 | +so, subject to the following conditions: | ||
119 | + | ||
120 | +The above copyright notice and this permission notice shall be included in all | ||
121 | +copies or substantial portions of the Software. | ||
122 | + | ||
123 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
124 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
125 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
126 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
127 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
128 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
129 | +SOFTWARE. |
node_modules/brace-expansion/index.js
0 → 100644
1 | +var concatMap = require('concat-map'); | ||
2 | +var balanced = require('balanced-match'); | ||
3 | + | ||
4 | +module.exports = expandTop; | ||
5 | + | ||
6 | +var escSlash = '\0SLASH'+Math.random()+'\0'; | ||
7 | +var escOpen = '\0OPEN'+Math.random()+'\0'; | ||
8 | +var escClose = '\0CLOSE'+Math.random()+'\0'; | ||
9 | +var escComma = '\0COMMA'+Math.random()+'\0'; | ||
10 | +var escPeriod = '\0PERIOD'+Math.random()+'\0'; | ||
11 | + | ||
12 | +function numeric(str) { | ||
13 | + return parseInt(str, 10) == str | ||
14 | + ? parseInt(str, 10) | ||
15 | + : str.charCodeAt(0); | ||
16 | +} | ||
17 | + | ||
18 | +function escapeBraces(str) { | ||
19 | + return str.split('\\\\').join(escSlash) | ||
20 | + .split('\\{').join(escOpen) | ||
21 | + .split('\\}').join(escClose) | ||
22 | + .split('\\,').join(escComma) | ||
23 | + .split('\\.').join(escPeriod); | ||
24 | +} | ||
25 | + | ||
26 | +function unescapeBraces(str) { | ||
27 | + return str.split(escSlash).join('\\') | ||
28 | + .split(escOpen).join('{') | ||
29 | + .split(escClose).join('}') | ||
30 | + .split(escComma).join(',') | ||
31 | + .split(escPeriod).join('.'); | ||
32 | +} | ||
33 | + | ||
34 | + | ||
35 | +// Basically just str.split(","), but handling cases | ||
36 | +// where we have nested braced sections, which should be | ||
37 | +// treated as individual members, like {a,{b,c},d} | ||
38 | +function parseCommaParts(str) { | ||
39 | + if (!str) | ||
40 | + return ['']; | ||
41 | + | ||
42 | + var parts = []; | ||
43 | + var m = balanced('{', '}', str); | ||
44 | + | ||
45 | + if (!m) | ||
46 | + return str.split(','); | ||
47 | + | ||
48 | + var pre = m.pre; | ||
49 | + var body = m.body; | ||
50 | + var post = m.post; | ||
51 | + var p = pre.split(','); | ||
52 | + | ||
53 | + p[p.length-1] += '{' + body + '}'; | ||
54 | + var postParts = parseCommaParts(post); | ||
55 | + if (post.length) { | ||
56 | + p[p.length-1] += postParts.shift(); | ||
57 | + p.push.apply(p, postParts); | ||
58 | + } | ||
59 | + | ||
60 | + parts.push.apply(parts, p); | ||
61 | + | ||
62 | + return parts; | ||
63 | +} | ||
64 | + | ||
65 | +function expandTop(str) { | ||
66 | + if (!str) | ||
67 | + return []; | ||
68 | + | ||
69 | + // I don't know why Bash 4.3 does this, but it does. | ||
70 | + // Anything starting with {} will have the first two bytes preserved | ||
71 | + // but *only* at the top level, so {},a}b will not expand to anything, | ||
72 | + // but a{},b}c will be expanded to [a}c,abc]. | ||
73 | + // One could argue that this is a bug in Bash, but since the goal of | ||
74 | + // this module is to match Bash's rules, we escape a leading {} | ||
75 | + if (str.substr(0, 2) === '{}') { | ||
76 | + str = '\\{\\}' + str.substr(2); | ||
77 | + } | ||
78 | + | ||
79 | + return expand(escapeBraces(str), true).map(unescapeBraces); | ||
80 | +} | ||
81 | + | ||
82 | +function identity(e) { | ||
83 | + return e; | ||
84 | +} | ||
85 | + | ||
86 | +function embrace(str) { | ||
87 | + return '{' + str + '}'; | ||
88 | +} | ||
89 | +function isPadded(el) { | ||
90 | + return /^-?0\d/.test(el); | ||
91 | +} | ||
92 | + | ||
93 | +function lte(i, y) { | ||
94 | + return i <= y; | ||
95 | +} | ||
96 | +function gte(i, y) { | ||
97 | + return i >= y; | ||
98 | +} | ||
99 | + | ||
100 | +function expand(str, isTop) { | ||
101 | + var expansions = []; | ||
102 | + | ||
103 | + var m = balanced('{', '}', str); | ||
104 | + if (!m || /\$$/.test(m.pre)) return [str]; | ||
105 | + | ||
106 | + var isNumericSequence = /^-?\d+\.\.-?\d+(?:\.\.-?\d+)?$/.test(m.body); | ||
107 | + var isAlphaSequence = /^[a-zA-Z]\.\.[a-zA-Z](?:\.\.-?\d+)?$/.test(m.body); | ||
108 | + var isSequence = isNumericSequence || isAlphaSequence; | ||
109 | + var isOptions = m.body.indexOf(',') >= 0; | ||
110 | + if (!isSequence && !isOptions) { | ||
111 | + // {a},b} | ||
112 | + if (m.post.match(/,.*\}/)) { | ||
113 | + str = m.pre + '{' + m.body + escClose + m.post; | ||
114 | + return expand(str); | ||
115 | + } | ||
116 | + return [str]; | ||
117 | + } | ||
118 | + | ||
119 | + var n; | ||
120 | + if (isSequence) { | ||
121 | + n = m.body.split(/\.\./); | ||
122 | + } else { | ||
123 | + n = parseCommaParts(m.body); | ||
124 | + if (n.length === 1) { | ||
125 | + // x{{a,b}}y ==> x{a}y x{b}y | ||
126 | + n = expand(n[0], false).map(embrace); | ||
127 | + if (n.length === 1) { | ||
128 | + var post = m.post.length | ||
129 | + ? expand(m.post, false) | ||
130 | + : ['']; | ||
131 | + return post.map(function(p) { | ||
132 | + return m.pre + n[0] + p; | ||
133 | + }); | ||
134 | + } | ||
135 | + } | ||
136 | + } | ||
137 | + | ||
138 | + // at this point, n is the parts, and we know it's not a comma set | ||
139 | + // with a single entry. | ||
140 | + | ||
141 | + // no need to expand pre, since it is guaranteed to be free of brace-sets | ||
142 | + var pre = m.pre; | ||
143 | + var post = m.post.length | ||
144 | + ? expand(m.post, false) | ||
145 | + : ['']; | ||
146 | + | ||
147 | + var N; | ||
148 | + | ||
149 | + if (isSequence) { | ||
150 | + var x = numeric(n[0]); | ||
151 | + var y = numeric(n[1]); | ||
152 | + var width = Math.max(n[0].length, n[1].length) | ||
153 | + var incr = n.length == 3 | ||
154 | + ? Math.abs(numeric(n[2])) | ||
155 | + : 1; | ||
156 | + var test = lte; | ||
157 | + var reverse = y < x; | ||
158 | + if (reverse) { | ||
159 | + incr *= -1; | ||
160 | + test = gte; | ||
161 | + } | ||
162 | + var pad = n.some(isPadded); | ||
163 | + | ||
164 | + N = []; | ||
165 | + | ||
166 | + for (var i = x; test(i, y); i += incr) { | ||
167 | + var c; | ||
168 | + if (isAlphaSequence) { | ||
169 | + c = String.fromCharCode(i); | ||
170 | + if (c === '\\') | ||
171 | + c = ''; | ||
172 | + } else { | ||
173 | + c = String(i); | ||
174 | + if (pad) { | ||
175 | + var need = width - c.length; | ||
176 | + if (need > 0) { | ||
177 | + var z = new Array(need + 1).join('0'); | ||
178 | + if (i < 0) | ||
179 | + c = '-' + z + c.slice(1); | ||
180 | + else | ||
181 | + c = z + c; | ||
182 | + } | ||
183 | + } | ||
184 | + } | ||
185 | + N.push(c); | ||
186 | + } | ||
187 | + } else { | ||
188 | + N = concatMap(n, function(el) { return expand(el, false) }); | ||
189 | + } | ||
190 | + | ||
191 | + for (var j = 0; j < N.length; j++) { | ||
192 | + for (var k = 0; k < post.length; k++) { | ||
193 | + var expansion = pre + N[j] + post[k]; | ||
194 | + if (!isTop || isSequence || expansion) | ||
195 | + expansions.push(expansion); | ||
196 | + } | ||
197 | + } | ||
198 | + | ||
199 | + return expansions; | ||
200 | +} | ||
201 | + |
node_modules/brace-expansion/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "brace-expansion", | ||
3 | + "description": "Brace expansion as known from sh/bash", | ||
4 | + "version": "1.1.11", | ||
5 | + "repository": { | ||
6 | + "type": "git", | ||
7 | + "url": "git://github.com/juliangruber/brace-expansion.git" | ||
8 | + }, | ||
9 | + "homepage": "https://github.com/juliangruber/brace-expansion", | ||
10 | + "main": "index.js", | ||
11 | + "scripts": { | ||
12 | + "test": "tape test/*.js", | ||
13 | + "gentest": "bash test/generate.sh", | ||
14 | + "bench": "matcha test/perf/bench.js" | ||
15 | + }, | ||
16 | + "dependencies": { | ||
17 | + "balanced-match": "^1.0.0", | ||
18 | + "concat-map": "0.0.1" | ||
19 | + }, | ||
20 | + "devDependencies": { | ||
21 | + "matcha": "^0.7.0", | ||
22 | + "tape": "^4.6.0" | ||
23 | + }, | ||
24 | + "keywords": [], | ||
25 | + "author": { | ||
26 | + "name": "Julian Gruber", | ||
27 | + "email": "mail@juliangruber.com", | ||
28 | + "url": "http://juliangruber.com" | ||
29 | + }, | ||
30 | + "license": "MIT", | ||
31 | + "testling": { | ||
32 | + "files": "test/*.js", | ||
33 | + "browsers": [ | ||
34 | + "ie/8..latest", | ||
35 | + "firefox/20..latest", | ||
36 | + "firefox/nightly", | ||
37 | + "chrome/25..latest", | ||
38 | + "chrome/canary", | ||
39 | + "opera/12..latest", | ||
40 | + "opera/next", | ||
41 | + "safari/5.1..latest", | ||
42 | + "ipad/6.0..latest", | ||
43 | + "iphone/6.0..latest", | ||
44 | + "android-browser/4.2..latest" | ||
45 | + ] | ||
46 | + } | ||
47 | +} |
node_modules/colors/LICENSE
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Original Library | ||
4 | + - Copyright (c) Marak Squires | ||
5 | + | ||
6 | +Additional Functionality | ||
7 | + - Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
8 | + | ||
9 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
10 | +of this software and associated documentation files (the "Software"), to deal | ||
11 | +in the Software without restriction, including without limitation the rights | ||
12 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
13 | +copies of the Software, and to permit persons to whom the Software is | ||
14 | +furnished to do so, subject to the following conditions: | ||
15 | + | ||
16 | +The above copyright notice and this permission notice shall be included in | ||
17 | +all copies or substantial portions of the Software. | ||
18 | + | ||
19 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
20 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
21 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
22 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
23 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
24 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
25 | +THE SOFTWARE. |
node_modules/colors/README.md
0 → 100644
1 | +# colors.js | ||
2 | +[](https://travis-ci.org/Marak/colors.js) | ||
3 | +[](https://www.npmjs.org/package/colors) | ||
4 | +[](https://david-dm.org/Marak/colors.js) | ||
5 | +[](https://david-dm.org/Marak/colors.js#info=devDependencies) | ||
6 | + | ||
7 | +Please check out the [roadmap](ROADMAP.md) for upcoming features and releases. Please open Issues to provide feedback, and check the `develop` branch for the latest bleeding-edge updates. | ||
8 | + | ||
9 | +## get color and style in your node.js console | ||
10 | + | ||
11 | +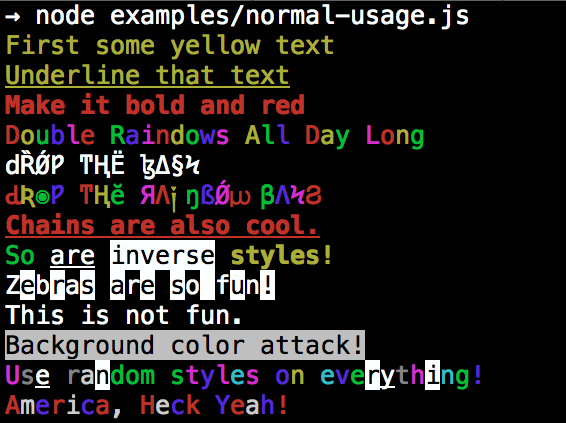 | ||
12 | + | ||
13 | +## Installation | ||
14 | + | ||
15 | + npm install colors | ||
16 | + | ||
17 | +## colors and styles! | ||
18 | + | ||
19 | +### text colors | ||
20 | + | ||
21 | + - black | ||
22 | + - red | ||
23 | + - green | ||
24 | + - yellow | ||
25 | + - blue | ||
26 | + - magenta | ||
27 | + - cyan | ||
28 | + - white | ||
29 | + - gray | ||
30 | + - grey | ||
31 | + | ||
32 | +### bright text colors | ||
33 | + | ||
34 | + - brightRed | ||
35 | + - brightGreen | ||
36 | + - brightYellow | ||
37 | + - brightBlue | ||
38 | + - brightMagenta | ||
39 | + - brightCyan | ||
40 | + - brightWhite | ||
41 | + | ||
42 | +### background colors | ||
43 | + | ||
44 | + - bgBlack | ||
45 | + - bgRed | ||
46 | + - bgGreen | ||
47 | + - bgYellow | ||
48 | + - bgBlue | ||
49 | + - bgMagenta | ||
50 | + - bgCyan | ||
51 | + - bgWhite | ||
52 | + - bgGray | ||
53 | + - bgGrey | ||
54 | + | ||
55 | +### bright background colors | ||
56 | + | ||
57 | + - bgBrightRed | ||
58 | + - bgBrightGreen | ||
59 | + - bgBrightYellow | ||
60 | + - bgBrightBlue | ||
61 | + - bgBrightMagenta | ||
62 | + - bgBrightCyan | ||
63 | + - bgBrightWhite | ||
64 | + | ||
65 | +### styles | ||
66 | + | ||
67 | + - reset | ||
68 | + - bold | ||
69 | + - dim | ||
70 | + - italic | ||
71 | + - underline | ||
72 | + - inverse | ||
73 | + - hidden | ||
74 | + - strikethrough | ||
75 | + | ||
76 | +### extras | ||
77 | + | ||
78 | + - rainbow | ||
79 | + - zebra | ||
80 | + - america | ||
81 | + - trap | ||
82 | + - random | ||
83 | + | ||
84 | + | ||
85 | +## Usage | ||
86 | + | ||
87 | +By popular demand, `colors` now ships with two types of usages! | ||
88 | + | ||
89 | +The super nifty way | ||
90 | + | ||
91 | +```js | ||
92 | +var colors = require('colors'); | ||
93 | + | ||
94 | +console.log('hello'.green); // outputs green text | ||
95 | +console.log('i like cake and pies'.underline.red) // outputs red underlined text | ||
96 | +console.log('inverse the color'.inverse); // inverses the color | ||
97 | +console.log('OMG Rainbows!'.rainbow); // rainbow | ||
98 | +console.log('Run the trap'.trap); // Drops the bass | ||
99 | + | ||
100 | +``` | ||
101 | + | ||
102 | +or a slightly less nifty way which doesn't extend `String.prototype` | ||
103 | + | ||
104 | +```js | ||
105 | +var colors = require('colors/safe'); | ||
106 | + | ||
107 | +console.log(colors.green('hello')); // outputs green text | ||
108 | +console.log(colors.red.underline('i like cake and pies')) // outputs red underlined text | ||
109 | +console.log(colors.inverse('inverse the color')); // inverses the color | ||
110 | +console.log(colors.rainbow('OMG Rainbows!')); // rainbow | ||
111 | +console.log(colors.trap('Run the trap')); // Drops the bass | ||
112 | + | ||
113 | +``` | ||
114 | + | ||
115 | +I prefer the first way. Some people seem to be afraid of extending `String.prototype` and prefer the second way. | ||
116 | + | ||
117 | +If you are writing good code you will never have an issue with the first approach. If you really don't want to touch `String.prototype`, the second usage will not touch `String` native object. | ||
118 | + | ||
119 | +## Enabling/Disabling Colors | ||
120 | + | ||
121 | +The package will auto-detect whether your terminal can use colors and enable/disable accordingly. When colors are disabled, the color functions do nothing. You can override this with a command-line flag: | ||
122 | + | ||
123 | +```bash | ||
124 | +node myapp.js --no-color | ||
125 | +node myapp.js --color=false | ||
126 | + | ||
127 | +node myapp.js --color | ||
128 | +node myapp.js --color=true | ||
129 | +node myapp.js --color=always | ||
130 | + | ||
131 | +FORCE_COLOR=1 node myapp.js | ||
132 | +``` | ||
133 | + | ||
134 | +Or in code: | ||
135 | + | ||
136 | +```javascript | ||
137 | +var colors = require('colors'); | ||
138 | +colors.enable(); | ||
139 | +colors.disable(); | ||
140 | +``` | ||
141 | + | ||
142 | +## Console.log [string substitution](http://nodejs.org/docs/latest/api/console.html#console_console_log_data) | ||
143 | + | ||
144 | +```js | ||
145 | +var name = 'Marak'; | ||
146 | +console.log(colors.green('Hello %s'), name); | ||
147 | +// outputs -> 'Hello Marak' | ||
148 | +``` | ||
149 | + | ||
150 | +## Custom themes | ||
151 | + | ||
152 | +### Using standard API | ||
153 | + | ||
154 | +```js | ||
155 | + | ||
156 | +var colors = require('colors'); | ||
157 | + | ||
158 | +colors.setTheme({ | ||
159 | + silly: 'rainbow', | ||
160 | + input: 'grey', | ||
161 | + verbose: 'cyan', | ||
162 | + prompt: 'grey', | ||
163 | + info: 'green', | ||
164 | + data: 'grey', | ||
165 | + help: 'cyan', | ||
166 | + warn: 'yellow', | ||
167 | + debug: 'blue', | ||
168 | + error: 'red' | ||
169 | +}); | ||
170 | + | ||
171 | +// outputs red text | ||
172 | +console.log("this is an error".error); | ||
173 | + | ||
174 | +// outputs yellow text | ||
175 | +console.log("this is a warning".warn); | ||
176 | +``` | ||
177 | + | ||
178 | +### Using string safe API | ||
179 | + | ||
180 | +```js | ||
181 | +var colors = require('colors/safe'); | ||
182 | + | ||
183 | +// set single property | ||
184 | +var error = colors.red; | ||
185 | +error('this is red'); | ||
186 | + | ||
187 | +// set theme | ||
188 | +colors.setTheme({ | ||
189 | + silly: 'rainbow', | ||
190 | + input: 'grey', | ||
191 | + verbose: 'cyan', | ||
192 | + prompt: 'grey', | ||
193 | + info: 'green', | ||
194 | + data: 'grey', | ||
195 | + help: 'cyan', | ||
196 | + warn: 'yellow', | ||
197 | + debug: 'blue', | ||
198 | + error: 'red' | ||
199 | +}); | ||
200 | + | ||
201 | +// outputs red text | ||
202 | +console.log(colors.error("this is an error")); | ||
203 | + | ||
204 | +// outputs yellow text | ||
205 | +console.log(colors.warn("this is a warning")); | ||
206 | + | ||
207 | +``` | ||
208 | + | ||
209 | +### Combining Colors | ||
210 | + | ||
211 | +```javascript | ||
212 | +var colors = require('colors'); | ||
213 | + | ||
214 | +colors.setTheme({ | ||
215 | + custom: ['red', 'underline'] | ||
216 | +}); | ||
217 | + | ||
218 | +console.log('test'.custom); | ||
219 | +``` | ||
220 | + | ||
221 | +*Protip: There is a secret undocumented style in `colors`. If you find the style you can summon him.* |
node_modules/colors/examples/normal-usage.js
0 → 100644
1 | +var colors = require('../lib/index'); | ||
2 | + | ||
3 | +console.log('First some yellow text'.yellow); | ||
4 | + | ||
5 | +console.log('Underline that text'.yellow.underline); | ||
6 | + | ||
7 | +console.log('Make it bold and red'.red.bold); | ||
8 | + | ||
9 | +console.log(('Double Raindows All Day Long').rainbow); | ||
10 | + | ||
11 | +console.log('Drop the bass'.trap); | ||
12 | + | ||
13 | +console.log('DROP THE RAINBOW BASS'.trap.rainbow); | ||
14 | + | ||
15 | +// styles not widely supported | ||
16 | +console.log('Chains are also cool.'.bold.italic.underline.red); | ||
17 | + | ||
18 | +// styles not widely supported | ||
19 | +console.log('So '.green + 'are'.underline + ' ' + 'inverse'.inverse | ||
20 | + + ' styles! '.yellow.bold); | ||
21 | +console.log('Zebras are so fun!'.zebra); | ||
22 | + | ||
23 | +// | ||
24 | +// Remark: .strikethrough may not work with Mac OS Terminal App | ||
25 | +// | ||
26 | +console.log('This is ' + 'not'.strikethrough + ' fun.'); | ||
27 | + | ||
28 | +console.log('Background color attack!'.black.bgWhite); | ||
29 | +console.log('Use random styles on everything!'.random); | ||
30 | +console.log('America, Heck Yeah!'.america); | ||
31 | + | ||
32 | +console.log('Blindingly '.brightCyan + 'bright? '.brightRed + 'Why '.brightYellow + 'not?!'.brightGreen); | ||
33 | + | ||
34 | +console.log('Setting themes is useful'); | ||
35 | + | ||
36 | +// | ||
37 | +// Custom themes | ||
38 | +// | ||
39 | +console.log('Generic logging theme as JSON'.green.bold.underline); | ||
40 | +// Load theme with JSON literal | ||
41 | +colors.setTheme({ | ||
42 | + silly: 'rainbow', | ||
43 | + input: 'grey', | ||
44 | + verbose: 'cyan', | ||
45 | + prompt: 'grey', | ||
46 | + info: 'green', | ||
47 | + data: 'grey', | ||
48 | + help: 'cyan', | ||
49 | + warn: 'yellow', | ||
50 | + debug: 'blue', | ||
51 | + error: 'red', | ||
52 | +}); | ||
53 | + | ||
54 | +// outputs red text | ||
55 | +console.log('this is an error'.error); | ||
56 | + | ||
57 | +// outputs yellow text | ||
58 | +console.log('this is a warning'.warn); | ||
59 | + | ||
60 | +// outputs grey text | ||
61 | +console.log('this is an input'.input); | ||
62 | + | ||
63 | +console.log('Generic logging theme as file'.green.bold.underline); | ||
64 | + | ||
65 | +// Load a theme from file | ||
66 | +try { | ||
67 | + colors.setTheme(require(__dirname + '/../themes/generic-logging.js')); | ||
68 | +} catch (err) { | ||
69 | + console.log(err); | ||
70 | +} | ||
71 | + | ||
72 | +// outputs red text | ||
73 | +console.log('this is an error'.error); | ||
74 | + | ||
75 | +// outputs yellow text | ||
76 | +console.log('this is a warning'.warn); | ||
77 | + | ||
78 | +// outputs grey text | ||
79 | +console.log('this is an input'.input); | ||
80 | + | ||
81 | +// console.log("Don't summon".zalgo) | ||
82 | + |
node_modules/colors/examples/safe-string.js
0 → 100644
1 | +var colors = require('../safe'); | ||
2 | + | ||
3 | +console.log(colors.yellow('First some yellow text')); | ||
4 | + | ||
5 | +console.log(colors.yellow.underline('Underline that text')); | ||
6 | + | ||
7 | +console.log(colors.red.bold('Make it bold and red')); | ||
8 | + | ||
9 | +console.log(colors.rainbow('Double Raindows All Day Long')); | ||
10 | + | ||
11 | +console.log(colors.trap('Drop the bass')); | ||
12 | + | ||
13 | +console.log(colors.rainbow(colors.trap('DROP THE RAINBOW BASS'))); | ||
14 | + | ||
15 | +// styles not widely supported | ||
16 | +console.log(colors.bold.italic.underline.red('Chains are also cool.')); | ||
17 | + | ||
18 | +// styles not widely supported | ||
19 | +console.log(colors.green('So ') + colors.underline('are') + ' ' | ||
20 | + + colors.inverse('inverse') + colors.yellow.bold(' styles! ')); | ||
21 | + | ||
22 | +console.log(colors.zebra('Zebras are so fun!')); | ||
23 | + | ||
24 | +console.log('This is ' + colors.strikethrough('not') + ' fun.'); | ||
25 | + | ||
26 | + | ||
27 | +console.log(colors.black.bgWhite('Background color attack!')); | ||
28 | +console.log(colors.random('Use random styles on everything!')); | ||
29 | +console.log(colors.america('America, Heck Yeah!')); | ||
30 | + | ||
31 | +console.log(colors.brightCyan('Blindingly ') + colors.brightRed('bright? ') + colors.brightYellow('Why ') + colors.brightGreen('not?!')); | ||
32 | + | ||
33 | +console.log('Setting themes is useful'); | ||
34 | + | ||
35 | +// | ||
36 | +// Custom themes | ||
37 | +// | ||
38 | +// console.log('Generic logging theme as JSON'.green.bold.underline); | ||
39 | +// Load theme with JSON literal | ||
40 | +colors.setTheme({ | ||
41 | + silly: 'rainbow', | ||
42 | + input: 'blue', | ||
43 | + verbose: 'cyan', | ||
44 | + prompt: 'grey', | ||
45 | + info: 'green', | ||
46 | + data: 'grey', | ||
47 | + help: 'cyan', | ||
48 | + warn: 'yellow', | ||
49 | + debug: 'blue', | ||
50 | + error: 'red', | ||
51 | +}); | ||
52 | + | ||
53 | +// outputs red text | ||
54 | +console.log(colors.error('this is an error')); | ||
55 | + | ||
56 | +// outputs yellow text | ||
57 | +console.log(colors.warn('this is a warning')); | ||
58 | + | ||
59 | +// outputs blue text | ||
60 | +console.log(colors.input('this is an input')); | ||
61 | + | ||
62 | + | ||
63 | +// console.log('Generic logging theme as file'.green.bold.underline); | ||
64 | + | ||
65 | +// Load a theme from file | ||
66 | +colors.setTheme(require(__dirname + '/../themes/generic-logging.js')); | ||
67 | + | ||
68 | +// outputs red text | ||
69 | +console.log(colors.error('this is an error')); | ||
70 | + | ||
71 | +// outputs yellow text | ||
72 | +console.log(colors.warn('this is a warning')); | ||
73 | + | ||
74 | +// outputs grey text | ||
75 | +console.log(colors.input('this is an input')); | ||
76 | + | ||
77 | +// console.log(colors.zalgo("Don't summon him")) | ||
78 | + | ||
79 | + |
node_modules/colors/index.d.ts
0 → 100644
1 | +// Type definitions for Colors.js 1.2 | ||
2 | +// Project: https://github.com/Marak/colors.js | ||
3 | +// Definitions by: Bart van der Schoor <https://github.com/Bartvds>, Staffan Eketorp <https://github.com/staeke> | ||
4 | +// Definitions: https://github.com/Marak/colors.js | ||
5 | + | ||
6 | +export interface Color { | ||
7 | + (text: string): string; | ||
8 | + | ||
9 | + strip: Color; | ||
10 | + stripColors: Color; | ||
11 | + | ||
12 | + black: Color; | ||
13 | + red: Color; | ||
14 | + green: Color; | ||
15 | + yellow: Color; | ||
16 | + blue: Color; | ||
17 | + magenta: Color; | ||
18 | + cyan: Color; | ||
19 | + white: Color; | ||
20 | + gray: Color; | ||
21 | + grey: Color; | ||
22 | + | ||
23 | + bgBlack: Color; | ||
24 | + bgRed: Color; | ||
25 | + bgGreen: Color; | ||
26 | + bgYellow: Color; | ||
27 | + bgBlue: Color; | ||
28 | + bgMagenta: Color; | ||
29 | + bgCyan: Color; | ||
30 | + bgWhite: Color; | ||
31 | + | ||
32 | + reset: Color; | ||
33 | + bold: Color; | ||
34 | + dim: Color; | ||
35 | + italic: Color; | ||
36 | + underline: Color; | ||
37 | + inverse: Color; | ||
38 | + hidden: Color; | ||
39 | + strikethrough: Color; | ||
40 | + | ||
41 | + rainbow: Color; | ||
42 | + zebra: Color; | ||
43 | + america: Color; | ||
44 | + trap: Color; | ||
45 | + random: Color; | ||
46 | + zalgo: Color; | ||
47 | +} | ||
48 | + | ||
49 | +export function enable(): void; | ||
50 | +export function disable(): void; | ||
51 | +export function setTheme(theme: any): void; | ||
52 | + | ||
53 | +export let enabled: boolean; | ||
54 | + | ||
55 | +export const strip: Color; | ||
56 | +export const stripColors: Color; | ||
57 | + | ||
58 | +export const black: Color; | ||
59 | +export const red: Color; | ||
60 | +export const green: Color; | ||
61 | +export const yellow: Color; | ||
62 | +export const blue: Color; | ||
63 | +export const magenta: Color; | ||
64 | +export const cyan: Color; | ||
65 | +export const white: Color; | ||
66 | +export const gray: Color; | ||
67 | +export const grey: Color; | ||
68 | + | ||
69 | +export const bgBlack: Color; | ||
70 | +export const bgRed: Color; | ||
71 | +export const bgGreen: Color; | ||
72 | +export const bgYellow: Color; | ||
73 | +export const bgBlue: Color; | ||
74 | +export const bgMagenta: Color; | ||
75 | +export const bgCyan: Color; | ||
76 | +export const bgWhite: Color; | ||
77 | + | ||
78 | +export const reset: Color; | ||
79 | +export const bold: Color; | ||
80 | +export const dim: Color; | ||
81 | +export const italic: Color; | ||
82 | +export const underline: Color; | ||
83 | +export const inverse: Color; | ||
84 | +export const hidden: Color; | ||
85 | +export const strikethrough: Color; | ||
86 | + | ||
87 | +export const rainbow: Color; | ||
88 | +export const zebra: Color; | ||
89 | +export const america: Color; | ||
90 | +export const trap: Color; | ||
91 | +export const random: Color; | ||
92 | +export const zalgo: Color; | ||
93 | + | ||
94 | +declare global { | ||
95 | + interface String { | ||
96 | + strip: string; | ||
97 | + stripColors: string; | ||
98 | + | ||
99 | + black: string; | ||
100 | + red: string; | ||
101 | + green: string; | ||
102 | + yellow: string; | ||
103 | + blue: string; | ||
104 | + magenta: string; | ||
105 | + cyan: string; | ||
106 | + white: string; | ||
107 | + gray: string; | ||
108 | + grey: string; | ||
109 | + | ||
110 | + bgBlack: string; | ||
111 | + bgRed: string; | ||
112 | + bgGreen: string; | ||
113 | + bgYellow: string; | ||
114 | + bgBlue: string; | ||
115 | + bgMagenta: string; | ||
116 | + bgCyan: string; | ||
117 | + bgWhite: string; | ||
118 | + | ||
119 | + reset: string; | ||
120 | + // @ts-ignore | ||
121 | + bold: string; | ||
122 | + dim: string; | ||
123 | + italic: string; | ||
124 | + underline: string; | ||
125 | + inverse: string; | ||
126 | + hidden: string; | ||
127 | + strikethrough: string; | ||
128 | + | ||
129 | + rainbow: string; | ||
130 | + zebra: string; | ||
131 | + america: string; | ||
132 | + trap: string; | ||
133 | + random: string; | ||
134 | + zalgo: string; | ||
135 | + } | ||
136 | +} |
node_modules/colors/lib/colors.js
0 → 100644
1 | +/* | ||
2 | + | ||
3 | +The MIT License (MIT) | ||
4 | + | ||
5 | +Original Library | ||
6 | + - Copyright (c) Marak Squires | ||
7 | + | ||
8 | +Additional functionality | ||
9 | + - Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
10 | + | ||
11 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
12 | +of this software and associated documentation files (the "Software"), to deal | ||
13 | +in the Software without restriction, including without limitation the rights | ||
14 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
15 | +copies of the Software, and to permit persons to whom the Software is | ||
16 | +furnished to do so, subject to the following conditions: | ||
17 | + | ||
18 | +The above copyright notice and this permission notice shall be included in | ||
19 | +all copies or substantial portions of the Software. | ||
20 | + | ||
21 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
22 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
23 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
24 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
25 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
26 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
27 | +THE SOFTWARE. | ||
28 | + | ||
29 | +*/ | ||
30 | + | ||
31 | +var colors = {}; | ||
32 | +module['exports'] = colors; | ||
33 | + | ||
34 | +colors.themes = {}; | ||
35 | + | ||
36 | +var util = require('util'); | ||
37 | +var ansiStyles = colors.styles = require('./styles'); | ||
38 | +var defineProps = Object.defineProperties; | ||
39 | +var newLineRegex = new RegExp(/[\r\n]+/g); | ||
40 | + | ||
41 | +colors.supportsColor = require('./system/supports-colors').supportsColor; | ||
42 | + | ||
43 | +if (typeof colors.enabled === 'undefined') { | ||
44 | + colors.enabled = colors.supportsColor() !== false; | ||
45 | +} | ||
46 | + | ||
47 | +colors.enable = function() { | ||
48 | + colors.enabled = true; | ||
49 | +}; | ||
50 | + | ||
51 | +colors.disable = function() { | ||
52 | + colors.enabled = false; | ||
53 | +}; | ||
54 | + | ||
55 | +colors.stripColors = colors.strip = function(str) { | ||
56 | + return ('' + str).replace(/\x1B\[\d+m/g, ''); | ||
57 | +}; | ||
58 | + | ||
59 | +// eslint-disable-next-line no-unused-vars | ||
60 | +var stylize = colors.stylize = function stylize(str, style) { | ||
61 | + if (!colors.enabled) { | ||
62 | + return str+''; | ||
63 | + } | ||
64 | + | ||
65 | + var styleMap = ansiStyles[style]; | ||
66 | + | ||
67 | + // Stylize should work for non-ANSI styles, too | ||
68 | + if(!styleMap && style in colors){ | ||
69 | + // Style maps like trap operate as functions on strings; | ||
70 | + // they don't have properties like open or close. | ||
71 | + return colors[style](str); | ||
72 | + } | ||
73 | + | ||
74 | + return styleMap.open + str + styleMap.close; | ||
75 | +}; | ||
76 | + | ||
77 | +var matchOperatorsRe = /[|\\{}()[\]^$+*?.]/g; | ||
78 | +var escapeStringRegexp = function(str) { | ||
79 | + if (typeof str !== 'string') { | ||
80 | + throw new TypeError('Expected a string'); | ||
81 | + } | ||
82 | + return str.replace(matchOperatorsRe, '\\$&'); | ||
83 | +}; | ||
84 | + | ||
85 | +function build(_styles) { | ||
86 | + var builder = function builder() { | ||
87 | + return applyStyle.apply(builder, arguments); | ||
88 | + }; | ||
89 | + builder._styles = _styles; | ||
90 | + // __proto__ is used because we must return a function, but there is | ||
91 | + // no way to create a function with a different prototype. | ||
92 | + builder.__proto__ = proto; | ||
93 | + return builder; | ||
94 | +} | ||
95 | + | ||
96 | +var styles = (function() { | ||
97 | + var ret = {}; | ||
98 | + ansiStyles.grey = ansiStyles.gray; | ||
99 | + Object.keys(ansiStyles).forEach(function(key) { | ||
100 | + ansiStyles[key].closeRe = | ||
101 | + new RegExp(escapeStringRegexp(ansiStyles[key].close), 'g'); | ||
102 | + ret[key] = { | ||
103 | + get: function() { | ||
104 | + return build(this._styles.concat(key)); | ||
105 | + }, | ||
106 | + }; | ||
107 | + }); | ||
108 | + return ret; | ||
109 | +})(); | ||
110 | + | ||
111 | +var proto = defineProps(function colors() {}, styles); | ||
112 | + | ||
113 | +function applyStyle() { | ||
114 | + var args = Array.prototype.slice.call(arguments); | ||
115 | + | ||
116 | + var str = args.map(function(arg) { | ||
117 | + // Use weak equality check so we can colorize null/undefined in safe mode | ||
118 | + if (arg != null && arg.constructor === String) { | ||
119 | + return arg; | ||
120 | + } else { | ||
121 | + return util.inspect(arg); | ||
122 | + } | ||
123 | + }).join(' '); | ||
124 | + | ||
125 | + if (!colors.enabled || !str) { | ||
126 | + return str; | ||
127 | + } | ||
128 | + | ||
129 | + var newLinesPresent = str.indexOf('\n') != -1; | ||
130 | + | ||
131 | + var nestedStyles = this._styles; | ||
132 | + | ||
133 | + var i = nestedStyles.length; | ||
134 | + while (i--) { | ||
135 | + var code = ansiStyles[nestedStyles[i]]; | ||
136 | + str = code.open + str.replace(code.closeRe, code.open) + code.close; | ||
137 | + if (newLinesPresent) { | ||
138 | + str = str.replace(newLineRegex, function(match) { | ||
139 | + return code.close + match + code.open; | ||
140 | + }); | ||
141 | + } | ||
142 | + } | ||
143 | + | ||
144 | + return str; | ||
145 | +} | ||
146 | + | ||
147 | +colors.setTheme = function(theme) { | ||
148 | + if (typeof theme === 'string') { | ||
149 | + console.log('colors.setTheme now only accepts an object, not a string. ' + | ||
150 | + 'If you are trying to set a theme from a file, it is now your (the ' + | ||
151 | + 'caller\'s) responsibility to require the file. The old syntax ' + | ||
152 | + 'looked like colors.setTheme(__dirname + ' + | ||
153 | + '\'/../themes/generic-logging.js\'); The new syntax looks like '+ | ||
154 | + 'colors.setTheme(require(__dirname + ' + | ||
155 | + '\'/../themes/generic-logging.js\'));'); | ||
156 | + return; | ||
157 | + } | ||
158 | + for (var style in theme) { | ||
159 | + (function(style) { | ||
160 | + colors[style] = function(str) { | ||
161 | + if (typeof theme[style] === 'object') { | ||
162 | + var out = str; | ||
163 | + for (var i in theme[style]) { | ||
164 | + out = colors[theme[style][i]](out); | ||
165 | + } | ||
166 | + return out; | ||
167 | + } | ||
168 | + return colors[theme[style]](str); | ||
169 | + }; | ||
170 | + })(style); | ||
171 | + } | ||
172 | +}; | ||
173 | + | ||
174 | +function init() { | ||
175 | + var ret = {}; | ||
176 | + Object.keys(styles).forEach(function(name) { | ||
177 | + ret[name] = { | ||
178 | + get: function() { | ||
179 | + return build([name]); | ||
180 | + }, | ||
181 | + }; | ||
182 | + }); | ||
183 | + return ret; | ||
184 | +} | ||
185 | + | ||
186 | +var sequencer = function sequencer(map, str) { | ||
187 | + var exploded = str.split(''); | ||
188 | + exploded = exploded.map(map); | ||
189 | + return exploded.join(''); | ||
190 | +}; | ||
191 | + | ||
192 | +// custom formatter methods | ||
193 | +colors.trap = require('./custom/trap'); | ||
194 | +colors.zalgo = require('./custom/zalgo'); | ||
195 | + | ||
196 | +// maps | ||
197 | +colors.maps = {}; | ||
198 | +colors.maps.america = require('./maps/america')(colors); | ||
199 | +colors.maps.zebra = require('./maps/zebra')(colors); | ||
200 | +colors.maps.rainbow = require('./maps/rainbow')(colors); | ||
201 | +colors.maps.random = require('./maps/random')(colors); | ||
202 | + | ||
203 | +for (var map in colors.maps) { | ||
204 | + (function(map) { | ||
205 | + colors[map] = function(str) { | ||
206 | + return sequencer(colors.maps[map], str); | ||
207 | + }; | ||
208 | + })(map); | ||
209 | +} | ||
210 | + | ||
211 | +defineProps(colors, init()); |
node_modules/colors/lib/custom/trap.js
0 → 100644
1 | +module['exports'] = function runTheTrap(text, options) { | ||
2 | + var result = ''; | ||
3 | + text = text || 'Run the trap, drop the bass'; | ||
4 | + text = text.split(''); | ||
5 | + var trap = { | ||
6 | + a: ['\u0040', '\u0104', '\u023a', '\u0245', '\u0394', '\u039b', '\u0414'], | ||
7 | + b: ['\u00df', '\u0181', '\u0243', '\u026e', '\u03b2', '\u0e3f'], | ||
8 | + c: ['\u00a9', '\u023b', '\u03fe'], | ||
9 | + d: ['\u00d0', '\u018a', '\u0500', '\u0501', '\u0502', '\u0503'], | ||
10 | + e: ['\u00cb', '\u0115', '\u018e', '\u0258', '\u03a3', '\u03be', '\u04bc', | ||
11 | + '\u0a6c'], | ||
12 | + f: ['\u04fa'], | ||
13 | + g: ['\u0262'], | ||
14 | + h: ['\u0126', '\u0195', '\u04a2', '\u04ba', '\u04c7', '\u050a'], | ||
15 | + i: ['\u0f0f'], | ||
16 | + j: ['\u0134'], | ||
17 | + k: ['\u0138', '\u04a0', '\u04c3', '\u051e'], | ||
18 | + l: ['\u0139'], | ||
19 | + m: ['\u028d', '\u04cd', '\u04ce', '\u0520', '\u0521', '\u0d69'], | ||
20 | + n: ['\u00d1', '\u014b', '\u019d', '\u0376', '\u03a0', '\u048a'], | ||
21 | + o: ['\u00d8', '\u00f5', '\u00f8', '\u01fe', '\u0298', '\u047a', '\u05dd', | ||
22 | + '\u06dd', '\u0e4f'], | ||
23 | + p: ['\u01f7', '\u048e'], | ||
24 | + q: ['\u09cd'], | ||
25 | + r: ['\u00ae', '\u01a6', '\u0210', '\u024c', '\u0280', '\u042f'], | ||
26 | + s: ['\u00a7', '\u03de', '\u03df', '\u03e8'], | ||
27 | + t: ['\u0141', '\u0166', '\u0373'], | ||
28 | + u: ['\u01b1', '\u054d'], | ||
29 | + v: ['\u05d8'], | ||
30 | + w: ['\u0428', '\u0460', '\u047c', '\u0d70'], | ||
31 | + x: ['\u04b2', '\u04fe', '\u04fc', '\u04fd'], | ||
32 | + y: ['\u00a5', '\u04b0', '\u04cb'], | ||
33 | + z: ['\u01b5', '\u0240'], | ||
34 | + }; | ||
35 | + text.forEach(function(c) { | ||
36 | + c = c.toLowerCase(); | ||
37 | + var chars = trap[c] || [' ']; | ||
38 | + var rand = Math.floor(Math.random() * chars.length); | ||
39 | + if (typeof trap[c] !== 'undefined') { | ||
40 | + result += trap[c][rand]; | ||
41 | + } else { | ||
42 | + result += c; | ||
43 | + } | ||
44 | + }); | ||
45 | + return result; | ||
46 | +}; |
node_modules/colors/lib/custom/zalgo.js
0 → 100644
1 | +// please no | ||
2 | +module['exports'] = function zalgo(text, options) { | ||
3 | + text = text || ' he is here '; | ||
4 | + var soul = { | ||
5 | + 'up': [ | ||
6 | + '̍', '̎', '̄', '̅', | ||
7 | + '̿', '̑', '̆', '̐', | ||
8 | + '͒', '͗', '͑', '̇', | ||
9 | + '̈', '̊', '͂', '̓', | ||
10 | + '̈', '͊', '͋', '͌', | ||
11 | + '̃', '̂', '̌', '͐', | ||
12 | + '̀', '́', '̋', '̏', | ||
13 | + '̒', '̓', '̔', '̽', | ||
14 | + '̉', 'ͣ', 'ͤ', 'ͥ', | ||
15 | + 'ͦ', 'ͧ', 'ͨ', 'ͩ', | ||
16 | + 'ͪ', 'ͫ', 'ͬ', 'ͭ', | ||
17 | + 'ͮ', 'ͯ', '̾', '͛', | ||
18 | + '͆', '̚', | ||
19 | + ], | ||
20 | + 'down': [ | ||
21 | + '̖', '̗', '̘', '̙', | ||
22 | + '̜', '̝', '̞', '̟', | ||
23 | + '̠', '̤', '̥', '̦', | ||
24 | + '̩', '̪', '̫', '̬', | ||
25 | + '̭', '̮', '̯', '̰', | ||
26 | + '̱', '̲', '̳', '̹', | ||
27 | + '̺', '̻', '̼', 'ͅ', | ||
28 | + '͇', '͈', '͉', '͍', | ||
29 | + '͎', '͓', '͔', '͕', | ||
30 | + '͖', '͙', '͚', '̣', | ||
31 | + ], | ||
32 | + 'mid': [ | ||
33 | + '̕', '̛', '̀', '́', | ||
34 | + '͘', '̡', '̢', '̧', | ||
35 | + '̨', '̴', '̵', '̶', | ||
36 | + '͜', '͝', '͞', | ||
37 | + '͟', '͠', '͢', '̸', | ||
38 | + '̷', '͡', ' ҉', | ||
39 | + ], | ||
40 | + }; | ||
41 | + var all = [].concat(soul.up, soul.down, soul.mid); | ||
42 | + | ||
43 | + function randomNumber(range) { | ||
44 | + var r = Math.floor(Math.random() * range); | ||
45 | + return r; | ||
46 | + } | ||
47 | + | ||
48 | + function isChar(character) { | ||
49 | + var bool = false; | ||
50 | + all.filter(function(i) { | ||
51 | + bool = (i === character); | ||
52 | + }); | ||
53 | + return bool; | ||
54 | + } | ||
55 | + | ||
56 | + | ||
57 | + function heComes(text, options) { | ||
58 | + var result = ''; | ||
59 | + var counts; | ||
60 | + var l; | ||
61 | + options = options || {}; | ||
62 | + options['up'] = | ||
63 | + typeof options['up'] !== 'undefined' ? options['up'] : true; | ||
64 | + options['mid'] = | ||
65 | + typeof options['mid'] !== 'undefined' ? options['mid'] : true; | ||
66 | + options['down'] = | ||
67 | + typeof options['down'] !== 'undefined' ? options['down'] : true; | ||
68 | + options['size'] = | ||
69 | + typeof options['size'] !== 'undefined' ? options['size'] : 'maxi'; | ||
70 | + text = text.split(''); | ||
71 | + for (l in text) { | ||
72 | + if (isChar(l)) { | ||
73 | + continue; | ||
74 | + } | ||
75 | + result = result + text[l]; | ||
76 | + counts = {'up': 0, 'down': 0, 'mid': 0}; | ||
77 | + switch (options.size) { | ||
78 | + case 'mini': | ||
79 | + counts.up = randomNumber(8); | ||
80 | + counts.mid = randomNumber(2); | ||
81 | + counts.down = randomNumber(8); | ||
82 | + break; | ||
83 | + case 'maxi': | ||
84 | + counts.up = randomNumber(16) + 3; | ||
85 | + counts.mid = randomNumber(4) + 1; | ||
86 | + counts.down = randomNumber(64) + 3; | ||
87 | + break; | ||
88 | + default: | ||
89 | + counts.up = randomNumber(8) + 1; | ||
90 | + counts.mid = randomNumber(6) / 2; | ||
91 | + counts.down = randomNumber(8) + 1; | ||
92 | + break; | ||
93 | + } | ||
94 | + | ||
95 | + var arr = ['up', 'mid', 'down']; | ||
96 | + for (var d in arr) { | ||
97 | + var index = arr[d]; | ||
98 | + for (var i = 0; i <= counts[index]; i++) { | ||
99 | + if (options[index]) { | ||
100 | + result = result + soul[index][randomNumber(soul[index].length)]; | ||
101 | + } | ||
102 | + } | ||
103 | + } | ||
104 | + } | ||
105 | + return result; | ||
106 | + } | ||
107 | + // don't summon him | ||
108 | + return heComes(text, options); | ||
109 | +}; | ||
110 | + |
1 | +var colors = require('./colors'); | ||
2 | + | ||
3 | +module['exports'] = function() { | ||
4 | + // | ||
5 | + // Extends prototype of native string object to allow for "foo".red syntax | ||
6 | + // | ||
7 | + var addProperty = function(color, func) { | ||
8 | + String.prototype.__defineGetter__(color, func); | ||
9 | + }; | ||
10 | + | ||
11 | + addProperty('strip', function() { | ||
12 | + return colors.strip(this); | ||
13 | + }); | ||
14 | + | ||
15 | + addProperty('stripColors', function() { | ||
16 | + return colors.strip(this); | ||
17 | + }); | ||
18 | + | ||
19 | + addProperty('trap', function() { | ||
20 | + return colors.trap(this); | ||
21 | + }); | ||
22 | + | ||
23 | + addProperty('zalgo', function() { | ||
24 | + return colors.zalgo(this); | ||
25 | + }); | ||
26 | + | ||
27 | + addProperty('zebra', function() { | ||
28 | + return colors.zebra(this); | ||
29 | + }); | ||
30 | + | ||
31 | + addProperty('rainbow', function() { | ||
32 | + return colors.rainbow(this); | ||
33 | + }); | ||
34 | + | ||
35 | + addProperty('random', function() { | ||
36 | + return colors.random(this); | ||
37 | + }); | ||
38 | + | ||
39 | + addProperty('america', function() { | ||
40 | + return colors.america(this); | ||
41 | + }); | ||
42 | + | ||
43 | + // | ||
44 | + // Iterate through all default styles and colors | ||
45 | + // | ||
46 | + var x = Object.keys(colors.styles); | ||
47 | + x.forEach(function(style) { | ||
48 | + addProperty(style, function() { | ||
49 | + return colors.stylize(this, style); | ||
50 | + }); | ||
51 | + }); | ||
52 | + | ||
53 | + function applyTheme(theme) { | ||
54 | + // | ||
55 | + // Remark: This is a list of methods that exist | ||
56 | + // on String that you should not overwrite. | ||
57 | + // | ||
58 | + var stringPrototypeBlacklist = [ | ||
59 | + '__defineGetter__', '__defineSetter__', '__lookupGetter__', | ||
60 | + '__lookupSetter__', 'charAt', 'constructor', 'hasOwnProperty', | ||
61 | + 'isPrototypeOf', 'propertyIsEnumerable', 'toLocaleString', 'toString', | ||
62 | + 'valueOf', 'charCodeAt', 'indexOf', 'lastIndexOf', 'length', | ||
63 | + 'localeCompare', 'match', 'repeat', 'replace', 'search', 'slice', | ||
64 | + 'split', 'substring', 'toLocaleLowerCase', 'toLocaleUpperCase', | ||
65 | + 'toLowerCase', 'toUpperCase', 'trim', 'trimLeft', 'trimRight', | ||
66 | + ]; | ||
67 | + | ||
68 | + Object.keys(theme).forEach(function(prop) { | ||
69 | + if (stringPrototypeBlacklist.indexOf(prop) !== -1) { | ||
70 | + console.log('warn: '.red + ('String.prototype' + prop).magenta + | ||
71 | + ' is probably something you don\'t want to override. ' + | ||
72 | + 'Ignoring style name'); | ||
73 | + } else { | ||
74 | + if (typeof(theme[prop]) === 'string') { | ||
75 | + colors[prop] = colors[theme[prop]]; | ||
76 | + addProperty(prop, function() { | ||
77 | + return colors[prop](this); | ||
78 | + }); | ||
79 | + } else { | ||
80 | + var themePropApplicator = function(str) { | ||
81 | + var ret = str || this; | ||
82 | + for (var t = 0; t < theme[prop].length; t++) { | ||
83 | + ret = colors[theme[prop][t]](ret); | ||
84 | + } | ||
85 | + return ret; | ||
86 | + }; | ||
87 | + addProperty(prop, themePropApplicator); | ||
88 | + colors[prop] = function(str) { | ||
89 | + return themePropApplicator(str); | ||
90 | + }; | ||
91 | + } | ||
92 | + } | ||
93 | + }); | ||
94 | + } | ||
95 | + | ||
96 | + colors.setTheme = function(theme) { | ||
97 | + if (typeof theme === 'string') { | ||
98 | + console.log('colors.setTheme now only accepts an object, not a string. ' + | ||
99 | + 'If you are trying to set a theme from a file, it is now your (the ' + | ||
100 | + 'caller\'s) responsibility to require the file. The old syntax ' + | ||
101 | + 'looked like colors.setTheme(__dirname + ' + | ||
102 | + '\'/../themes/generic-logging.js\'); The new syntax looks like '+ | ||
103 | + 'colors.setTheme(require(__dirname + ' + | ||
104 | + '\'/../themes/generic-logging.js\'));'); | ||
105 | + return; | ||
106 | + } else { | ||
107 | + applyTheme(theme); | ||
108 | + } | ||
109 | + }; | ||
110 | +}; |
node_modules/colors/lib/index.js
0 → 100644
1 | +var colors = require('./colors'); | ||
2 | +module['exports'] = colors; | ||
3 | + | ||
4 | +// Remark: By default, colors will add style properties to String.prototype. | ||
5 | +// | ||
6 | +// If you don't wish to extend String.prototype, you can do this instead and | ||
7 | +// native String will not be touched: | ||
8 | +// | ||
9 | +// var colors = require('colors/safe); | ||
10 | +// colors.red("foo") | ||
11 | +// | ||
12 | +// | ||
13 | +require('./extendStringPrototype')(); |
node_modules/colors/lib/maps/america.js
0 → 100644
node_modules/colors/lib/maps/rainbow.js
0 → 100644
1 | +module['exports'] = function(colors) { | ||
2 | + // RoY G BiV | ||
3 | + var rainbowColors = ['red', 'yellow', 'green', 'blue', 'magenta']; | ||
4 | + return function(letter, i, exploded) { | ||
5 | + if (letter === ' ') { | ||
6 | + return letter; | ||
7 | + } else { | ||
8 | + return colors[rainbowColors[i++ % rainbowColors.length]](letter); | ||
9 | + } | ||
10 | + }; | ||
11 | +}; | ||
12 | + |
node_modules/colors/lib/maps/random.js
0 → 100644
1 | +module['exports'] = function(colors) { | ||
2 | + var available = ['underline', 'inverse', 'grey', 'yellow', 'red', 'green', | ||
3 | + 'blue', 'white', 'cyan', 'magenta', 'brightYellow', 'brightRed', | ||
4 | + 'brightGreen', 'brightBlue', 'brightWhite', 'brightCyan', 'brightMagenta']; | ||
5 | + return function(letter, i, exploded) { | ||
6 | + return letter === ' ' ? letter : | ||
7 | + colors[ | ||
8 | + available[Math.round(Math.random() * (available.length - 2))] | ||
9 | + ](letter); | ||
10 | + }; | ||
11 | +}; |
node_modules/colors/lib/maps/zebra.js
0 → 100644
node_modules/colors/lib/styles.js
0 → 100644
1 | +/* | ||
2 | +The MIT License (MIT) | ||
3 | + | ||
4 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
5 | + | ||
6 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
7 | +of this software and associated documentation files (the "Software"), to deal | ||
8 | +in the Software without restriction, including without limitation the rights | ||
9 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
10 | +copies of the Software, and to permit persons to whom the Software is | ||
11 | +furnished to do so, subject to the following conditions: | ||
12 | + | ||
13 | +The above copyright notice and this permission notice shall be included in | ||
14 | +all copies or substantial portions of the Software. | ||
15 | + | ||
16 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
17 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
18 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
19 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
20 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
21 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
22 | +THE SOFTWARE. | ||
23 | + | ||
24 | +*/ | ||
25 | + | ||
26 | +var styles = {}; | ||
27 | +module['exports'] = styles; | ||
28 | + | ||
29 | +var codes = { | ||
30 | + reset: [0, 0], | ||
31 | + | ||
32 | + bold: [1, 22], | ||
33 | + dim: [2, 22], | ||
34 | + italic: [3, 23], | ||
35 | + underline: [4, 24], | ||
36 | + inverse: [7, 27], | ||
37 | + hidden: [8, 28], | ||
38 | + strikethrough: [9, 29], | ||
39 | + | ||
40 | + black: [30, 39], | ||
41 | + red: [31, 39], | ||
42 | + green: [32, 39], | ||
43 | + yellow: [33, 39], | ||
44 | + blue: [34, 39], | ||
45 | + magenta: [35, 39], | ||
46 | + cyan: [36, 39], | ||
47 | + white: [37, 39], | ||
48 | + gray: [90, 39], | ||
49 | + grey: [90, 39], | ||
50 | + | ||
51 | + brightRed: [91, 39], | ||
52 | + brightGreen: [92, 39], | ||
53 | + brightYellow: [93, 39], | ||
54 | + brightBlue: [94, 39], | ||
55 | + brightMagenta: [95, 39], | ||
56 | + brightCyan: [96, 39], | ||
57 | + brightWhite: [97, 39], | ||
58 | + | ||
59 | + bgBlack: [40, 49], | ||
60 | + bgRed: [41, 49], | ||
61 | + bgGreen: [42, 49], | ||
62 | + bgYellow: [43, 49], | ||
63 | + bgBlue: [44, 49], | ||
64 | + bgMagenta: [45, 49], | ||
65 | + bgCyan: [46, 49], | ||
66 | + bgWhite: [47, 49], | ||
67 | + bgGray: [100, 49], | ||
68 | + bgGrey: [100, 49], | ||
69 | + | ||
70 | + bgBrightRed: [101, 49], | ||
71 | + bgBrightGreen: [102, 49], | ||
72 | + bgBrightYellow: [103, 49], | ||
73 | + bgBrightBlue: [104, 49], | ||
74 | + bgBrightMagenta: [105, 49], | ||
75 | + bgBrightCyan: [106, 49], | ||
76 | + bgBrightWhite: [107, 49], | ||
77 | + | ||
78 | + // legacy styles for colors pre v1.0.0 | ||
79 | + blackBG: [40, 49], | ||
80 | + redBG: [41, 49], | ||
81 | + greenBG: [42, 49], | ||
82 | + yellowBG: [43, 49], | ||
83 | + blueBG: [44, 49], | ||
84 | + magentaBG: [45, 49], | ||
85 | + cyanBG: [46, 49], | ||
86 | + whiteBG: [47, 49], | ||
87 | + | ||
88 | +}; | ||
89 | + | ||
90 | +Object.keys(codes).forEach(function(key) { | ||
91 | + var val = codes[key]; | ||
92 | + var style = styles[key] = []; | ||
93 | + style.open = '\u001b[' + val[0] + 'm'; | ||
94 | + style.close = '\u001b[' + val[1] + 'm'; | ||
95 | +}); |
node_modules/colors/lib/system/has-flag.js
0 → 100644
1 | +/* | ||
2 | +MIT License | ||
3 | + | ||
4 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
5 | + | ||
6 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
7 | +this software and associated documentation files (the "Software"), to deal in | ||
8 | +the Software without restriction, including without limitation the rights to | ||
9 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
10 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
11 | +so, subject to the following conditions: | ||
12 | + | ||
13 | +The above copyright notice and this permission notice shall be included in all | ||
14 | +copies or substantial portions of the Software. | ||
15 | + | ||
16 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
17 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
18 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
19 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
20 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
21 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
22 | +SOFTWARE. | ||
23 | +*/ | ||
24 | + | ||
25 | +'use strict'; | ||
26 | + | ||
27 | +module.exports = function(flag, argv) { | ||
28 | + argv = argv || process.argv; | ||
29 | + | ||
30 | + var terminatorPos = argv.indexOf('--'); | ||
31 | + var prefix = /^-{1,2}/.test(flag) ? '' : '--'; | ||
32 | + var pos = argv.indexOf(prefix + flag); | ||
33 | + | ||
34 | + return pos !== -1 && (terminatorPos === -1 ? true : pos < terminatorPos); | ||
35 | +}; |
1 | +/* | ||
2 | +The MIT License (MIT) | ||
3 | + | ||
4 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
5 | + | ||
6 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
7 | +of this software and associated documentation files (the "Software"), to deal | ||
8 | +in the Software without restriction, including without limitation the rights | ||
9 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
10 | +copies of the Software, and to permit persons to whom the Software is | ||
11 | +furnished to do so, subject to the following conditions: | ||
12 | + | ||
13 | +The above copyright notice and this permission notice shall be included in | ||
14 | +all copies or substantial portions of the Software. | ||
15 | + | ||
16 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
17 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
18 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
19 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
20 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
21 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
22 | +THE SOFTWARE. | ||
23 | + | ||
24 | +*/ | ||
25 | + | ||
26 | +'use strict'; | ||
27 | + | ||
28 | +var os = require('os'); | ||
29 | +var hasFlag = require('./has-flag.js'); | ||
30 | + | ||
31 | +var env = process.env; | ||
32 | + | ||
33 | +var forceColor = void 0; | ||
34 | +if (hasFlag('no-color') || hasFlag('no-colors') || hasFlag('color=false')) { | ||
35 | + forceColor = false; | ||
36 | +} else if (hasFlag('color') || hasFlag('colors') || hasFlag('color=true') | ||
37 | + || hasFlag('color=always')) { | ||
38 | + forceColor = true; | ||
39 | +} | ||
40 | +if ('FORCE_COLOR' in env) { | ||
41 | + forceColor = env.FORCE_COLOR.length === 0 | ||
42 | + || parseInt(env.FORCE_COLOR, 10) !== 0; | ||
43 | +} | ||
44 | + | ||
45 | +function translateLevel(level) { | ||
46 | + if (level === 0) { | ||
47 | + return false; | ||
48 | + } | ||
49 | + | ||
50 | + return { | ||
51 | + level: level, | ||
52 | + hasBasic: true, | ||
53 | + has256: level >= 2, | ||
54 | + has16m: level >= 3, | ||
55 | + }; | ||
56 | +} | ||
57 | + | ||
58 | +function supportsColor(stream) { | ||
59 | + if (forceColor === false) { | ||
60 | + return 0; | ||
61 | + } | ||
62 | + | ||
63 | + if (hasFlag('color=16m') || hasFlag('color=full') | ||
64 | + || hasFlag('color=truecolor')) { | ||
65 | + return 3; | ||
66 | + } | ||
67 | + | ||
68 | + if (hasFlag('color=256')) { | ||
69 | + return 2; | ||
70 | + } | ||
71 | + | ||
72 | + if (stream && !stream.isTTY && forceColor !== true) { | ||
73 | + return 0; | ||
74 | + } | ||
75 | + | ||
76 | + var min = forceColor ? 1 : 0; | ||
77 | + | ||
78 | + if (process.platform === 'win32') { | ||
79 | + // Node.js 7.5.0 is the first version of Node.js to include a patch to | ||
80 | + // libuv that enables 256 color output on Windows. Anything earlier and it | ||
81 | + // won't work. However, here we target Node.js 8 at minimum as it is an LTS | ||
82 | + // release, and Node.js 7 is not. Windows 10 build 10586 is the first | ||
83 | + // Windows release that supports 256 colors. Windows 10 build 14931 is the | ||
84 | + // first release that supports 16m/TrueColor. | ||
85 | + var osRelease = os.release().split('.'); | ||
86 | + if (Number(process.versions.node.split('.')[0]) >= 8 | ||
87 | + && Number(osRelease[0]) >= 10 && Number(osRelease[2]) >= 10586) { | ||
88 | + return Number(osRelease[2]) >= 14931 ? 3 : 2; | ||
89 | + } | ||
90 | + | ||
91 | + return 1; | ||
92 | + } | ||
93 | + | ||
94 | + if ('CI' in env) { | ||
95 | + if (['TRAVIS', 'CIRCLECI', 'APPVEYOR', 'GITLAB_CI'].some(function(sign) { | ||
96 | + return sign in env; | ||
97 | + }) || env.CI_NAME === 'codeship') { | ||
98 | + return 1; | ||
99 | + } | ||
100 | + | ||
101 | + return min; | ||
102 | + } | ||
103 | + | ||
104 | + if ('TEAMCITY_VERSION' in env) { | ||
105 | + return (/^(9\.(0*[1-9]\d*)\.|\d{2,}\.)/.test(env.TEAMCITY_VERSION) ? 1 : 0 | ||
106 | + ); | ||
107 | + } | ||
108 | + | ||
109 | + if ('TERM_PROGRAM' in env) { | ||
110 | + var version = parseInt((env.TERM_PROGRAM_VERSION || '').split('.')[0], 10); | ||
111 | + | ||
112 | + switch (env.TERM_PROGRAM) { | ||
113 | + case 'iTerm.app': | ||
114 | + return version >= 3 ? 3 : 2; | ||
115 | + case 'Hyper': | ||
116 | + return 3; | ||
117 | + case 'Apple_Terminal': | ||
118 | + return 2; | ||
119 | + // No default | ||
120 | + } | ||
121 | + } | ||
122 | + | ||
123 | + if (/-256(color)?$/i.test(env.TERM)) { | ||
124 | + return 2; | ||
125 | + } | ||
126 | + | ||
127 | + if (/^screen|^xterm|^vt100|^rxvt|color|ansi|cygwin|linux/i.test(env.TERM)) { | ||
128 | + return 1; | ||
129 | + } | ||
130 | + | ||
131 | + if ('COLORTERM' in env) { | ||
132 | + return 1; | ||
133 | + } | ||
134 | + | ||
135 | + if (env.TERM === 'dumb') { | ||
136 | + return min; | ||
137 | + } | ||
138 | + | ||
139 | + return min; | ||
140 | +} | ||
141 | + | ||
142 | +function getSupportLevel(stream) { | ||
143 | + var level = supportsColor(stream); | ||
144 | + return translateLevel(level); | ||
145 | +} | ||
146 | + | ||
147 | +module.exports = { | ||
148 | + supportsColor: getSupportLevel, | ||
149 | + stdout: getSupportLevel(process.stdout), | ||
150 | + stderr: getSupportLevel(process.stderr), | ||
151 | +}; |
node_modules/colors/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "colors", | ||
3 | + "description": "get colors in your node.js console", | ||
4 | + "version": "1.4.0", | ||
5 | + "author": "Marak Squires", | ||
6 | + "contributors": [ | ||
7 | + { | ||
8 | + "name": "DABH", | ||
9 | + "url": "https://github.com/DABH" | ||
10 | + } | ||
11 | + ], | ||
12 | + "homepage": "https://github.com/Marak/colors.js", | ||
13 | + "bugs": "https://github.com/Marak/colors.js/issues", | ||
14 | + "keywords": [ | ||
15 | + "ansi", | ||
16 | + "terminal", | ||
17 | + "colors" | ||
18 | + ], | ||
19 | + "repository": { | ||
20 | + "type": "git", | ||
21 | + "url": "http://github.com/Marak/colors.js.git" | ||
22 | + }, | ||
23 | + "license": "MIT", | ||
24 | + "scripts": { | ||
25 | + "lint": "eslint . --fix", | ||
26 | + "test": "node tests/basic-test.js && node tests/safe-test.js" | ||
27 | + }, | ||
28 | + "engines": { | ||
29 | + "node": ">=0.1.90" | ||
30 | + }, | ||
31 | + "main": "lib/index.js", | ||
32 | + "files": [ | ||
33 | + "examples", | ||
34 | + "lib", | ||
35 | + "LICENSE", | ||
36 | + "safe.js", | ||
37 | + "themes", | ||
38 | + "index.d.ts", | ||
39 | + "safe.d.ts" | ||
40 | + ], | ||
41 | + "devDependencies": { | ||
42 | + "eslint": "^5.2.0", | ||
43 | + "eslint-config-google": "^0.11.0" | ||
44 | + } | ||
45 | +} |
node_modules/colors/safe.d.ts
0 → 100644
1 | +// Type definitions for Colors.js 1.2 | ||
2 | +// Project: https://github.com/Marak/colors.js | ||
3 | +// Definitions by: Bart van der Schoor <https://github.com/Bartvds>, Staffan Eketorp <https://github.com/staeke> | ||
4 | +// Definitions: https://github.com/Marak/colors.js | ||
5 | + | ||
6 | +export const enabled: boolean; | ||
7 | +export function enable(): void; | ||
8 | +export function disable(): void; | ||
9 | +export function setTheme(theme: any): void; | ||
10 | + | ||
11 | +export function strip(str: string): string; | ||
12 | +export function stripColors(str: string): string; | ||
13 | + | ||
14 | +export function black(str: string): string; | ||
15 | +export function red(str: string): string; | ||
16 | +export function green(str: string): string; | ||
17 | +export function yellow(str: string): string; | ||
18 | +export function blue(str: string): string; | ||
19 | +export function magenta(str: string): string; | ||
20 | +export function cyan(str: string): string; | ||
21 | +export function white(str: string): string; | ||
22 | +export function gray(str: string): string; | ||
23 | +export function grey(str: string): string; | ||
24 | + | ||
25 | +export function bgBlack(str: string): string; | ||
26 | +export function bgRed(str: string): string; | ||
27 | +export function bgGreen(str: string): string; | ||
28 | +export function bgYellow(str: string): string; | ||
29 | +export function bgBlue(str: string): string; | ||
30 | +export function bgMagenta(str: string): string; | ||
31 | +export function bgCyan(str: string): string; | ||
32 | +export function bgWhite(str: string): string; | ||
33 | + | ||
34 | +export function reset(str: string): string; | ||
35 | +export function bold(str: string): string; | ||
36 | +export function dim(str: string): string; | ||
37 | +export function italic(str: string): string; | ||
38 | +export function underline(str: string): string; | ||
39 | +export function inverse(str: string): string; | ||
40 | +export function hidden(str: string): string; | ||
41 | +export function strikethrough(str: string): string; | ||
42 | + | ||
43 | +export function rainbow(str: string): string; | ||
44 | +export function zebra(str: string): string; | ||
45 | +export function america(str: string): string; | ||
46 | +export function trap(str: string): string; | ||
47 | +export function random(str: string): string; | ||
48 | +export function zalgo(str: string): string; |
node_modules/colors/safe.js
0 → 100644
node_modules/concat-map/.travis.yml
0 → 100644
node_modules/concat-map/LICENSE
0 → 100644
1 | +This software is released under the MIT license: | ||
2 | + | ||
3 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
4 | +this software and associated documentation files (the "Software"), to deal in | ||
5 | +the Software without restriction, including without limitation the rights to | ||
6 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of | ||
7 | +the Software, and to permit persons to whom the Software is furnished to do so, | ||
8 | +subject to the following conditions: | ||
9 | + | ||
10 | +The above copyright notice and this permission notice shall be included in all | ||
11 | +copies or substantial portions of the Software. | ||
12 | + | ||
13 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
14 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS | ||
15 | +FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR | ||
16 | +COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER | ||
17 | +IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN | ||
18 | +CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
node_modules/concat-map/README.markdown
0 → 100644
1 | +concat-map | ||
2 | +========== | ||
3 | + | ||
4 | +Concatenative mapdashery. | ||
5 | + | ||
6 | +[](http://ci.testling.com/substack/node-concat-map) | ||
7 | + | ||
8 | +[](http://travis-ci.org/substack/node-concat-map) | ||
9 | + | ||
10 | +example | ||
11 | +======= | ||
12 | + | ||
13 | +``` js | ||
14 | +var concatMap = require('concat-map'); | ||
15 | +var xs = [ 1, 2, 3, 4, 5, 6 ]; | ||
16 | +var ys = concatMap(xs, function (x) { | ||
17 | + return x % 2 ? [ x - 0.1, x, x + 0.1 ] : []; | ||
18 | +}); | ||
19 | +console.dir(ys); | ||
20 | +``` | ||
21 | + | ||
22 | +*** | ||
23 | + | ||
24 | +``` | ||
25 | +[ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ] | ||
26 | +``` | ||
27 | + | ||
28 | +methods | ||
29 | +======= | ||
30 | + | ||
31 | +``` js | ||
32 | +var concatMap = require('concat-map') | ||
33 | +``` | ||
34 | + | ||
35 | +concatMap(xs, fn) | ||
36 | +----------------- | ||
37 | + | ||
38 | +Return an array of concatenated elements by calling `fn(x, i)` for each element | ||
39 | +`x` and each index `i` in the array `xs`. | ||
40 | + | ||
41 | +When `fn(x, i)` returns an array, its result will be concatenated with the | ||
42 | +result array. If `fn(x, i)` returns anything else, that value will be pushed | ||
43 | +onto the end of the result array. | ||
44 | + | ||
45 | +install | ||
46 | +======= | ||
47 | + | ||
48 | +With [npm](http://npmjs.org) do: | ||
49 | + | ||
50 | +``` | ||
51 | +npm install concat-map | ||
52 | +``` | ||
53 | + | ||
54 | +license | ||
55 | +======= | ||
56 | + | ||
57 | +MIT | ||
58 | + | ||
59 | +notes | ||
60 | +===== | ||
61 | + | ||
62 | +This module was written while sitting high above the ground in a tree. |
node_modules/concat-map/example/map.js
0 → 100644
node_modules/concat-map/index.js
0 → 100644
1 | +module.exports = function (xs, fn) { | ||
2 | + var res = []; | ||
3 | + for (var i = 0; i < xs.length; i++) { | ||
4 | + var x = fn(xs[i], i); | ||
5 | + if (isArray(x)) res.push.apply(res, x); | ||
6 | + else res.push(x); | ||
7 | + } | ||
8 | + return res; | ||
9 | +}; | ||
10 | + | ||
11 | +var isArray = Array.isArray || function (xs) { | ||
12 | + return Object.prototype.toString.call(xs) === '[object Array]'; | ||
13 | +}; |
node_modules/concat-map/package.json
0 → 100644
1 | +{ | ||
2 | + "name" : "concat-map", | ||
3 | + "description" : "concatenative mapdashery", | ||
4 | + "version" : "0.0.1", | ||
5 | + "repository" : { | ||
6 | + "type" : "git", | ||
7 | + "url" : "git://github.com/substack/node-concat-map.git" | ||
8 | + }, | ||
9 | + "main" : "index.js", | ||
10 | + "keywords" : [ | ||
11 | + "concat", | ||
12 | + "concatMap", | ||
13 | + "map", | ||
14 | + "functional", | ||
15 | + "higher-order" | ||
16 | + ], | ||
17 | + "directories" : { | ||
18 | + "example" : "example", | ||
19 | + "test" : "test" | ||
20 | + }, | ||
21 | + "scripts" : { | ||
22 | + "test" : "tape test/*.js" | ||
23 | + }, | ||
24 | + "devDependencies" : { | ||
25 | + "tape" : "~2.4.0" | ||
26 | + }, | ||
27 | + "license" : "MIT", | ||
28 | + "author" : { | ||
29 | + "name" : "James Halliday", | ||
30 | + "email" : "mail@substack.net", | ||
31 | + "url" : "http://substack.net" | ||
32 | + }, | ||
33 | + "testling" : { | ||
34 | + "files" : "test/*.js", | ||
35 | + "browsers" : { | ||
36 | + "ie" : [ 6, 7, 8, 9 ], | ||
37 | + "ff" : [ 3.5, 10, 15.0 ], | ||
38 | + "chrome" : [ 10, 22 ], | ||
39 | + "safari" : [ 5.1 ], | ||
40 | + "opera" : [ 12 ] | ||
41 | + } | ||
42 | + } | ||
43 | +} |
node_modules/concat-map/test/map.js
0 → 100644
1 | +var concatMap = require('../'); | ||
2 | +var test = require('tape'); | ||
3 | + | ||
4 | +test('empty or not', function (t) { | ||
5 | + var xs = [ 1, 2, 3, 4, 5, 6 ]; | ||
6 | + var ixes = []; | ||
7 | + var ys = concatMap(xs, function (x, ix) { | ||
8 | + ixes.push(ix); | ||
9 | + return x % 2 ? [ x - 0.1, x, x + 0.1 ] : []; | ||
10 | + }); | ||
11 | + t.same(ys, [ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]); | ||
12 | + t.same(ixes, [ 0, 1, 2, 3, 4, 5 ]); | ||
13 | + t.end(); | ||
14 | +}); | ||
15 | + | ||
16 | +test('always something', function (t) { | ||
17 | + var xs = [ 'a', 'b', 'c', 'd' ]; | ||
18 | + var ys = concatMap(xs, function (x) { | ||
19 | + return x === 'b' ? [ 'B', 'B', 'B' ] : [ x ]; | ||
20 | + }); | ||
21 | + t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]); | ||
22 | + t.end(); | ||
23 | +}); | ||
24 | + | ||
25 | +test('scalars', function (t) { | ||
26 | + var xs = [ 'a', 'b', 'c', 'd' ]; | ||
27 | + var ys = concatMap(xs, function (x) { | ||
28 | + return x === 'b' ? [ 'B', 'B', 'B' ] : x; | ||
29 | + }); | ||
30 | + t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]); | ||
31 | + t.end(); | ||
32 | +}); | ||
33 | + | ||
34 | +test('undefs', function (t) { | ||
35 | + var xs = [ 'a', 'b', 'c', 'd' ]; | ||
36 | + var ys = concatMap(xs, function () {}); | ||
37 | + t.same(ys, [ undefined, undefined, undefined, undefined ]); | ||
38 | + t.end(); | ||
39 | +}); |
node_modules/file-type/index.d.ts
0 → 100644
1 | +/// <reference types="node"/> | ||
2 | +import {Readable as ReadableStream} from 'stream'; | ||
3 | + | ||
4 | +declare namespace fileType { | ||
5 | + type FileType = | ||
6 | + | 'jpg' | ||
7 | + | 'png' | ||
8 | + | 'apng' | ||
9 | + | 'gif' | ||
10 | + | 'webp' | ||
11 | + | 'flif' | ||
12 | + | 'cr2' | ||
13 | + | 'orf' | ||
14 | + | 'arw' | ||
15 | + | 'dng' | ||
16 | + | 'nef' | ||
17 | + | 'rw2' | ||
18 | + | 'raf' | ||
19 | + | 'tif' | ||
20 | + | 'bmp' | ||
21 | + | 'jxr' | ||
22 | + | 'psd' | ||
23 | + | 'zip' | ||
24 | + | 'tar' | ||
25 | + | 'rar' | ||
26 | + | 'gz' | ||
27 | + | 'bz2' | ||
28 | + | '7z' | ||
29 | + | 'dmg' | ||
30 | + | 'mp4' | ||
31 | + | 'mid' | ||
32 | + | 'mkv' | ||
33 | + | 'webm' | ||
34 | + | 'mov' | ||
35 | + | 'avi' | ||
36 | + | 'wmv' | ||
37 | + | 'mpg' | ||
38 | + | 'mp2' | ||
39 | + | 'mp3' | ||
40 | + | 'm4a' | ||
41 | + | 'ogg' | ||
42 | + | 'opus' | ||
43 | + | 'flac' | ||
44 | + | 'wav' | ||
45 | + | 'qcp' | ||
46 | + | 'amr' | ||
47 | + | 'pdf' | ||
48 | + | 'epub' | ||
49 | + | 'mobi' | ||
50 | + | 'exe' | ||
51 | + | 'swf' | ||
52 | + | 'rtf' | ||
53 | + | 'woff' | ||
54 | + | 'woff2' | ||
55 | + | 'eot' | ||
56 | + | 'ttf' | ||
57 | + | 'otf' | ||
58 | + | 'ico' | ||
59 | + | 'flv' | ||
60 | + | 'ps' | ||
61 | + | 'xz' | ||
62 | + | 'sqlite' | ||
63 | + | 'nes' | ||
64 | + | 'crx' | ||
65 | + | 'xpi' | ||
66 | + | 'cab' | ||
67 | + | 'deb' | ||
68 | + | 'ar' | ||
69 | + | 'rpm' | ||
70 | + | 'Z' | ||
71 | + | 'lz' | ||
72 | + | 'msi' | ||
73 | + | 'mxf' | ||
74 | + | 'mts' | ||
75 | + | 'wasm' | ||
76 | + | 'blend' | ||
77 | + | 'bpg' | ||
78 | + | 'docx' | ||
79 | + | 'pptx' | ||
80 | + | 'xlsx' | ||
81 | + | '3gp' | ||
82 | + | '3g2' | ||
83 | + | 'jp2' | ||
84 | + | 'jpm' | ||
85 | + | 'jpx' | ||
86 | + | 'mj2' | ||
87 | + | 'aif' | ||
88 | + | 'odt' | ||
89 | + | 'ods' | ||
90 | + | 'odp' | ||
91 | + | 'xml' | ||
92 | + | 'heic' | ||
93 | + | 'cur' | ||
94 | + | 'ktx' | ||
95 | + | 'ape' | ||
96 | + | 'wv' | ||
97 | + | 'asf' | ||
98 | + | 'wma' | ||
99 | + | 'dcm' | ||
100 | + | 'mpc' | ||
101 | + | 'ics' | ||
102 | + | 'glb' | ||
103 | + | 'pcap' | ||
104 | + | 'dsf' | ||
105 | + | 'lnk' | ||
106 | + | 'alias' | ||
107 | + | 'voc' | ||
108 | + | 'ac3' | ||
109 | + | 'm4b' | ||
110 | + | 'm4p' | ||
111 | + | 'm4v' | ||
112 | + | 'f4a' | ||
113 | + | 'f4b' | ||
114 | + | 'f4p' | ||
115 | + | 'f4v' | ||
116 | + | 'mie' | ||
117 | + | 'ogv' | ||
118 | + | 'ogm' | ||
119 | + | 'oga' | ||
120 | + | 'spx' | ||
121 | + | 'ogx' | ||
122 | + | 'arrow' | ||
123 | + | 'shp'; | ||
124 | + | ||
125 | + type MimeType = | ||
126 | + | 'image/jpeg' | ||
127 | + | 'image/png' | ||
128 | + | 'image/gif' | ||
129 | + | 'image/webp' | ||
130 | + | 'image/flif' | ||
131 | + | 'image/x-canon-cr2' | ||
132 | + | 'image/tiff' | ||
133 | + | 'image/bmp' | ||
134 | + | 'image/vnd.ms-photo' | ||
135 | + | 'image/vnd.adobe.photoshop' | ||
136 | + | 'application/epub+zip' | ||
137 | + | 'application/x-xpinstall' | ||
138 | + | 'application/vnd.oasis.opendocument.text' | ||
139 | + | 'application/vnd.oasis.opendocument.spreadsheet' | ||
140 | + | 'application/vnd.oasis.opendocument.presentation' | ||
141 | + | 'application/vnd.openxmlformats-officedocument.wordprocessingml.document' | ||
142 | + | 'application/vnd.openxmlformats-officedocument.presentationml.presentation' | ||
143 | + | 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet' | ||
144 | + | 'application/zip' | ||
145 | + | 'application/x-tar' | ||
146 | + | 'application/x-rar-compressed' | ||
147 | + | 'application/gzip' | ||
148 | + | 'application/x-bzip2' | ||
149 | + | 'application/x-7z-compressed' | ||
150 | + | 'application/x-apple-diskimage' | ||
151 | + | 'video/mp4' | ||
152 | + | 'audio/midi' | ||
153 | + | 'video/x-matroska' | ||
154 | + | 'video/webm' | ||
155 | + | 'video/quicktime' | ||
156 | + | 'video/vnd.avi' | ||
157 | + | 'audio/vnd.wave' | ||
158 | + | 'audio/qcelp' | ||
159 | + | 'audio/x-ms-wma' | ||
160 | + | 'video/x-ms-asf' | ||
161 | + | 'application/vnd.ms-asf' | ||
162 | + | 'video/mpeg' | ||
163 | + | 'video/3gpp' | ||
164 | + | 'audio/mpeg' | ||
165 | + | 'audio/mp4' // RFC 4337 | ||
166 | + | 'audio/opus' | ||
167 | + | 'video/ogg' | ||
168 | + | 'audio/ogg' | ||
169 | + | 'application/ogg' | ||
170 | + | 'audio/x-flac' | ||
171 | + | 'audio/ape' | ||
172 | + | 'audio/wavpack' | ||
173 | + | 'audio/amr' | ||
174 | + | 'application/pdf' | ||
175 | + | 'application/x-msdownload' | ||
176 | + | 'application/x-shockwave-flash' | ||
177 | + | 'application/rtf' | ||
178 | + | 'application/wasm' | ||
179 | + | 'font/woff' | ||
180 | + | 'font/woff2' | ||
181 | + | 'application/vnd.ms-fontobject' | ||
182 | + | 'font/ttf' | ||
183 | + | 'font/otf' | ||
184 | + | 'image/x-icon' | ||
185 | + | 'video/x-flv' | ||
186 | + | 'application/postscript' | ||
187 | + | 'application/x-xz' | ||
188 | + | 'application/x-sqlite3' | ||
189 | + | 'application/x-nintendo-nes-rom' | ||
190 | + | 'application/x-google-chrome-extension' | ||
191 | + | 'application/vnd.ms-cab-compressed' | ||
192 | + | 'application/x-deb' | ||
193 | + | 'application/x-unix-archive' | ||
194 | + | 'application/x-rpm' | ||
195 | + | 'application/x-compress' | ||
196 | + | 'application/x-lzip' | ||
197 | + | 'application/x-msi' | ||
198 | + | 'application/x-mie' | ||
199 | + | 'application/x-apache-arrow' | ||
200 | + | 'application/mxf' | ||
201 | + | 'video/mp2t' | ||
202 | + | 'application/x-blender' | ||
203 | + | 'image/bpg' | ||
204 | + | 'image/jp2' | ||
205 | + | 'image/jpx' | ||
206 | + | 'image/jpm' | ||
207 | + | 'image/mj2' | ||
208 | + | 'audio/aiff' | ||
209 | + | 'application/xml' | ||
210 | + | 'application/x-mobipocket-ebook' | ||
211 | + | 'image/heif' | ||
212 | + | 'image/heif-sequence' | ||
213 | + | 'image/heic' | ||
214 | + | 'image/heic-sequence' | ||
215 | + | 'image/ktx' | ||
216 | + | 'application/dicom' | ||
217 | + | 'audio/x-musepack' | ||
218 | + | 'text/calendar' | ||
219 | + | 'model/gltf-binary' | ||
220 | + | 'application/vnd.tcpdump.pcap' | ||
221 | + | 'audio/x-dsf' // Non-standard | ||
222 | + | 'application/x.ms.shortcut' // Invented by us | ||
223 | + | 'application/x.apple.alias' // Invented by us | ||
224 | + | 'audio/x-voc' | ||
225 | + | 'audio/vnd.dolby.dd-raw' | ||
226 | + | 'audio/x-m4a' | ||
227 | + | 'image/apng' | ||
228 | + | 'image/x-olympus-orf' | ||
229 | + | 'image/x-sony-arw' | ||
230 | + | 'image/x-adobe-dng' | ||
231 | + | 'image/x-nikon-nef' | ||
232 | + | 'image/x-panasonic-rw2' | ||
233 | + | 'image/x-fujifilm-raf' | ||
234 | + | 'video/x-m4v' | ||
235 | + | 'video/3gpp2' | ||
236 | + | 'application/x-esri-shape'; | ||
237 | + | ||
238 | + interface FileTypeResult { | ||
239 | + /** | ||
240 | + One of the supported [file types](https://github.com/sindresorhus/file-type#supported-file-types). | ||
241 | + */ | ||
242 | + ext: FileType; | ||
243 | + | ||
244 | + /** | ||
245 | + The detected [MIME type](https://en.wikipedia.org/wiki/Internet_media_type). | ||
246 | + */ | ||
247 | + mime: MimeType; | ||
248 | + } | ||
249 | + | ||
250 | + type ReadableStreamWithFileType = ReadableStream & { | ||
251 | + readonly fileType?: FileTypeResult; | ||
252 | + }; | ||
253 | +} | ||
254 | + | ||
255 | +declare const fileType: { | ||
256 | + /** | ||
257 | + Detect the file type of a `Buffer`/`Uint8Array`/`ArrayBuffer`. The file type is detected by checking the [magic number](https://en.wikipedia.org/wiki/Magic_number_(programming)#Magic_numbers_in_files) of the buffer. | ||
258 | + | ||
259 | + @param buffer - It only needs the first `.minimumBytes` bytes. The exception is detection of `docx`, `pptx`, and `xlsx` which potentially requires reading the whole file. | ||
260 | + @returns The detected file type and MIME type or `undefined` when there was no match. | ||
261 | + | ||
262 | + @example | ||
263 | + ``` | ||
264 | + import readChunk = require('read-chunk'); | ||
265 | + import fileType = require('file-type'); | ||
266 | + | ||
267 | + const buffer = readChunk.sync('unicorn.png', 0, fileType.minimumBytes); | ||
268 | + | ||
269 | + fileType(buffer); | ||
270 | + //=> {ext: 'png', mime: 'image/png'} | ||
271 | + | ||
272 | + | ||
273 | + // Or from a remote location: | ||
274 | + | ||
275 | + import * as http from 'http'; | ||
276 | + | ||
277 | + const url = 'https://assets-cdn.github.com/images/spinners/octocat-spinner-32.gif'; | ||
278 | + | ||
279 | + http.get(url, response => { | ||
280 | + response.on('readable', () => { | ||
281 | + const chunk = response.read(fileType.minimumBytes); | ||
282 | + response.destroy(); | ||
283 | + console.log(fileType(chunk)); | ||
284 | + //=> {ext: 'gif', mime: 'image/gif'} | ||
285 | + }); | ||
286 | + }); | ||
287 | + ``` | ||
288 | + */ | ||
289 | + (buffer: Buffer | Uint8Array | ArrayBuffer): fileType.FileTypeResult | undefined; | ||
290 | + | ||
291 | + /** | ||
292 | + The minimum amount of bytes needed to detect a file type. Currently, it's 4100 bytes, but it can change, so don't hard-code it. | ||
293 | + */ | ||
294 | + readonly minimumBytes: number; | ||
295 | + | ||
296 | + /** | ||
297 | + Supported file extensions. | ||
298 | + */ | ||
299 | + readonly extensions: readonly fileType.FileType[]; | ||
300 | + | ||
301 | + /** | ||
302 | + Supported MIME types. | ||
303 | + */ | ||
304 | + readonly mimeTypes: readonly fileType.MimeType[]; | ||
305 | + | ||
306 | + /** | ||
307 | + Detect the file type of a readable stream. | ||
308 | + | ||
309 | + @param readableStream - A readable stream containing a file to examine, see: [`stream.Readable`](https://nodejs.org/api/stream.html#stream_class_stream_readable). | ||
310 | + @returns A `Promise` which resolves to the original readable stream argument, but with an added `fileType` property, which is an object like the one returned from `fileType()`. | ||
311 | + | ||
312 | + @example | ||
313 | + ``` | ||
314 | + import * as fs from 'fs'; | ||
315 | + import * as crypto from 'crypto'; | ||
316 | + import fileType = require('file-type'); | ||
317 | + | ||
318 | + (async () => { | ||
319 | + const read = fs.createReadStream('encrypted.enc'); | ||
320 | + const decipher = crypto.createDecipheriv(alg, key, iv); | ||
321 | + | ||
322 | + const stream = await fileType.stream(read.pipe(decipher)); | ||
323 | + | ||
324 | + console.log(stream.fileType); | ||
325 | + //=> {ext: 'mov', mime: 'video/quicktime'} | ||
326 | + | ||
327 | + const write = fs.createWriteStream(`decrypted.${stream.fileType.ext}`); | ||
328 | + stream.pipe(write); | ||
329 | + })(); | ||
330 | + ``` | ||
331 | + */ | ||
332 | + readonly stream: ( | ||
333 | + readableStream: ReadableStream | ||
334 | + ) => Promise<fileType.ReadableStreamWithFileType>; | ||
335 | +}; | ||
336 | + | ||
337 | +export = fileType; |
node_modules/file-type/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/file-type/license
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: | ||
6 | + | ||
7 | +The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. | ||
8 | + | ||
9 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
node_modules/file-type/package.json
0 → 100644
1 | +{ | ||
2 | + "name": "file-type", | ||
3 | + "version": "12.4.2", | ||
4 | + "description": "Detect the file type of a Buffer/Uint8Array/ArrayBuffer", | ||
5 | + "license": "MIT", | ||
6 | + "repository": "sindresorhus/file-type", | ||
7 | + "author": { | ||
8 | + "name": "Sindre Sorhus", | ||
9 | + "email": "sindresorhus@gmail.com", | ||
10 | + "url": "sindresorhus.com" | ||
11 | + }, | ||
12 | + "engines": { | ||
13 | + "node": ">=8" | ||
14 | + }, | ||
15 | + "scripts": { | ||
16 | + "test": "xo && ava && tsd" | ||
17 | + }, | ||
18 | + "files": [ | ||
19 | + "index.js", | ||
20 | + "index.d.ts", | ||
21 | + "supported.js", | ||
22 | + "util.js" | ||
23 | + ], | ||
24 | + "keywords": [ | ||
25 | + "mime", | ||
26 | + "file", | ||
27 | + "type", | ||
28 | + "archive", | ||
29 | + "image", | ||
30 | + "img", | ||
31 | + "pic", | ||
32 | + "picture", | ||
33 | + "flash", | ||
34 | + "photo", | ||
35 | + "video", | ||
36 | + "detect", | ||
37 | + "check", | ||
38 | + "is", | ||
39 | + "exif", | ||
40 | + "exe", | ||
41 | + "binary", | ||
42 | + "buffer", | ||
43 | + "uint8array", | ||
44 | + "jpg", | ||
45 | + "png", | ||
46 | + "apng", | ||
47 | + "gif", | ||
48 | + "webp", | ||
49 | + "flif", | ||
50 | + "cr2", | ||
51 | + "orf", | ||
52 | + "arw", | ||
53 | + "dng", | ||
54 | + "nef", | ||
55 | + "rw2", | ||
56 | + "raf", | ||
57 | + "tif", | ||
58 | + "bmp", | ||
59 | + "jxr", | ||
60 | + "psd", | ||
61 | + "zip", | ||
62 | + "tar", | ||
63 | + "rar", | ||
64 | + "gz", | ||
65 | + "bz2", | ||
66 | + "7z", | ||
67 | + "dmg", | ||
68 | + "mp4", | ||
69 | + "mid", | ||
70 | + "mkv", | ||
71 | + "webm", | ||
72 | + "mov", | ||
73 | + "avi", | ||
74 | + "mpg", | ||
75 | + "mp2", | ||
76 | + "mp3", | ||
77 | + "m4a", | ||
78 | + "ogg", | ||
79 | + "opus", | ||
80 | + "flac", | ||
81 | + "wav", | ||
82 | + "amr", | ||
83 | + "pdf", | ||
84 | + "epub", | ||
85 | + "mobi", | ||
86 | + "swf", | ||
87 | + "rtf", | ||
88 | + "woff", | ||
89 | + "woff2", | ||
90 | + "eot", | ||
91 | + "ttf", | ||
92 | + "otf", | ||
93 | + "ico", | ||
94 | + "flv", | ||
95 | + "ps", | ||
96 | + "xz", | ||
97 | + "sqlite", | ||
98 | + "xpi", | ||
99 | + "cab", | ||
100 | + "deb", | ||
101 | + "ar", | ||
102 | + "rpm", | ||
103 | + "Z", | ||
104 | + "lz", | ||
105 | + "msi", | ||
106 | + "mxf", | ||
107 | + "mts", | ||
108 | + "wasm", | ||
109 | + "webassembly", | ||
110 | + "blend", | ||
111 | + "bpg", | ||
112 | + "docx", | ||
113 | + "pptx", | ||
114 | + "xlsx", | ||
115 | + "3gp", | ||
116 | + "jp2", | ||
117 | + "jpm", | ||
118 | + "jpx", | ||
119 | + "mj2", | ||
120 | + "aif", | ||
121 | + "odt", | ||
122 | + "ods", | ||
123 | + "odp", | ||
124 | + "xml", | ||
125 | + "heic", | ||
126 | + "wma", | ||
127 | + "ics", | ||
128 | + "glb", | ||
129 | + "pcap", | ||
130 | + "dsf", | ||
131 | + "lnk", | ||
132 | + "alias", | ||
133 | + "voc", | ||
134 | + "ac3", | ||
135 | + "3g2", | ||
136 | + "m4b", | ||
137 | + "m4p", | ||
138 | + "m4v", | ||
139 | + "f4a", | ||
140 | + "f4b", | ||
141 | + "f4p", | ||
142 | + "f4v", | ||
143 | + "mie", | ||
144 | + "qcp", | ||
145 | + "wmv", | ||
146 | + "asf", | ||
147 | + "ogv", | ||
148 | + "ogm", | ||
149 | + "oga", | ||
150 | + "spx", | ||
151 | + "ogx", | ||
152 | + "ape", | ||
153 | + "wv", | ||
154 | + "cur", | ||
155 | + "nes", | ||
156 | + "crx", | ||
157 | + "ktx", | ||
158 | + "dcm", | ||
159 | + "mpc", | ||
160 | + "arrow", | ||
161 | + "shp" | ||
162 | + ], | ||
163 | + "devDependencies": { | ||
164 | + "@types/node": "^12.7.2", | ||
165 | + "ava": "^2.3.0", | ||
166 | + "noop-stream": "^0.1.0", | ||
167 | + "pify": "^4.0.1", | ||
168 | + "read-chunk": "^3.2.0", | ||
169 | + "tsd": "^0.7.1", | ||
170 | + "xo": "^0.24.0" | ||
171 | + } | ||
172 | +} |
node_modules/file-type/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/file-type/supported.js
0 → 100644
1 | +'use strict'; | ||
2 | + | ||
3 | +module.exports = { | ||
4 | + extensions: [ | ||
5 | + 'jpg', | ||
6 | + 'png', | ||
7 | + 'apng', | ||
8 | + 'gif', | ||
9 | + 'webp', | ||
10 | + 'flif', | ||
11 | + 'cr2', | ||
12 | + 'orf', | ||
13 | + 'arw', | ||
14 | + 'dng', | ||
15 | + 'nef', | ||
16 | + 'rw2', | ||
17 | + 'raf', | ||
18 | + 'tif', | ||
19 | + 'bmp', | ||
20 | + 'jxr', | ||
21 | + 'psd', | ||
22 | + 'zip', | ||
23 | + 'tar', | ||
24 | + 'rar', | ||
25 | + 'gz', | ||
26 | + 'bz2', | ||
27 | + '7z', | ||
28 | + 'dmg', | ||
29 | + 'mp4', | ||
30 | + 'mid', | ||
31 | + 'mkv', | ||
32 | + 'webm', | ||
33 | + 'mov', | ||
34 | + 'avi', | ||
35 | + 'mpg', | ||
36 | + 'mp2', | ||
37 | + 'mp3', | ||
38 | + 'm4a', | ||
39 | + 'oga', | ||
40 | + 'ogg', | ||
41 | + 'ogv', | ||
42 | + 'opus', | ||
43 | + 'flac', | ||
44 | + 'wav', | ||
45 | + 'spx', | ||
46 | + 'amr', | ||
47 | + 'pdf', | ||
48 | + 'epub', | ||
49 | + 'exe', | ||
50 | + 'swf', | ||
51 | + 'rtf', | ||
52 | + 'wasm', | ||
53 | + 'woff', | ||
54 | + 'woff2', | ||
55 | + 'eot', | ||
56 | + 'ttf', | ||
57 | + 'otf', | ||
58 | + 'ico', | ||
59 | + 'flv', | ||
60 | + 'ps', | ||
61 | + 'xz', | ||
62 | + 'sqlite', | ||
63 | + 'nes', | ||
64 | + 'crx', | ||
65 | + 'xpi', | ||
66 | + 'cab', | ||
67 | + 'deb', | ||
68 | + 'ar', | ||
69 | + 'rpm', | ||
70 | + 'Z', | ||
71 | + 'lz', | ||
72 | + 'msi', | ||
73 | + 'mxf', | ||
74 | + 'mts', | ||
75 | + 'blend', | ||
76 | + 'bpg', | ||
77 | + 'docx', | ||
78 | + 'pptx', | ||
79 | + 'xlsx', | ||
80 | + '3gp', | ||
81 | + '3g2', | ||
82 | + 'jp2', | ||
83 | + 'jpm', | ||
84 | + 'jpx', | ||
85 | + 'mj2', | ||
86 | + 'aif', | ||
87 | + 'qcp', | ||
88 | + 'odt', | ||
89 | + 'ods', | ||
90 | + 'odp', | ||
91 | + 'xml', | ||
92 | + 'mobi', | ||
93 | + 'heic', | ||
94 | + 'cur', | ||
95 | + 'ktx', | ||
96 | + 'ape', | ||
97 | + 'wv', | ||
98 | + 'wmv', | ||
99 | + 'wma', | ||
100 | + 'dcm', | ||
101 | + 'ics', | ||
102 | + 'glb', | ||
103 | + 'pcap', | ||
104 | + 'dsf', | ||
105 | + 'lnk', | ||
106 | + 'alias', | ||
107 | + 'voc', | ||
108 | + 'ac3', | ||
109 | + 'm4v', | ||
110 | + 'm4p', | ||
111 | + 'm4b', | ||
112 | + 'f4v', | ||
113 | + 'f4p', | ||
114 | + 'f4b', | ||
115 | + 'f4a', | ||
116 | + 'mie', | ||
117 | + 'asf', | ||
118 | + 'ogm', | ||
119 | + 'ogx', | ||
120 | + 'mpc', | ||
121 | + 'arrow', | ||
122 | + 'shp' | ||
123 | + ], | ||
124 | + mimeTypes: [ | ||
125 | + 'image/jpeg', | ||
126 | + 'image/png', | ||
127 | + 'image/gif', | ||
128 | + 'image/webp', | ||
129 | + 'image/flif', | ||
130 | + 'image/x-canon-cr2', | ||
131 | + 'image/tiff', | ||
132 | + 'image/bmp', | ||
133 | + 'image/vnd.ms-photo', | ||
134 | + 'image/vnd.adobe.photoshop', | ||
135 | + 'application/epub+zip', | ||
136 | + 'application/x-xpinstall', | ||
137 | + 'application/vnd.oasis.opendocument.text', | ||
138 | + 'application/vnd.oasis.opendocument.spreadsheet', | ||
139 | + 'application/vnd.oasis.opendocument.presentation', | ||
140 | + 'application/vnd.openxmlformats-officedocument.wordprocessingml.document', | ||
141 | + 'application/vnd.openxmlformats-officedocument.presentationml.presentation', | ||
142 | + 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet', | ||
143 | + 'application/zip', | ||
144 | + 'application/x-tar', | ||
145 | + 'application/x-rar-compressed', | ||
146 | + 'application/gzip', | ||
147 | + 'application/x-bzip2', | ||
148 | + 'application/x-7z-compressed', | ||
149 | + 'application/x-apple-diskimage', | ||
150 | + 'application/x-apache-arrow', | ||
151 | + 'video/mp4', | ||
152 | + 'audio/midi', | ||
153 | + 'video/x-matroska', | ||
154 | + 'video/webm', | ||
155 | + 'video/quicktime', | ||
156 | + 'video/vnd.avi', | ||
157 | + 'audio/vnd.wave', | ||
158 | + 'audio/qcelp', | ||
159 | + 'audio/x-ms-wma', | ||
160 | + 'video/x-ms-asf', | ||
161 | + 'application/vnd.ms-asf', | ||
162 | + 'video/mpeg', | ||
163 | + 'video/3gpp', | ||
164 | + 'audio/mpeg', | ||
165 | + 'audio/mp4', // RFC 4337 | ||
166 | + 'audio/opus', | ||
167 | + 'video/ogg', | ||
168 | + 'audio/ogg', | ||
169 | + 'application/ogg', | ||
170 | + 'audio/x-flac', | ||
171 | + 'audio/ape', | ||
172 | + 'audio/wavpack', | ||
173 | + 'audio/amr', | ||
174 | + 'application/pdf', | ||
175 | + 'application/x-msdownload', | ||
176 | + 'application/x-shockwave-flash', | ||
177 | + 'application/rtf', | ||
178 | + 'application/wasm', | ||
179 | + 'font/woff', | ||
180 | + 'font/woff2', | ||
181 | + 'application/vnd.ms-fontobject', | ||
182 | + 'font/ttf', | ||
183 | + 'font/otf', | ||
184 | + 'image/x-icon', | ||
185 | + 'video/x-flv', | ||
186 | + 'application/postscript', | ||
187 | + 'application/x-xz', | ||
188 | + 'application/x-sqlite3', | ||
189 | + 'application/x-nintendo-nes-rom', | ||
190 | + 'application/x-google-chrome-extension', | ||
191 | + 'application/vnd.ms-cab-compressed', | ||
192 | + 'application/x-deb', | ||
193 | + 'application/x-unix-archive', | ||
194 | + 'application/x-rpm', | ||
195 | + 'application/x-compress', | ||
196 | + 'application/x-lzip', | ||
197 | + 'application/x-msi', | ||
198 | + 'application/x-mie', | ||
199 | + 'application/mxf', | ||
200 | + 'video/mp2t', | ||
201 | + 'application/x-blender', | ||
202 | + 'image/bpg', | ||
203 | + 'image/jp2', | ||
204 | + 'image/jpx', | ||
205 | + 'image/jpm', | ||
206 | + 'image/mj2', | ||
207 | + 'audio/aiff', | ||
208 | + 'application/xml', | ||
209 | + 'application/x-mobipocket-ebook', | ||
210 | + 'image/heif', | ||
211 | + 'image/heif-sequence', | ||
212 | + 'image/heic', | ||
213 | + 'image/heic-sequence', | ||
214 | + 'image/ktx', | ||
215 | + 'application/dicom', | ||
216 | + 'audio/x-musepack', | ||
217 | + 'text/calendar', | ||
218 | + 'model/gltf-binary', | ||
219 | + 'application/vnd.tcpdump.pcap', | ||
220 | + 'audio/x-dsf', // Non-standard | ||
221 | + 'application/x.ms.shortcut', // Invented by us | ||
222 | + 'application/x.apple.alias', // Invented by us | ||
223 | + 'audio/x-voc', | ||
224 | + 'audio/vnd.dolby.dd-raw', | ||
225 | + 'audio/x-m4a', | ||
226 | + 'image/apng', | ||
227 | + 'image/x-olympus-orf', | ||
228 | + 'image/x-sony-arw', | ||
229 | + 'image/x-adobe-dng', | ||
230 | + 'image/x-nikon-nef', | ||
231 | + 'image/x-panasonic-rw2', | ||
232 | + 'image/x-fujifilm-raf', | ||
233 | + 'video/x-m4v', | ||
234 | + 'video/3gpp2', | ||
235 | + 'application/x-esri-shape' | ||
236 | + ] | ||
237 | +}; |
node_modules/file-type/util.js
0 → 100644
1 | +'use strict'; | ||
2 | + | ||
3 | +exports.stringToBytes = string => [...string].map(character => character.charCodeAt(0)); | ||
4 | + | ||
5 | +const uint8ArrayUtf8ByteString = (array, start, end) => { | ||
6 | + return String.fromCharCode(...array.slice(start, end)); | ||
7 | +}; | ||
8 | + | ||
9 | +exports.readUInt64LE = (buffer, offset = 0) => { | ||
10 | + let n = buffer[offset]; | ||
11 | + let mul = 1; | ||
12 | + let i = 0; | ||
13 | + | ||
14 | + while (++i < 8) { | ||
15 | + mul *= 0x100; | ||
16 | + n += buffer[offset + i] * mul; | ||
17 | + } | ||
18 | + | ||
19 | + return n; | ||
20 | +}; | ||
21 | + | ||
22 | +exports.tarHeaderChecksumMatches = buffer => { // Does not check if checksum field characters are valid | ||
23 | + if (buffer.length < 512) { // `tar` header size, cannot compute checksum without it | ||
24 | + return false; | ||
25 | + } | ||
26 | + | ||
27 | + const MASK_8TH_BIT = 0x80; | ||
28 | + | ||
29 | + let sum = 256; // Intitalize sum, with 256 as sum of 8 spaces in checksum field | ||
30 | + let signedBitSum = 0; // Initialize signed bit sum | ||
31 | + | ||
32 | + for (let i = 0; i < 148; i++) { | ||
33 | + const byte = buffer[i]; | ||
34 | + sum += byte; | ||
35 | + signedBitSum += byte & MASK_8TH_BIT; // Add signed bit to signed bit sum | ||
36 | + } | ||
37 | + | ||
38 | + // Skip checksum field | ||
39 | + | ||
40 | + for (let i = 156; i < 512; i++) { | ||
41 | + const byte = buffer[i]; | ||
42 | + sum += byte; | ||
43 | + signedBitSum += byte & MASK_8TH_BIT; // Add signed bit to signed bit sum | ||
44 | + } | ||
45 | + | ||
46 | + const readSum = parseInt(uint8ArrayUtf8ByteString(buffer, 148, 154), 8); // Read sum in header | ||
47 | + | ||
48 | + // Some implementations compute checksum incorrectly using signed bytes | ||
49 | + return ( | ||
50 | + // Checksum in header equals the sum we calculated | ||
51 | + readSum === sum || | ||
52 | + | ||
53 | + // Checksum in header equals sum we calculated plus signed-to-unsigned delta | ||
54 | + readSum === (sum - (signedBitSum << 1)) | ||
55 | + ); | ||
56 | +}; | ||
57 | + | ||
58 | +exports.multiByteIndexOf = (buffer, bytesToSearch, startAt = 0) => { | ||
59 | + // `Buffer#indexOf()` can search for multiple bytes | ||
60 | + if (Buffer && Buffer.isBuffer(buffer)) { | ||
61 | + return buffer.indexOf(Buffer.from(bytesToSearch), startAt); | ||
62 | + } | ||
63 | + | ||
64 | + const nextBytesMatch = (buffer, bytes, startIndex) => { | ||
65 | + for (let i = 1; i < bytes.length; i++) { | ||
66 | + if (bytes[i] !== buffer[startIndex + i]) { | ||
67 | + return false; | ||
68 | + } | ||
69 | + } | ||
70 | + | ||
71 | + return true; | ||
72 | + }; | ||
73 | + | ||
74 | + // `Uint8Array#indexOf()` can search for only a single byte | ||
75 | + let index = buffer.indexOf(bytesToSearch[0], startAt); | ||
76 | + while (index >= 0) { | ||
77 | + if (nextBytesMatch(buffer, bytesToSearch, index)) { | ||
78 | + return index; | ||
79 | + } | ||
80 | + | ||
81 | + index = buffer.indexOf(bytesToSearch[0], index + 1); | ||
82 | + } | ||
83 | + | ||
84 | + return -1; | ||
85 | +}; | ||
86 | + | ||
87 | +exports.uint8ArrayUtf8ByteString = uint8ArrayUtf8ByteString; |
node_modules/fs.realpath/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/fs.realpath/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/fs.realpath/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/fs.realpath/old.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/fs.realpath/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/glob/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/glob/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/glob/common.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/glob/glob.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/glob/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/glob/sync.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/.babelrc
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/LICENCE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/README.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/dist/idb-keyval.d.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/dist/idb-keyval.mjs
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/idb-keyval.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/missing-types.d.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/rollup.config.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/idb-keyval/tsconfig.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inflight/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inflight/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inflight/inflight.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inflight/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inherits/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inherits/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inherits/inherits.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inherits/inherits_browser.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/inherits/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-electron/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-electron/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-electron/index.d.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-electron/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-electron/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/.travis.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/History.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/LICENSE-MIT
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/Readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/is-url/test/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-autorotate/license.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-autorotate/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-autorotate/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-autorotate/src/cli.js
0 → 100755
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/jpeg-autorotate/src/main.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/.travis.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/CONTRIBUTING.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/index.d.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/lib/decoder.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/lib/encoder.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jpeg-js/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/minimatch/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/minimatch/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/minimatch/minimatch.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/minimatch/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/once/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/once/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/once/once.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/once/package.json
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/path-is-absolute/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/path-is-absolute/license
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/path-is-absolute/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/path-is-absolute/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/.gitattributes
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/LICENSE.txt
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/README.rst
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/azure-pipelines.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/bower.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/piexifjs/piexif.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/regenerator-runtime/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/regenerator-runtime/README.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/regenerator-runtime/path.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/regenerator-runtime/runtime.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/.jshintrc
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/bower.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/changelog.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/component.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/resolve-url.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/resolve-url/test/resolve-url.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js-core/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js-core/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js-core/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js-core/package.json
0 → 100644
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
This diff could not be displayed because it is too large.
No preview for this file type
This diff could not be displayed because it is too large.
node_modules/tesseract.js/.eslintrc
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/.gitpod.Dockerfile
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/.gitpod.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/LICENSE.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/README.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
node_modules/tesseract.js/dist/worker.min.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
node_modules/tesseract.js/docs/api.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/docs/examples.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/docs/faq.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.

97 KB

105 KB

237 KB
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/scripts/.eslintrc
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/scripts/server.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/src/Tesseract.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/src/createJob.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/src/index.d.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/src/index.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/src/utils/getId.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/tesseract.js/src/utils/log.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/wrappy/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/wrappy/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/wrappy/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/wrappy/wrappy.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/CHANGELOG.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/LICENSE.txt
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/browser.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/build/index.cjs
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/build/lib/index.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/yargs-parser/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/ChangeLog.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/README.en.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/.gitkeep
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/crc32.dev.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/crc32.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/deflate.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/deflate.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/gunzip.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/gunzip.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/gzip.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/gzip.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/inflate.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/inflate.min.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/node-zlib.dev.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/node-zlib.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/rawdeflate.min.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/rawinflate.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/unzip.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/unzip.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/zip.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/zip.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/zlib.dev.min.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/zlib.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/bin/zlib.pretty.dev.js
0 → 100644
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/zlib.pretty.js
0 → 100644
This diff could not be displayed because it is too large.
This diff could not be displayed because it is too large.
node_modules/zlibjs/bin/zlib_and_gzip.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/zlibjs/package.json
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
-
Please register or login to post a comment